POJ066 Treasure Hunt(计算几何)
来源:互联网 发布:网络订餐食品安全 编辑:程序博客网 时间:2024/05/24 00:10
Treasure Hunt
Time Limit: 1000MS Memory Limit: 10000KTotal Submissions: 7387 Accepted: 3046
Description
Archeologists from the Antiquities and Curios Museum (ACM) have flown to Egypt to examine the great pyramid of Key-Ops. Using state-of-the-art technology they are able to determine that the lower floor of the pyramid is constructed from a series of straightline walls, which intersect to form numerous enclosed chambers. Currently, no doors exist to allow access to any chamber. This state-of-the-art technology has also pinpointed the location of the treasure room. What these dedicated (and greedy) archeologists want to do is blast doors through the walls to get to the treasure room. However, to minimize the damage to the artwork in the intervening chambers (and stay under their government grant for dynamite) they want to blast through the minimum number of doors. For structural integrity purposes, doors should only be blasted at the midpoint of the wall of the room being entered. You are to write a program which determines this minimum number of doors.
An example is shown below:
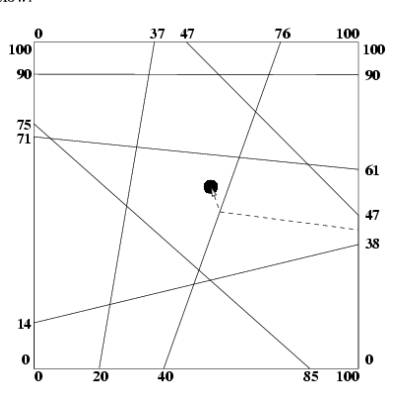
An example is shown below:
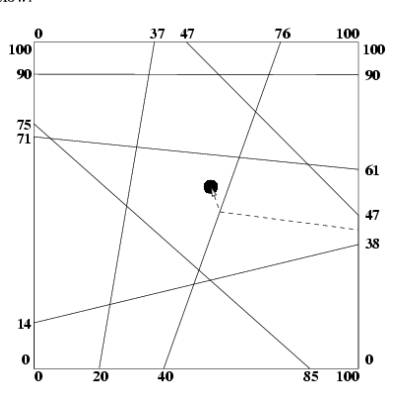
Input
The input will consist of one case. The first line will be an integer n (0 <= n <= 30) specifying number of interior walls, followed by n lines containing integer endpoints of each wall x1 y1 x2 y2 . The 4 enclosing walls of the pyramid have fixed endpoints at (0,0); (0,100); (100,100) and (100,0) and are not included in the list of walls. The interior walls always span from one exterior wall to another exterior wall and are arranged such that no more than two walls intersect at any point. You may assume that no two given walls coincide. After the listing of the interior walls there will be one final line containing the floating point coordinates of the treasure in the treasure room (guaranteed not to lie on a wall).
Output
Print a single line listing the minimum number of doors which need to be created, in the format shown below.
Sample Input
7 20 0 37 100 40 0 76 100 85 0 0 75 100 90 0 90 0 71 100 61 0 14 100 38 100 47 47 100 54.5 55.4
Sample Output
Number of doors = 2
题意:一个矩形里有一个点,有很多线段,问从那个点到矩形外最少经过多少线段,每次只能从线段中间过.
算法:枚举线段端点和目标点形成的线段,计算交点个数.
#include<cstdio>#include<cstdlib>#include<cstring>#include<cmath>#include<vector>#include<algorithm>#include <iostream>#include <string>#include <set>#include <map>#include <queue>using namespace std;const int MAXN = 5000+10;const int INF=1e9+7;const double eps=1e-8;const double pi=acos(-1.0);//计算几何误差修正//输入为一个double类型的数,返回-1表示负数,1表示正数,0表示x为0int cmp(double x){ if(fabs(x)<eps) return 0; if(x>0) return 1; return -1;}//计算几何点类inline double sqr(double x){ return x*x;}struct point{ double x,y; point(){} point(double a,double b):x(a),y(b){} void input(){ scanf("%lf%lf",&x,&y); } //加法 friend point operator + (const point &a,const point &b){ return point(a.x+b.x,a.y+b.y); } //减法 friend point operator - (const point &a,const point &b){ return point(a.x-b.x,a.y-b.y); } //判断相等 friend bool operator == (const point &a,const point &b){ return cmp(a.x-b.x)==0&&cmp(a.y-b.y)==0; } //倍增 friend point operator * (const point &a,const double &b){ return point(a.x*b,a.y*b); } //倍增 friend point operator * (const double &b,const point &a){ return point(a.x*b,a.y*b); } //除法 friend point operator / (const point &a,const double b){ return point(a.x/b,a.y/b); } //模长 double norm(){ return sqrt(sqr(x)+sqr(y)); }};//叉积,a×b>0代表a在b的顺时针方向,<0代表a在b的逆时针方向,等于0代表a和b向量共线,但不确定方向是否相同double det(const point &a,const point &b){ return a.x*b.y-a.y*b.x;}//点积double dot(const point &a,const point &b){ return a.x*b.x+a.y*b.y;}//距离double dist(const point &a,const point &b){ return (a-b).norm();}//op向量绕原点逆时针旋转A(弧度)point rotate_point(const point &p,double A){ double tx=p.x,ty=p.y; return point(tx*cos(A)-ty*sin(A),tx*sin(A)+ty*cos(A));}//计算几何线段类struct line{ point a,b; line(){} line(point x,point y):a(x),b(y){} void input(){ a.input(); b.input(); }};//用两个点a,b生成的一个线段或者直线line point_make_line(point a,point b){ return line(a,b);}//求点p到线段st的距离double dis_point_segment(point p,point s,point t){ if(cmp(dot(p-s,t-s))<0) return (p-s).norm(); if(cmp(dot(p-s,s-t))<0) return (p-t).norm(); return fabs(det(s-p,t-p)/dist(s,t));}//求点p到线段st的垂足,保存在cp中void PointProjLine(point p,point s,point t,point &cp){ double r=dot((t-s),(p-s))/dot(t-s,t-s); cp=s+(t-s)*r;}//判断p点是否在线段st上bool PointOnSegment(point p,point s,point t){ return cmp(det(p-s,t-s))==0&&cmp(dot(p-s,p-t))<=0;}//判断a和b是否平行bool parallel(line a,line b){ return !cmp(det(a.a-a.b,b.a-b.b));}//判断a和b是否共线bool contribution(line a,line b){ if(!parallel(a, b)) return false; if(!cmp(det(a.b-a.a,b.a-a.b))) return true; return false;}//判断a和b是否相交,若相交则返回true且交点保存在res中bool line_make_point(line a,line b,point &res){ if(parallel(a, b)) return false; double s1=det(a.a-b.a,b.b-b.a); double s2=det(a.b-b.a,b.b-b.a); res=(s1*a.b-s2*a.a)/(s1-s2); return true;}//判断线段是否相交bool segment_make_point(line a,line b,point &res){ if(!line_make_point(a,b,res)&&!PointOnSegment(a.a, b.a, b.b)&&!PointOnSegment(a.b, b.a, b.b)) return false; if(PointOnSegment(res, a.a, a.b)&&PointOnSegment(res, b.a, b.b)) return true; return false;}//判断线段和直线是否相交,a是直线,b是线段bool line_across_segment(line a,line b){ if(cmp(det(a.b-a.a,b.a-a.a)*det(a.b-a.a,b.b-a.a))==1){ return false; } return true;}//将直线a沿法向量方向平移距离len得到的直线line move_d(line a,const double &len){ point d=a.b-a.a; d=d/d.norm(); d=rotate_point(d, pi/2); return line(a.a+d*len,a.b+d*len);}point p[MAXN];line l[MAXN];int main(){ int n; scanf("%d",&n); int s1=0; for(int i=0;i<n;i++){ l[i].input(); p[s1++]=l[i].a; p[s1++]=l[i].b; } point p0; p0.input(); int res=INF; for(int i=0;i<s1;i++){ line L=point_make_line(p[i],p0); int cnt=0; for(int j=0;j<n;j++){ point ans; if(segment_make_point(L, l[j], ans)) cnt++; } res=min(res,cnt); } if(!n) res=1; printf("Number of doors = %d\n",res);}
阅读全文
0 0
- POJ066 Treasure Hunt(计算几何)
- 【计算几何】poj1066 Treasure Hunt
- pku 1066 Treasure Hunt(计算几何)
- poj 1066 Treasure Hunt 计算几何计数
- POJ 1066 Treasure Hunt(计算几何)
- POJ 1066Treasure Hunt 计算几何
- POJ 1066 Treasure Hunt [线段相交]【计算几何】
- POJ1066---Treasure Hunt (计算几何基础:线段相交)
- POJ-1066Treasure Hunt(计算几何线段相交)
- UVa 754 Treasure Hunt <计算几何(相交判断)>
- POJ 1066 Treasure Hunt 计算几何 枚举线段与直线相交
- 2015年ALPC暑期专题练习I (计算几何) G - Treasure Hunt
- POJ 1066 Treasure Hunt --几何,线段相交
- uva 754 - Treasure Hunt(几何)
- POJ 2606 Rabbit hunt(计算几何)
- Google Treasure Hunt 2008
- pku1066 Treasure Hunt
- poj 1066 Treasure Hunt
- 为抵抗洪水,战士连续作战89小时,编程计算共多少天零多少小时?
- hadoop-HA启动,以及配了HA之后hdfs的路径问题
- C# 网络编程之基于SMTP发送电子邮件
- 使用 Spring Boot websocket 写简单网页聊天室
- 03 Spring框架 bean的属性以及bean前处理和bean后处理
- POJ066 Treasure Hunt(计算几何)
- C++中虚析构函数的作用
- const关键字的作用
- 在安卓中使用正则表达式2
- 第一篇博客
- 单片机 利用C语言产生正弦波DA数据
- linux部署JDK+tomcat+mariaDb
- 怎么使用CSDN-markdown编辑器写博客?
- 2017-8-31