pyQt5-Hello World
来源:互联网 发布:帝国cms图片集 编辑:程序博客网 时间:2024/06/06 10:46
参考:
1、https://maicss.gitbooks.io/pyqt5/content/
2、https://github.com/maicss/PyQt5-Chinese-tutoral
3、https://doc.qt.io/qt-5/classes.html API
PyQt4和PyQt5的区别
PyQt5不兼容PyQt4。PyQt5有一些巨大的改进。但是,迁移并不是很难,两者的区别如下:
- 重新组合模块,一些模块已经被废弃(QtScript),有些被分为两个子模块(QtGui, QtWebKit)。
- 添加了新的模块,比如QtBluetooth, QtPositioning,和Enginio。
- 废弃了SINGAL()和SLOT()的调用方式,使用了新的信号和xx处理方式。
- 不再支持所有被标记为废弃的或不建议使用的Qt API。
Hello World
本章学习Qt的基本功能
例1,简单的窗口
#!/usr/bin/python3# -*- coding: utf-8 -*-"""ZetCode PyQt5 tutorial In this example, we create a simplewindow in PyQt5.author: Jan Bodnarwebsite: zetcode.com Last edited: August 2017"""import sysfrom PyQt5.QtWidgets import QApplication, QWidgetif __name__ == '__main__': app = QApplication(sys.argv) # 创建一个应用对象 sys.argv是一组命令行参数的列表 # QWidge控件是一个用户界面的基本控件,它提供了基本的应用构造器。 # 默认情况下,构造器是没有父级的,没有父级的构造器被称为窗口(window)。 w = QWidget() # resize()方法能改变控件的大小,这里的意思是窗口宽250px,高150px。 w.resize(250, 150) # move()是修改控件位置的的方法。它把控件放置到屏幕坐标的(300, 300)的位置。 # 注:屏幕坐标系的原点是屏幕的左上角。 w.move(300, 300) # 我们给这个窗口添加了一个标题,标题在标题栏展示(虽然这看起来是一句废话, # 但是后面还有各种栏,还是要注意一下,多了就蒙了)。 w.setWindowTitle('Simple') # show()能让控件在桌面上显示出来。控件在内存里创建,之后才能在显示器上显示出来。 w.show() # 最后,我们进入了应用的主循环中,事件处理器这个时候开始工作。主循环从窗口上接收事件, # 并把事件传入到派发到应用控件里。当调用exit()方法或直接销毁主控件时,主循环就会结束。 # sys.exit()方法能确保主循环安全退出。外部环境能通知主控件怎么结束 # exec_()之所以有个下划线,是因为exec是一个Python的关键字。 sys.exit(app.exec_())
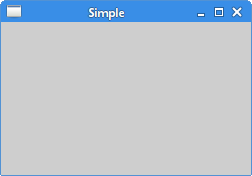
例2,带窗口图标
#!/usr/bin/python3# -*- coding: utf-8 -*-"""ZetCode PyQt5 tutorial This example shows an iconin the titlebar of the window.Author: Jan BodnarWebsite: zetcode.com Last edited: August 2017"""import sysfrom PyQt5.QtWidgets import QApplication, QWidgetfrom PyQt5.QtGui import QIconclass Example(QWidget): def __init__(self): super().__init__() # super()构造器方法返回父级的对象 self.initUI() def initUI(self): # 这三个方法都继承自QWidget类。 # 把窗口放到屏幕上并且设置窗口大小。参数分别代表屏幕坐标的x、y和窗口大小的宽、高。 # 也就是说这个方法是resize()和move()的合体。 self.setGeometry(300, 300, 300, 220) # 窗口添加了一个标题 self.setWindowTitle('Icon') # 添加了图标,先创建一个QIcon对象,然后接受一个路径作为参数显示图标 self.setWindowIcon(QIcon('1234.ico')) self.show()if __name__ == '__main__': # 创建一个应用对象 sys.argv是一组命令行参数的列表 app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_())
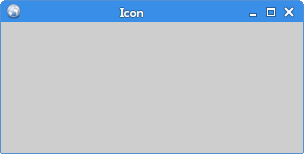
例3,提示框
#!/usr/bin/python3# -*- coding: utf-8 -*-"""ZetCode PyQt5 tutorial This example shows a tooltip on a window and a button.Author: Jan BodnarWebsite: zetcode.com Last edited: August 2017"""import sysfrom PyQt5.QtWidgets import (QWidget, QToolTip, QPushButton, QApplication)from PyQt5.QtGui import QFontclass Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): # 创建了一个提示框,设置了提示框的字体,我们使用了10px的SansSerif字体 QToolTip.setFont(QFont('SansSerif', 10)) # 创建提示框可以使用富文本格式的内容 self.setToolTip('This is a <b>QWidget</b> widget') # 创建一个按钮,并且为按钮添加了一个提示框 btn = QPushButton('Button', self) btn.setToolTip('This is a <b>QPushButton</b> widget') # 调整按钮大小,并让按钮在屏幕上显示出来,sizeHint()方法提供了一个默认的按钮大小 btn.resize(btn.sizeHint()) btn.move(50, 50) # 对主窗口设置 self.setGeometry(300, 300, 300, 200) self.setWindowTitle('Tooltips') self.show()if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_())
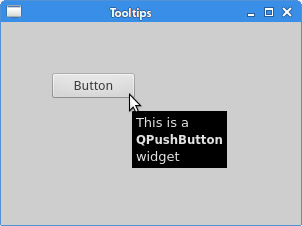
例4,关闭窗口
#!/usr/bin/python3# -*- coding: utf-8 -*-"""ZetCode PyQt5 tutorial This program creates a quitbutton. When we press the button,the application terminates. Author: Jan BodnarWebsite: zetcode.com Last edited: August 2017"""import sysfrom PyQt5.QtWidgets import QWidget, QPushButton, QApplicationfrom PyQt5.QtCore import QCoreApplicationclass Example(QWidget): def __init__(self): super().__init__() # super()构造器方法返回父级的对象 self.initUI() def initUI(self): qbtn = QPushButton('Quit', self) # 这里的self 是主窗口QWidget返回的对象 # QCoreApplication包含了事件的主循环,它能添加和删除所有的事件, # instance()创建了一个它的实例。 qbtn.clicked.connect(QCoreApplication.instance().quit) qbtn.resize(qbtn.sizeHint()) qbtn.move(50, 50) self.setGeometry(300, 300, 250, 150) self.setWindowTitle('Quit button') self.show()if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_())
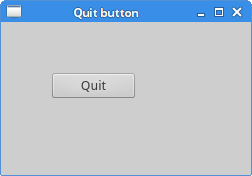
例5,消息盒子
#!/usr/bin/python3# -*- coding: utf-8 -*-"""ZetCode PyQt5 tutorial This program shows a confirmation message box when we click on the closebutton of the application window. Author: Jan BodnarWebsite: zetcode.com Last edited: August 2017"""import sysfrom PyQt5.QtWidgets import QWidget, QMessageBox, QApplicationclass Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): self.setGeometry(300, 300, 250, 150) self.setWindowTitle('Message box') self.show() # 如果关闭QWidget,就会产生一个QCloseEvent。 def closeEvent(self, event): # 创建了一个消息框,上面有俩按钮:Yes和No.第一个字符串显示在消息框的标题栏, # 第二个字符串显示在对话框,第三个参数是消息框的俩按钮,最后一个参数是默认按钮, # 这个按钮是默认选中的。返回值在变量reply里。 reply = QMessageBox.question(self, 'Message', "Are you sure to quit?", QMessageBox.Yes | QMessageBox.No, QMessageBox.No) if reply == QMessageBox.Yes: # reply == QtGui.QMessageBox.Yes event.accept() else: event.ignore()if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_())
例6,窗口居中
#!/usr/bin/python3# -*- coding: utf-8 -*-"""ZetCode PyQt5 tutorial This program centers a window on the screen. Author: Jan BodnarWebsite: zetcode.com Last edited: August 2017"""import sysfrom PyQt5.QtWidgets import QWidget, QDesktopWidget, QApplicationclass Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): self.resize(250, 150) self.center() self.setWindowTitle('Center') self.show() # QtGui.QDesktopWidget提供了用户的桌面信息,包括屏幕的大小 # 实现对话框居中的方法 def center(self): qr = self.frameGeometry() # 得到了主窗口的大小 cp = QDesktopWidget().availableGeometry().center() # 获取显示器的分辨率,然后得到中间点的位置 qr.moveCenter(cp) # 把自己窗口的中心点放置到qr的中心点 self.move(qr.topLeft()) # 把窗口的坐上角的坐标设置为qr的矩形左上角的坐标,这样就把窗口居中了if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_())
阅读全文
0 0
- pyQt5-Hello World
- python PyQt5初级教程hello world
- python PyQt5初级教程hello world
- PyQt5中文基础教程2 Hello World
- 小白学PyQt5(2):Hello World
- PyQt5的例子(一)——hello world
- PyQt5 IDE安装并执行第一个hello world程序
- Hello World!【Hello World】
- 用 eric6 与 PyQt5 实现python的极速GUI编程(系列01)--Hello world!
- Hello, world!
- Hello World!
- Hello world!
- Hello World!
- Hello World!
- hello world!
- Hello World !
- Hello,World!
- Hello World!
- javascript:void()的理解
- cmake cannot find -lopencv_dep_cudart
- wps打印文章省纸省墨技巧最大限度的节约纸张资源
- java创建二叉树以及8种遍历方法
- 2017的金秋,派卧底去阿里、京东、美团、滴滴带回来的面试题及答案
- pyQt5-Hello World
- java学习第32天,Calendar计算时间
- 锋利的jQuery学习笔记(二)—————解决jQuery和其它库的冲突
- 不能给祖国母亲添堵!技术人讲解十九大期间如何护航网站安全
- 0基础lua学习(一)HelloWorld
- learning r with swirl -basic building blocks
- 计算机基础知识(一)
- wps制作班级管理日志让班主任的管理工作事半功倍
- jQuery操作数组、Ajax