C#制作简易播放器
来源:互联网 发布:powerdesigner 转sql 编辑:程序博客网 时间:2024/05/14 06:36
.net framework里有了许多COM组件,我们可以根据应用程序的需要来使用这些组件,不用自己再去 “造轮子”了。
第一个示例是来制作一个VCD播放器.这里我使用了Windows自带的Media Play来播放多媒体文件。
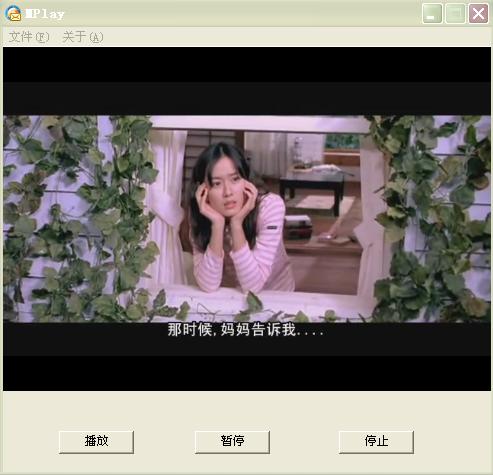
选择‘工具箱’—‘组件’,右击,选择‘添加/移除项’—‘COM组件‘(如果你是第一次使用Windows Media Play控件,在列表中是没有这个控件的,可以在系统文件夹下找到,一般是在C:/Windows/System32/msdxm.ocx,把这个控件加入到控件列表中,就可以使用了。
代码如下:
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;

namespace MPlayDemo


{
public class Form1 : System.Windows.Forms.Form

{
private AxMediaPlayer.AxMediaPlayer axMediaPlayer1;
public Form1()

{
InitializeComponent();
}


#region
private void InitializeComponent()

{
this.axMediaPlayer1 = new AxMediaPlayer.AxMediaPlayer();
//
// axMediaPlayer1
//
this.axMediaPlayer1.Dock = System.Windows.Forms.DockStyle.Top;
this.axMediaPlayer1.Location = new System.Drawing.Point(0, 0);
this.axMediaPlayer1.Name = "axMediaPlayer1";
this.axMediaPlayer1.OcxState = ((System.Windows.Forms.AxHost.State)(resources.GetObject("axMediaPlayer1.OcxState")));
this.axMediaPlayer1.Size = new System.Drawing.Size(488, 376);
this.axMediaPlayer1.TabIndex = 0;
}
#endregion

private void btnPlay_Click(object sender, System.EventArgs e)

{
if(this.axMediaPlayer1.FileName.Trim()=="")

{
MessageBox.Show(this," 请选择要播放的文件!!!!","信息提示",MessageBoxButtons.OK,MessageBoxIcon.Information);
return;
}
this.axMediaPlayer1.Play();
}

private void btnPause_Click(object sender, System.EventArgs e)

{
if(this.axMediaPlayer1.FileName.Trim()=="")

{
MessageBox.Show(this," 请选择要播放的文件!!!"," 信息提示",MessageBoxButtons.OK,MessageBoxIcon.Information);
return;
}
this.axMediaPlayer1.Pause();
}

private void btnStop_Click(object sender, System.EventArgs e)

{
if(this.axMediaPlayer1.FileName.Trim()=="")

{
MessageBox.Show(this,"请选择要播放的文件","信息提示",MessageBoxButtons.OK,MessageBoxIcon.Information);
return;
}
this.axMediaPlayer1.Stop();
}


private void menuItem3_Click(object sender, System.EventArgs e)

{
Application.Exit();
}

private void menuItem2_Click(object sender, System.EventArgs e)

{
this.openFileDialog1.ShowDialog();
string strFileName = this.openFileDialog1.FileName;
if(strFileName.Trim()=="")
return;
this.axMediaPlayer1.FileName = strFileName;

}

private void menuItem4_Click(object sender, System.EventArgs e)

{
this.axMediaPlayer1.AboutBox();
}

private void menuItem5_Click(object sender, System.EventArgs e)

{
this.axMediaPlayer1.FastForward();
}
}

第二个示例是制作一个DVD播放器:
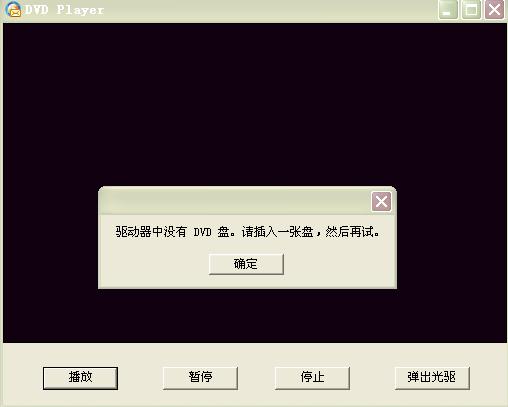
选择‘工具箱’—‘组件’,右击,选择‘添加/移除项’—‘COM组件‘把“MSWebDVD
”这个控件加入到控件列表中,就可以使用了。
代码如下:
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;

namespace DVDPlayDemo


{
public class Form1 : System.Windows.Forms.Form

{
private AxMSWEBDVDLib.AxMSWebDVD axMSWebDVD1;



Windows#region Windows
private void InitializeComponent()

{
this.axMSWebDVD1 = new AxMSWEBDVDLib.AxMSWebDVD();
//
// axMSWebDVD1
//
this.axMSWebDVD1.Dock = System.Windows.Forms.DockStyle.Top;
this.axMSWebDVD1.Enabled = true;
this.axMSWebDVD1.Location = new System.Drawing.Point(0, 0);
this.axMSWebDVD1.Name = "axMSWebDVD1";
this.axMSWebDVD1.OcxState = ((System.Windows.Forms.AxHost.State)(resources.GetObject("axMSWebDVD1.OcxState")));
this.axMSWebDVD1.Size = new System.Drawing.Size(504, 320);
this.axMSWebDVD1.TabIndex = 0;

}
#endregion


private void btnPlay_Click(object sender, System.EventArgs e)

{
try

{
this.axMSWebDVD1.Play();
}
catch(System.Exception ex)

{
MessageBox.Show(ex.Message.ToString());
}
}

private void btnPause_Click(object sender, System.EventArgs e)

{
try

{
this.axMSWebDVD1.Pause();
}
catch(System.Exception ex)

{
MessageBox.Show(ex.Message.ToString());
}
}

private void btnStop_Click(object sender, System.EventArgs e)

{
try

{
this.axMSWebDVD1.Stop();
}
catch(System.Exception ex)

{
MessageBox.Show(ex.Message.ToString());
}
}

private void btnOut_Click(object sender, System.EventArgs e)

{
try

{
this.axMSWebDVD1.Eject();
}
catch(System.Exception ex)

{
MessageBox.Show(ex.Message.ToString());
}
}


}
}

第三个示例是制作一个Flash播放器。选择‘工具箱’—‘组件’,右击,选择‘添加/移除项’—‘COM组件‘把“Shockwave Flash Object”这个控件加入到控件列表中,就可以使用了。
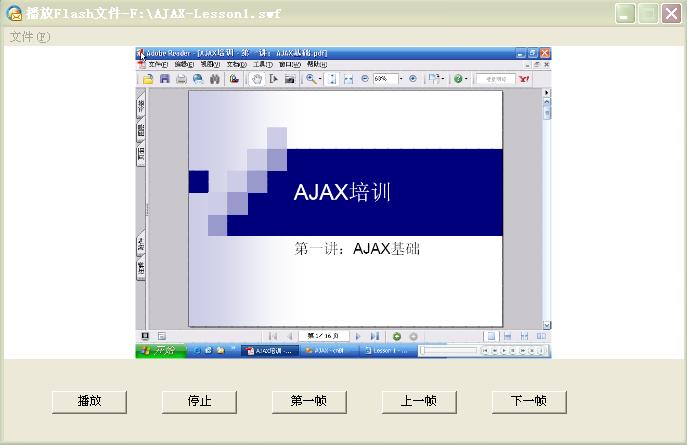
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;

namespace FlashPlayDemo


{
public class Form1 : System.Windows.Forms.Form

{
private AxShockwaveFlashObjects.AxShockwaveFlash axShockwaveFlash1;



#region
private void InitializeComponent()

{
this.axShockwaveFlash1 = new AxShockwaveFlashObjects.AxShockwaveFlash();
//
// axShockwaveFlash1
//
this.axShockwaveFlash1.Dock = System.Windows.Forms.DockStyle.Top;
this.axShockwaveFlash1.Enabled = true;
this.axShockwaveFlash1.Location = new System.Drawing.Point(0, 0);
this.axShockwaveFlash1.Name = "axShockwaveFlash1";
this.axShockwaveFlash1.OcxState = ((System.Windows.Forms.AxHost.State)(resources.GetObject("axShockwaveFlash1.OcxState")));
this.axShockwaveFlash1.Size = new System.Drawing.Size(680, 312);
this.axShockwaveFlash1.TabIndex = 0;
}
#endregion

private void menuItem2_Click(object sender, System.EventArgs e)

{
if(this.openFileDialog1.ShowDialog()==DialogResult.OK)

{
this.axShockwaveFlash1.Movie = this.openFileDialog1.FileName;
this.Text = "播放的是-"+this.openFileDialog1.FileName;
}

}

private void btnPlay_Click(object sender, System.EventArgs e)

{
if(this.openFileDialog1.FileName.Length>0)

{
this.axShockwaveFlash1.Play();
}
else

{
MessageBox.Show(this,"请选择文件!!!","信息提示",MessageBoxButtons.OK,MessageBoxIcon.Information);
}
}

private void btnStop_Click(object sender, System.EventArgs e)

{
if(this.openFileDialog1.FileName.Length>0)

{
this.axShockwaveFlash1.Stop();
}
else

{
MessageBox.Show(this,"请选择文件!!!","信息提示",MessageBoxButtons.OK,MessageBoxIcon.Information);
}
}

private void btnFisrt_Click(object sender, System.EventArgs e)

{
if(this.openFileDialog1.FileName.Length>0)

{
this.axShockwaveFlash1.Rewind();
}
else

{
MessageBox.Show(this,"请选择文件!!!","信息提示",MessageBoxButtons.OK,MessageBoxIcon.Information);
}
}

private void btnLast_Click(object sender, System.EventArgs e)

{
if(this.openFileDialog1.FileName.Length>0)

{
this.axShockwaveFlash1.Back();
}
else

{
MessageBox.Show(this,"请选择文件!!!","信息提示“,MessageBoxButtons.OK,MessageBoxIcon.Information);
}
}

private void btnNext_Click(object sender, System.EventArgs e)

{
if(this.openFileDialog1.FileName.Length>0)

{
this.axShockwaveFlash1.Forward();
}
else

{
MessageBox.Show(this,"请选择文件!!!","信息提示”,MessageBoxButtons.OK,MessageBoxIcon.Information);
}
}


}
}

第四个示例是制作一个RealPlay播放器。选择‘工具箱’—‘组件’,右击,选择‘添加/移除项’—‘COM组件‘,把“RealPlayer G2 Control”这个控件加入到控件列表中,就可以使用了。
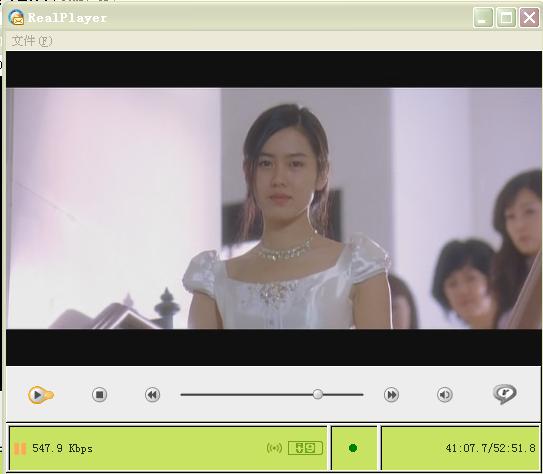
为了简单起见,就直接使用它的控制面板了,代码如下:
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;

namespace RealPlayDemo


{
public class Form1 : System.Windows.Forms.Form

{
private AxRealAudioObjects.AxRealAudio axRealAudio1;

#region
private void InitializeComponent()

{
this.axRealAudio1 = new AxRealAudioObjects.AxRealAudio();
}
#endregion

private void menuItem2_Click(object sender, System.EventArgs e)

{
if(this.openFileDialog1.ShowDialog()==DialogResult.OK)

{
this.axRealAudio1.Source = this.openFileDialog1.FileName;
this.axRealAudio1.DoPlay();
}
}

private void menuItem3_Click(object sender, System.EventArgs e)

{
Application.Exit();
}
}
}

最后一个示例是对第一个Windows Media Play播放器的扩展,用它来制作一个Mp3播放器。选择‘工具箱’—‘组件’,右击,选择‘添加/移除项’—‘COM组件‘,把“Windows Media Play”这个控件加入到控件列表中,就可以使用了。
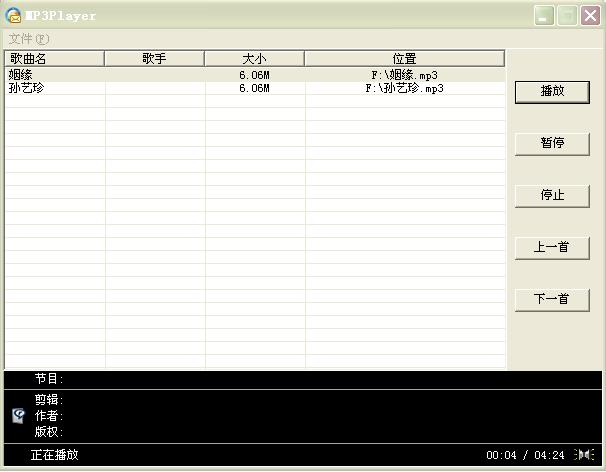
代码如下:
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.IO;

namespace MP3PlayerDemo


{
public class Form1 : System.Windows.Forms.Form

{
private AxMediaPlayer.AxMediaPlayer axMediaPlayer1;

#region
private void InitializeComponent()

{
this.axMediaPlayer1 = new AxMediaPlayer.AxMediaPlayer();
}
#endregion

private void menuItem3_Click(object sender, System.EventArgs e)

{
Application.Exit();
}

private void menuItem2_Click(object sender, System.EventArgs e)

{
if(this.openFileDialog1.ShowDialog()==DialogResult.OK)

{
this.listView1.Items.Clear();
string[] FileNames = this.openFileDialog1.FileNames;
foreach(string fName in FileNames)

{
System.IO.FileInfo fInfo = new FileInfo(fName);
float fSize = (float)fInfo.Length/(1024*1024);
this.axMediaPlayer1.FileName = fName;
this.axMediaPlayer1.Stop();
string author = this.axMediaPlayer1.GetMediaInfoString(MediaPlayer.MPMediaInfoType.mpClipAuthor);
string shortFileName = fName.Substring(fName.LastIndexOf("//")+1);
shortFileName = shortFileName.Substring(0,shortFileName.Length-4);

string[] subItem =
{shortFileName,author,fSize.ToString().Substring(0,4)+"M",fName};
ListViewItem item = new ListViewItem(subItem);
this.listView1.Items.Add(item);
this.listView1.Items[0].Selected = true;
}

}
}

private void btnPlay_Click(object sender, System.EventArgs e)

{
if(this.listView1.Items.Count>0)

{
if(this.listView1.SelectedItems.Count>0)

{
int pos = this.listView1.SelectedItems[0].Index;
string fName = this.listView1.Items[pos].SubItems[3].Text;
this.axMediaPlayer1.FileName = fName;
this.axMediaPlayer1.Play();
}
else

{
MessageBox.Show(this,"ÇëÑ¡ÔñÒª²¥•ŵĸèÇú!!!","ÐÅÏ¢Ìáʾ",MessageBoxButtons.OK,MessageBoxIcon.Information);

}
}

}

private void btnPause_Click(object sender, System.EventArgs e)

{
if(this.axMediaPlayer1.FileName.Length>0)

{
this.axMediaPlayer1.Pause();
}
else

{
MessageBox.Show(this,"请选择要播放的歌曲!!!","信息提示",MessageBoxButtons.OK,MessageBoxIcon.Information);

}
}

private void btnStop_Click(object sender, System.EventArgs e)

{
if(this.axMediaPlayer1.FileName.Length>0)

{
this.axMediaPlayer1.Stop();
}
else

{
MessageBox.Show(this," 请选择要播放的歌曲!!!"," 信息提示",MessageBoxButtons.OK,MessageBoxIcon.Information);

}
}

private void btnLast_Click(object sender, System.EventArgs e)

{
if(this.listView1.Items.Count>0)

{
if(this.listView1.SelectedItems.Count>0)

{
int pos = this.listView1.SelectedItems[0].Index;
if(pos>0)

{
this.listView1.Items[pos-1].Selected = true;
string fName = this.listView1.Items[pos-1].SubItems[3].Text;
this.axMediaPlayer1.FileName = fName;
this.axMediaPlayer1.Play();
}
else

{
MessageBox.Show(this,"已经是第一首歌曲了!!!"," 信息提示",MessageBoxButtons.OK,MessageBoxIcon.Information);

}
}
else

{
MessageBox.Show(this," 请选择要播放的歌曲!!!"," 信息提示",MessageBoxButtons.OK,MessageBoxIcon.Information);

}

}

}

private void btnNext_Click(object sender, System.EventArgs e)

{
if(this.listView1.Items.Count>0)

{
if(this.listView1.SelectedItems.Count>0)

{
int pos = this.listView1.SelectedItems[0].Index;
if(pos<this.listView1.Items.Count-1)

{
this.listView1.Items[pos+1].Selected = true;
string fName = this.listView1.Items[pos+1].SubItems[3].Text;
this.axMediaPlayer1.FileName = fName;
this.axMediaPlayer1.Play();
}
else

{
MessageBox.Show(this,"已经是最后一首歌曲了!!!","信息提示",MessageBoxButtons.OK,MessageBoxIcon.Information);

}
}
else

{
MessageBox.Show(this," 请选择要播放的歌曲!!!","信息提示",MessageBoxButtons.OK,MessageBoxIcon.Information);

}

}
}

}
}




