线程 Thread Runnable Join
来源:互联网 发布:淘宝内部优惠券口令 编辑:程序博客网 时间:2024/05/01 00:58
1.main是主线程,使用继承Thread的方法,无法多线程之间共享实例属性,因为每new一个Thread有自己的属性i,所以不共享。
class FirstThread extends Thread{private int i;//重写run方法public void run(){for(i=0; i<100; i++){System.out.println(getName() + " " +i); //getName返回该线程的名字}}public static void main(String[] args) {for(int i=0; i<100; i++){System.out.println(Thread.currentThread().getName() + " " + i); //当前线程名字if(i==20){new FirstThread().start(); //创建启动第一条线程new FirstThread().start(); //创建启动第二条线程}}}}
输出:
---------- 运行Java程序 ----------
main 0
main 1
main 2
main 3
main 4
main 5
main 6
main 7
main 8
main 9
main 10
main 11
main 12
main 13
main 14
main 15
main 16
main 17
main 18
main 19
main 20
Thread-0 0
Thread-0 1
main 21
Thread-0 2
main 22
Thread-0 3
Thread-0 4
Thread-1 0
Thread-1 1
Thread-1 2
Thread-1 3
........省略
2.继承Runnable接口的方法实现线程,由于创建线程时时同一个target的st,所以线程之间共享i,是连续的
class SecondThread implements Runnable{private int i;public void run(){for(; i<100; i++){System.out.println(Thread.currentThread().getName() + " " + i);}}public static void main(String[] args) {for(int i=0; i<100; i++){System.out.println(Thread.currentThread().getName() + " " + i);if(i==20){SecondThread st = new SecondThread();//(Runnable target, String name) SeconThread是同一个target,共享inew Thread(st, "新线程1").start(); new Thread(st, "新线程2").start();}}}}
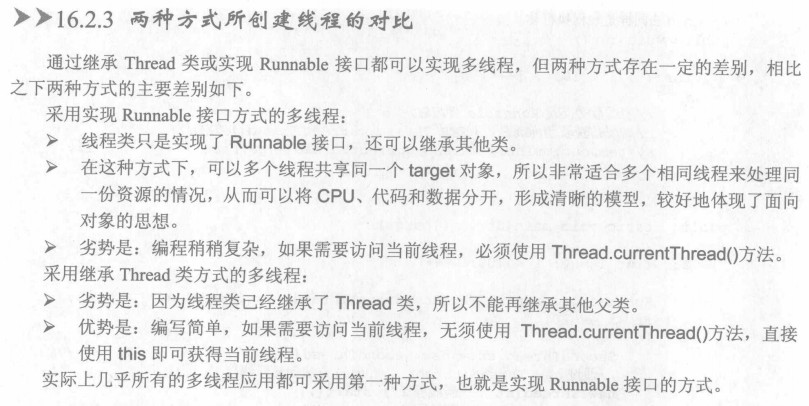
线程的生命周期
新建New
就绪Runnable start()以后处于就绪状态
运行running 执行run()方法时
阻塞Blocked 调用sleep等方法
死亡Dead run()方法执行完,异常,stop()等
控制线程 Join
main线程中调另一个线程jt.join(),main要等jt执行完毕才能执行
class JoinThread extends Thread {public JoinThread(String name){super(name);}public void run(){for(int i=0; i<100; i++){System.out.println(getName() + " " + i);}}public static void main(String[] args) throws Exception{new JoinThread("新线程").start();for(int i=0; i<100; i++){if(i==20){JoinThread jt = new JoinThread("被Join线程");jt.start();//main线程调用jt的join方法,所以main线程要等jt执行完毕后才能执行jt.join(); }System.out.println(Thread.currentThread().getName() + " " + i);}}}
后台线程
所有前台线程死亡后,后台线程就自动死亡,JVM的垃圾回收线程就是典型的后台线程
setDaemon必须在start之前设置
Thread t = new Thread();t.setDaemon(true);t.start();
线程睡眠
暂停一段时间
public void run(){for(int i=0; i<100; i++){System.out.println(getName() + " " + i);Thead.sleep(1000); //暂停1秒}}
线程的让步
Thread.yield()优先级高的线程优先执行,高级执行完再执行低级,低级暂定
线程优先级设置
setPriority()
class ThreadYield extends Thread{private int i;ThreadYield(String name){super(name);}public void run(){for(; i<100; i++){System.out.println(getName() + " " + i);if(i==0){Thread.yield();}}}public static void main(String[] args) {ThreadYield ty1 = new ThreadYield("高级");//ty1.setPriority(Thread.MAX_PRIORITY);ty1.start();ThreadYield ty2 = new ThreadYield("低级");//ty2.setPriority(Thread.MIN_PRIORITY);ty2.start();}}
线程的同步
不同时,num的值小于0了,还能继续计算,导致出错
class ThreadSafe implements Runnable{int num = 10;public void run(){for(int i=0; i<20; i++){if(num>0){try{Thread.sleep(1000);}catch(Exception e){e.printStackTrace();}System.out.println("tickets:" + num--);}}}public static void main(String[] args) {ThreadSafe t = new ThreadSafe();Thread A = new Thread(t);Thread B = new Thread(t);Thread C = new Thread(t);Thread D = new Thread(t);A.start();B.start();C.start();D.start();}}
---------- 运行Java程序 ----------
tickets:10
tickets:10
tickets:8
tickets:9
tickets:7
tickets:5
tickets:6
tickets:7
tickets:4
tickets:3
tickets:4
tickets:2
tickets:1
tickets:0
tickets:1
tickets:-1
输出完成 (耗时 4 秒) - 正常终止
同步代码块
synchronized(Object){ } Object为任意代码块,标识位为0正在其他线程运行,1处于就绪状态
class ThreadSafe implements Runnable{int num = 10;public void run(){synchronized(""){for(int i=0; i<20; i++){if(num>0){try{Thread.sleep(1000);}catch(Exception e){e.printStackTrace();}System.out.println("tickets:" + num--);}}}}public static void main(String[] args) {ThreadSafe t = new ThreadSafe();Thread A = new Thread(t);Thread B = new Thread(t);Thread C = new Thread(t);Thread D = new Thread(t);A.start();B.start();C.start();D.start();}}
---------- 运行Java程序 ----------
tickets:10
tickets:9
tickets:8
tickets:7
tickets:6
tickets:5
tickets:4
tickets:3
tickets:2
tickets:1
输出完成 (耗时 10 秒) - 正常终止
同步方法
run方法不能设置为同步方法
class ThreadSafe implements Runnable{int num = 10;public void run(){doit();}public synchronized void doit(){for(int i=0; i<20; i++){if(num>0){try{Thread.sleep(1000);}catch(Exception e){e.printStackTrace();}System.out.println("tickets:" + num--);}}}public static void main(String[] args) {ThreadSafe t = new ThreadSafe();Thread A = new Thread(t);Thread B = new Thread(t);Thread C = new Thread(t);Thread D = new Thread(t);A.start();B.start();C.start();D.start();}}
---------- 运行Java程序 ----------
tickets:10
tickets:9
tickets:8
tickets:7
tickets:6
tickets:5
tickets:4
tickets:3
tickets:2
tickets:1
输出完成 (耗时 10 秒) - 正常终止
同步锁 Lock
ReentrantLock类,lock()和unlock()方法
import java.util.concurrent.locks.ReentrantLock;class ThreadSafe implements Runnable{private final ReentrantLock lock = new ReentrantLock();int num = 10;public void run(){lock.lock();try{for(int i=0; i<20; i++){if(num>0){try{Thread.sleep(1000);}catch(Exception e){e.printStackTrace();}System.out.println("tickets:" + num--);}}}finally{lock.unlock();}}public static void main(String[] args) {ThreadSafe t = new ThreadSafe();Thread A = new Thread(t);Thread B = new Thread(t);Thread C = new Thread(t);Thread D = new Thread(t);A.start();B.start();C.start();D.start();}}
---------- 运行Java程序 ----------
tickets:10
tickets:9
tickets:8
tickets:7
tickets:6
tickets:5
tickets:4
tickets:3
tickets:2
tickets:1
输出完成 (耗时 10 秒) - 正常终止
- 线程 Thread Runnable Join
- Java.线程.Thread类.Runnable接口.start().setDaemon().join()
- 线程:thread与runnable
- Java 线程 Thread Runnable
- 线程(Thread) 和 Runnable
- java 线程 thread runnable
- 线程(Thread类,Runnable接口)
- Runnable Thread 线程的实现
- Android 线程Thread Runnable解析
- Thread 与 Runnable 线程 初识
- 线程 Thread VS Runnable 基础
- 线程创建Thread和Runnable
- Android线程—Thread、Runnable
- 线程Thread与Runnable实现
- Thread和Runnable创建线程
- 线程 Thread Runnable start run
- 线程Thread与Runnable接口
- 线程终止 Thread.join()
- 启用SQL2008外围管理器
- excel 让单元格上的文字全部显示(英文版)
- http://www.uml.org.cn 学习
- jquery-ajax简单示例
- C#实现并口输出输入高低电位
- 线程 Thread Runnable Join
- javascript鼠标点入input内value值隐藏事件
- Android实现异步加载图片 ListView
- 现代数字影视 电影使用标准
- 坐标变换
- java,hibernate和sql server对应的数据类型
- win 2008R2 下SqlServer 2005 修改密码报错解决方法
- JavaScript File API 文件上传
- java字节流对汉字输出为乱码的问题