堆栈和队列的实现
来源:互联网 发布:淘宝信用货款怎么申请 编辑:程序博客网 时间:2024/04/28 06:21
堆栈和队列都是线性表结构。只不过堆栈的逻辑数据特点是先进后出(FILO),而队列的逻辑数据特点是先进先出(FIFO)。我们先用数组来存放这两种数据结构,也就是线性的存储结构。
请看下例:
public class StackT {private int maxSize; // 堆栈的大小private int[] stackArray;private int top; // 堆栈顶部指针public StackT(int s) {maxSize = s; // 初始化数组大小stackArray = new int[maxSize]; // 初始化一个数组top = -1;}public void push(int j){if (!isFull())stackArray[++top] = j;elsereturn;}public int pop(){return stackArray[top--];}public int peek(){// 得到栈顶的数据而不是出栈return stackArray[top];}public boolean isEmpty(){return (top == -1);}public boolean isFull(){return (top == maxSize - 1);}public String toString(){StringBuffer sb = new StringBuffer();for (int i = top; i >= 0; i--)sb.append("" + stackArray[i] + "\n");return sb.toString();}} // end class StackXclass StackApp {public static void main(String[] args) {StackT theStack = new StackT(10); // 初始化一个堆栈theStack.push(20);theStack.push(40);theStack.push(60);theStack.push(80);System.out.println(theStack);System.out.println("");} // end main()} // end class StackApp
运行的结果如图:
队列的实现(循环队列):
public class Queue {private int maxSize; // 表示队列的大小private int[] queArr; // 用数组来存放有队列的数据private int front; // 取数据的下标private int rear; // 存数据的下标private int nItems; // 记录存放的数据个数public Queue(int s) {maxSize = s;queArr = new int[maxSize];front = 0;rear = -1;nItems = 0;}public void insert(int j) { // 增加数据的方法if (isFull())return;// 如果下标到达数组顶部的话,让rear指向数组的第一个位置之前if (rear == maxSize - 1)rear = -1;queArr[++rear] = j; // increment rear and insertnItems++; // one more item}public int remove(){int temp = queArr[front++];// 如果下标到达数组顶部的话,让front指向数组的第一个位置if (front == maxSize)front = 0;nItems--;return temp;}public int peekFront(){// 只是返回最前面那个元素的值,并不删除return queArr[front];}public boolean isEmpty() {return (nItems == 0);}public boolean isFull() {return (nItems == maxSize);}public int size() {return nItems;}} // end class Queueclass QueueApp {public static void main(String[] args) {Queue theQueue = new Queue(5);theQueue.insert(10); // 插入4个数据theQueue.insert(20);theQueue.insert(30);theQueue.insert(40);theQueue.remove(); // 删除(10, 20, 30)theQueue.remove();theQueue.remove();theQueue.insert(50); // 再插入4个数据theQueue.insert(60);theQueue.insert(70);theQueue.insert(80);while (!theQueue.isEmpty())// 取出所有数据System.out.println(theQueue.remove());} }
运行的结果如图:
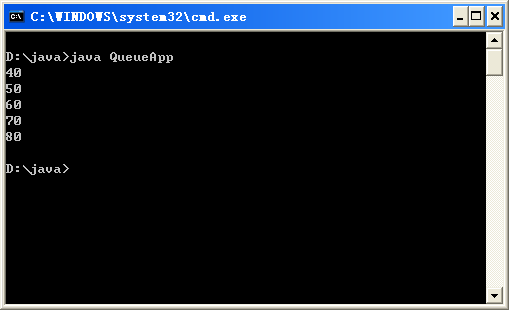
- 堆栈和队列的实现
- 堆栈和队列的实现
- 用双链表实现的堆栈和队列
- 堆栈和队列的java实现
- 堆栈,队列的实现
- Java 实现堆栈和队列
- python实现堆栈和队列
- python实现堆栈和队列
- 关于树的队列实现和堆栈实现的分析
- 堆栈及队列的实现
- 队列和堆栈实现二叉树的遍历
- java中堆栈和队列的实现方式
- 队列和堆栈的区别
- 堆栈和队列的应用
- 用数组实现堆栈和队列
- 回文字(堆栈和队列实现)
- java用链表实现堆栈和队列
- 用数组实现堆栈和队列
- 编译andorid时使用ccache
- MD5加密
- PLSQL Developer连接不上Win7 64为系统下安装的Oracle11g64位的解决办法
- C#byte[]序列化及xml序列化,支持序列化后再压缩.
- oracle及操作系统对于文件大小的限制
- 堆栈和队列的实现
- Flex 修改shape颜色的方法
- js中的escape的用法汇总
- python in or of opencv
- 修改android4.0.3的屏幕超时
- freemarker的内置处理相关数据的函数
- 软RAID 0的技术概要及实现 v0.1b (正在修订之中)
- 单链表的实现
- 写串口程序时waitcommevent或writefile导致死锁问题