C++ standard exceptions
来源:互联网 发布:windows更新后c盘变小 编辑:程序博客网 时间:2024/05/29 16:37
Standard exceptions
The C++ Standard library provides a base class specifically designed to declare objects to be thrown as exceptions. It is called exception and is defined in the <exception> header file under the namespace std. This class has the usual default and copy constructors, operators and destructors, plus an additional virtual member function called what that returns a null-terminated character sequence (char *) and that can be overwritten in derived classes to contain some sort of description of the exception.We have placed a handler that catches exception objects by reference (notice the ampersand & after the type), therefore this catches also classes derived from exception, like our myex object of class myexception.
All exceptions thrown by components of the C++ Standard library throw exceptions derived from thisstd::exception class. These are:
C++ provides a list of standard exceptions defined in <exception> which we can use in our programs. These are arranged in an a parent-child class hierarchy shown below:
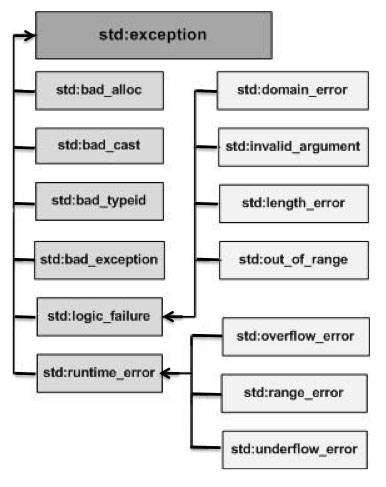
Here is the small description of each exception mentioned in the above hierarchy:
Define New Exceptions:
You can define your own exceptions by inheriting and overriding exception class functionality. Following is the example which shows how you can use std::exception class to implement your own exception in standard way:
#include <iostream>#include <exception>using namespace std;struct MyException : public exception{ const char * what () const throw () { return "C++ Exception"; }}; int main(){ try { throw MyException(); } catch(MyException& e) { std::cout << "MyException caught" << std::endl; std::cout << e.what() << std::endl; } catch(std::exception& e) { //Other errors }}
This would produce following result:
MyException caughtC++ Exception
Here what() is a public method provided by exception class and it has been overridden by all the child exception classes. This returns the cause of an exception.
- C++ standard exceptions
- C++ Standard Exceptions(回顾基础)
- Standard C
- Standard C
- c/c++ Exceptions
- C++--异常(Exceptions)
- C Standard Library
- C language standard headers
- C Standard Library
- The Standard C Library
- C Standard Library
- C Programming Language Standard
- C standard library headers
- C Standard Library
- Standard - C 标准函数库
- C Coding Standard
- Standard - C 标准函数库
- C Code Standard
- Starling:ScrollText
- TinyXML:一个优秀的C++ XML解析器
- Can't create handler inside thread
- java实现简单的单点登录
- hpux 11.11 连接HDS 存储,采用HDLM管理带来的麻烦,需重启。
- C++ standard exceptions
- wdos—linux服务器管理系统
- java内存管理
- struts2配置中Action的name和package的name和namespace的用法,以及extends属性
- Hough变换原理
- .spec文件笔记
- 揭秘程序员大脑编程的七大“误区”
- 第七周项目四——复数模板类
- 关于vmlinux,vmlinuz,bzImage,zImage的区别和联系