UITabBarView(Managing Tabs at Runtime)
来源:互联网 发布:美发大全软件下载 编辑:程序博客网 时间:2024/04/29 17:08
Managing Tabs at Runtime
After creating your tab bar interface, there are several ways to modify it and respond to changes in your app. You can add and remove tabs or use adelegate object to prevent the selection of tabs based on dynamic conditions. You can also add badges to individual tabs to call the user’s attention to that tab.
Adding and Removing Tabs
If the number of tabs in your tab bar interface can change dynamically, you can make the appropriate changes at runtime as needed. You change the tabs at runtime in the same way you specify tabs at creation time, by assigning the appropriate set of view controllers to your tab bar controller. If you are adding or removing tabs in a way that might be seen by the user, you can animate the tab changes using thesetViewControllers:animated:
method.
Listing 2-3 shows a method that removes the currently selected tab in response to a tap in a specific button in the same tab. This method is implemented by the view controller for the tab. You might use something similar in your own code if you want to remove a tab that is no longer needed. For example, you could use it to remove a tab containing some user-specific data that needs to be entered only once.
Listing 2-3 Removing the current tab
- (IBAction)processUserInformation:(id)sender
{
// Call some app-specific method to validate the user data.
// If the custom method returns YES, remove the tab.
if ([self userDataIsValid])
{
NSMutableArray* newArray = [NSMutableArray arrayWithArray:self.tabBarController.viewControllers];
[newArray removeObject:self];
[self.tabBarController setViewControllers:newArray animated:YES];
}
}
Preventing the Selection of Tabs
If you need to prevent the user from selecting a tab, you can do so by providing a delegate object and implementing thetabBarController:shouldSelectViewController:
method on that object. Preventing the selection of tabs should be done only on a temporary basis, such as when a tab does not have any content. For example, if your app requires the user to provide some specific information, such as a login name and password, you could disable all tabs except the one that prompts the user for the required information. Listing 2-4 shows an example of what such a method looks like. The hasValidLogin
method is a custom method that you implement to validate the provided information.
Listing 2-4 Preventing the selection of tabs
- (BOOL)tabBarController:(UITabBarController *)aTabBar
shouldSelectViewController:(UIViewController *)viewController
{
if (![self hasValidLogin] && (viewController != [aTabBar.viewControllers objectAtIndex:0]) )
{
// Disable all but the first tab.
return NO;
}
return YES;
}
Monitoring User-Initiated Tab Changes
There are two types of user-initiated changes that can occur on a tab bar:
The user can select a tab.
The user can rearrange the tabs.
Both types of changes are reported to the tab bar controller’s delegate, which is an object that conforms to the UITabBarControllerDelegate
protocol. You might provide a delegate to keep track of user changes and update your app’s state information accordingly. However, you should not use these notifications to perform work that would normally be handled by the view controllers being hidden and shown. For example, you would not use your tab bar controller delegate to change the appearance of the status bar to match the style of the currently selected view. Visual changes of that nature are best handled by your content view controllers.
For more information about the methods of the UITabBarControllerDelegate
protocol and how you use them, see UITabBarControllerDelegate Protocol Reference.
Preventing the Customization of Tabs
The More view controller provides built-in support for the user to modify the items displayed in the tab bar. For apps with lots of tabs, this support allows the user to pick and choose which screens are readily accessible and which require additional navigation to reach. The left side of Figure 2-5shows the More selection screen displayed by the iPod app. When the user taps the Edit button in the upper-left corner of this screen, the More controller automatically displays the configuration screen shown on the right. From this screen, the user can replace the contents of the tab bar by dragging new items to it.
Figure 2-5 Configuring the tab bar of the iPod app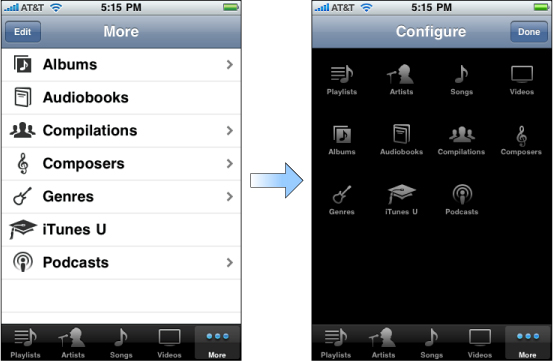
While it is a good idea to let the user rearrange tabs most of the time, there may be situations where you might not want the user to remove specific tabs from the tab bar or place specific tabs on the tab bar. In these situations, you can assign an array of view controller objects to thecustomizableViewControllers
property. This array should contain the subset of view controllers that it is all right to rearrange. View controllers not in the array are not displayed on the configuration screen and cannot be removed from the tab bar if they are already there.
Important: Adding or removing view controllers in your tab bar interface also resets the array of customizable view controllers to the default value, allowing all view controllers to be customized again. Therefore, if you make modifications to the viewControllers
property (either directly or by calling the setViewControllers:animated:
method) and still want to limit the customizable view controllers, you must also update the array of objects in the customizableViewControllers
property.
Changing a Tab’s Badge
The appearance of a tab in a tab bar interface normally does not change, except when it is selected. To call attention to a specific tab, perhaps because there is new content on that tab for the user to look at, you can use a badge.
A badge is a small red marker displayed in the corner of the tab. Inside the badge is some custom text that you provide. Typically, badges contain numerical values reflecting the number of new items available on the tab, but you can also specify very short character strings too. Figure 2-6 shows badges for tabs in the Phone app.
Figure 2-6 Badges for tab bar items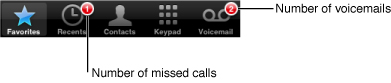
To assign a badge to a tab, assign a non-nil value to the badgeValue
property of the corresponding tab bar item. Listing 2-5 shows an example of how a view controller that displays the number of new items in its badge might set the badge value.
Listing 2-5 Setting a tab’s badge
if (numberOfNewItems == 0)
self.tabBarItem.badgeValue = nil;
else
self.tabBarItem.badgeValue = [NSString stringWithFormat:@"%d", numberOfNewItems];
It is up to you to decide when to display badge values and to update the badge value at the appropriate times. However, if your view controller contains a property with such a value, you can use key-value observing (KVO) notifications to detect changes to the value and update the badge accordingly. For information about setting up and handling KVO notifications, see Key-Value Observing Programming Guide.
Tab Bar Controllers and View Rotation
Tab bar controllers support a portrait orientation by default and do not rotate to a landscape orientation unless all of the contained view controllers support such an orientation. When a device orientation change occurs, the tab bar controller queries its array of view controllers. If any one of them does not support the orientation, the tab bar controller does not change its orientation.
Tab Bars and Full-Screen Layout
Tab bar controllers support full-screen layout differently from the way most other controllers support it. You can still set thewantsFullScreenLayout
property of your content view controller to YES
if you want its view to underlap the status bar or a navigation bar (if present). However, setting this property to YES
does not cause the view to underlap the tab bar view. The tab bar controller always resizes your view to prevent it from underlapping the tab bar.
For more information on full-screen layout for custom views, see “Creating Custom Content View Controllers” in View Controller Programming Guide for iOS.
- UITabBarView(Managing Tabs at Runtime)
- How to Cheat at Managing Information Security
- Resizing Controls at Runtime
- Switching Cameras at runtime
- Skipping tests at runtime
- Android: Tabs at the bottom with FragmentTabHost
- Tomcat reload class at runtime
- Change Locale at the runtime
- Compiling C++ code at runtime
- Unity Loading Resources at Runtime
- Create class at OC runtime
- UITabBarView 添加自定义图片
- Creating and managing container children at run time
- Tabs
- Tabs
- Tabs
- Changing Target Web Service At Runtime
- Adding Controls to a DataGrid at Runtime
- ASP.NET实验五:实现输入内容提示的功能(仿google_百度输入框提示)
- windows进程通信之共享内存那点事
- hdu1319 Prime Cuts
- css透明---css样式之美
- 操作系统概论二
- UITabBarView(Managing Tabs at Runtime)
- PHP自学之路-----接口方法
- hdu4545(魔法串)
- S3C6410启动模式介绍
- 我知道我是各种被虐。
- vc++External Dependencies
- chrome、firefox、IE 处理input 光标的区别
- jquery实现自动提示
- MSHID->Linux KCL->Android KCL Mapping