Using UIPageControl as a container UIViewController
来源:互联网 发布:linux怎么配置jdk 编辑:程序博客网 时间:2024/06/14 09:10
Using UIPageControl as a container UIViewController
虽然看上去用 UIPageControl 在一系列 UIView或UIViewController中导航是很平常的事情,但实际上 Apple公司并没有提供一个这样的方法或者演示Demo:
在最新的iOS版本中(5.0 现在已经不是最新的),Apple公司提供了很多如何用其他方式实现
UIViewController容器的方式(可以从这里参考),但悲剧的是它们与 UIPageControl 没多大关系。
那么我们今天就来专门解决这个问题。
创建界面
首先我们需要在 Interface Builder 中在一个UIViewController里面创建一个UIPageControl与UIScrollView。当然你可以创建很多类似的UIViewController。
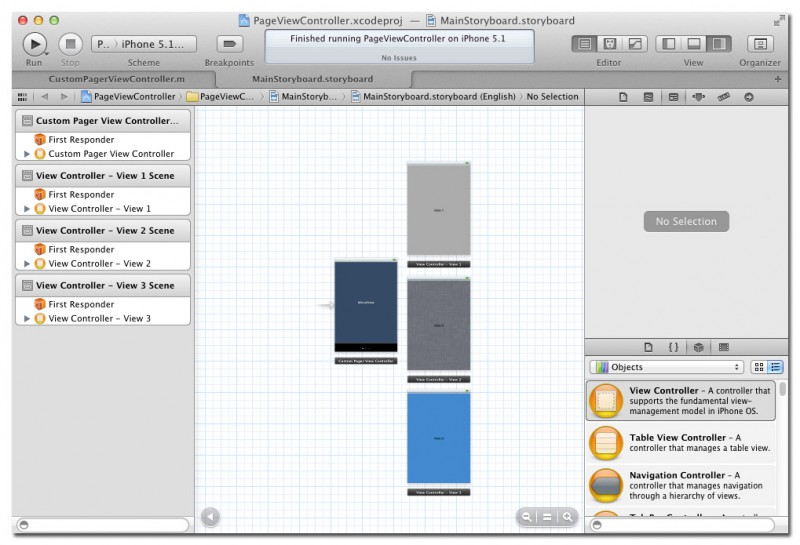
设置 PageViewController 类
这里的 PageViewController 对象将包含一个 UIScrollView 与一个 UIPageControl。而且 UIScrollView 将会包含所有的 subview, 这些subview就是被UIPageControl控制的子页面。
你也许会在 viewController 中添加一些算法来专门处理页面转换: addChildViewController:方法。然而在我这个案例中却不是这么做的,来一起看看代码:
- @interface PagerViewController : UIViewController
- @property (nonatomic, strong) IBOutlet UIScrollView *scrollView;
- @property (nonatomic, strong) IBOutlet UIPageControl *pageControl;
- - (IBAction)changePage:(id)sender;
- @end
在实现的代码文件中,首先我先不让它能够旋转屏幕。然后当 PagerViewController 预备显示时,我们需要标记一下当前哪一个view是被激活的,即被显示的,那么需要在 viewDidApplear 以及 viewWillDisappear方法中加入以下代码:
- - (BOOL)automaticallyForwardAppearanceAndRotationMethodsToChildViewControllers {
- returnNO;
- }
- - (void)viewDidAppear:(BOOL)animated {
- [super viewDidAppear:animated];
- UIViewController *viewController = [self.childViewControllers objectAtIndex:self.pageControl.currentPage];
- if(viewController.view.superview != nil) {
- [viewController viewDidAppear:animated];
- }
- }
- - (void)viewWillDisappear:(BOOL)animated {
- UIViewController *viewController = [self.childViewControllers objectAtIndex:self.pageControl.currentPage];
- if(viewController.view.superview != nil) {
- [viewController viewWillDisappear:animated];
- }
- [super viewWillDisappear:animated];
- }
- - (void)viewDidDisappear:(BOOL)animated {
- UIViewController *viewController = [self.childViewControllers objectAtIndex:self.pageControl.currentPage];
- if(viewController.view.superview != nil) {
- [viewController viewDidDisappear:animated];
- }
- [super viewDidDisappear:animated];
- }
viewWillAppear 可能会有点复杂,因为我们也需要加载所有的子view到 scrollView中,而且必须确保scrollview的contentsize比子view要大。
- - (void)viewWillAppear:(BOOL)animated {
- [super viewWillAppear:animated];
- for(NSUInteger i =0; i < [self.childViewControllers count]; i++) {
- [self loadScrollViewWithPage:i];
- }
- self.pageControl.currentPage = 0;
- _page = 0;
- [self.pageControl setNumberOfPages:[self.childViewControllers count]];
- UIViewController *viewController = [self.childViewControllers objectAtIndex:self.pageControl.currentPage];
- if(viewController.view.superview != nil) {
- [viewController viewWillAppear:animated];
- }
- self.scrollView.contentSize = CGSizeMake(scrollView.frame.size.width * [self.childViewControllers count], scrollView.frame.size.height);
- }
为了将UIViewController的内容加载到UIScrolView的contentView中,需要如下代码:
- - (void)loadScrollViewWithPage:(int)page {
- if(page < 0)
- return;
- if(page >= [self.childViewControllers count])
- return;
- // replace the placeholder if necessary
- UIViewController *controller = [self.childViewControllers objectAtIndex:page];
- if(controller == nil) {
- return;
- }
- // add the controller's view to the scroll view
- if(controller.view.superview == nil) {
- CGRect frame = self.scrollView.frame;
- frame.origin.x = frame.size.width * page;
- frame.origin.y = 0;
- controller.view.frame = frame;
- [self.scrollView addSubview:controller.view];
- }
- }
处理滚动
为了处理滚动,我们需要实现几个 UIScrollViewDelegate 的方法以及尽早声明方法 - (IBAction)changePage:(id)sender
首先必须先知道滚动是如何实现的,要么是手势,要么就是点击了 UIPageControl 的一侧。
来一起看看下面的代码:
- // At the begin of scroll dragging, reset the boolean used when scrolls originate from the UIPageControl
- - (void)scrollViewWillBeginDragging:(UIScrollView *)scrollView {
- _pageControlUsed = NO;
- }
- // At the end of scroll animation, reset the boolean used when scrolls originate from the UIPageControl
- - (void)scrollViewDidEndDecelerating:(UIScrollView *)scrollView {
- _pageControlUsed = NO;
- }
- - (void)scrollViewDidEndScrollingAnimation:(UIScrollView *)scrollView {
- UIViewController *oldViewController = [self.childViewControllers objectAtIndex:_page];
- UIViewController *newViewController = [self.childViewControllers objectAtIndex:self.pageControl.currentPage];
- [oldViewController viewDidDisappear:YES];
- [newViewController viewDidAppear:YES];
- _page = self.pageControl.currentPage;
- }
现在,为了当页面改变时也能更新当前的显示,我们只需要实现 scrollViewDidScroll delegate方法以及 changePage IBAction 方法。
- - (IBAction)changePage:(id)sender {
- intpage = ((UIPageControl *)sender).currentPage;
- // update the scroll view to the appropriate page
- CGRect frame = self.scrollView.frame;
- frame.origin.x = frame.size.width * page;
- frame.origin.y = 0;
- UIViewController *oldViewController = [self.childViewControllers objectAtIndex:_page];
- UIViewController *newViewController = [self.childViewControllers objectAtIndex:self.pageControl.currentPage];
- [oldViewController viewWillDisappear:YES];
- [newViewController viewWillAppear:YES];
- [self.scrollView scrollRectToVisible:frame animated:YES];
- // Set the boolean used when scrolls originate from the UIPageControl. See scrollViewDidScroll: above.
- _pageControlUsed = YES;
- }
- - (void)scrollViewDidScroll:(UIScrollView *)sender {
- // We don't want a "feedback loop" between the UIPageControl and the scroll delegate in
- // which a scroll event generated from the user hitting the page control triggers updates from
- // the delegate method. We use a boolean to disable the delegate logic when the page control is used.
- if(_pageControlUsed || _rotating) {
- // do nothing - the scroll was initiated from the page control, not the user dragging
- return;
- }
- // Switch the indicator when more than 50% of the previous/next page is visible
- CGFloat pageWidth = self.scrollView.frame.size.width;
- intpage = floor((self.scrollView.contentOffset.x - pageWidth / 2) / pageWidth) + 1;
- if(self.pageControl.currentPage != page) {
- UIViewController *oldViewController = [self.childViewControllers objectAtIndex:self.pageControl.currentPage];
- UIViewController *newViewController = [self.childViewControllers objectAtIndex:page];
- [oldViewController viewWillDisappear:YES];
- [newViewController viewWillAppear:YES];
- self.pageControl.currentPage = page;
- [oldViewController viewDidDisappear:YES];
- [newViewController viewDidAppear:YES];
- _page = page;
- }
- }
最后处理旋转
首先在shouldAutorotateToInterfaceOrientation方法中返回 YES,同时也需要传输一下3个消息给当前被显示的子 UIViewController。
- willAnimateRotationToInterfaceOrientation:duration:
- willRotateToInterfaceOrientation:duration:
- didRotateFromInterfaceOrientation:
但我们还是要自己处理旋转的问题,比如要重新缩放scrollview的contentView,以及调整子view的帧率,否则会出现一些无法预料的事情。所以来看看以下处理方式
- - (BOOL)shouldAutorotateToInterfaceOrientation:(UIInterfaceOrientation)interfaceOrientation
- {
- returnYES;
- }
- - (void)willRotateToInterfaceOrientation:(UIInterfaceOrientation)toInterfaceOrientation duration:(NSTimeInterval)duration {
- UIViewController *viewController = [self.childViewControllers objectAtIndex:self.pageControl.currentPage];
- [viewController willRotateToInterfaceOrientation:toInterfaceOrientation duration:duration];
- _rotating = YES;
- }
- - (void)willAnimateRotationToInterfaceOrientation:(UIInterfaceOrientation)toInterfaceOrientation duration:(NSTimeInterval)duration {
- UIViewController *viewController = [self.childViewControllers objectAtIndex:self.pageControl.currentPage];
- [viewController willAnimateRotationToInterfaceOrientation:toInterfaceOrientation duration:duration];
- self.scrollView.contentSize = CGSizeMake(scrollView.frame.size.width * [self.childViewControllers count], scrollView.frame.size.height);
- NSUInteger page = 0;
- for(viewController in self.childViewControllers) {
- CGRect frame = self.scrollView.frame;
- frame.origin.x = frame.size.width * page;
- frame.origin.y = 0;
- viewController.view.frame = frame;
- page++;
- }
- CGRect frame = self.scrollView.frame;
- frame.origin.x = frame.size.width * _page;
- frame.origin.y = 0;
- [self.scrollView scrollRectToVisible:frame animated:NO];
- }
- - (void)didRotateFromInterfaceOrientation:(UIInterfaceOrientation)fromInterfaceOrientation {
- _rotating = NO;
- UIViewController *viewController = [self.childViewControllers objectAtIndex:self.pageControl.currentPage];
- [viewController didRotateFromInterfaceOrientation:fromInterfaceOrientation];
- }
总结
废话少说,这里放出代码,在GitHub上- Using UIPageControl as a container UIViewController
- Using UIPageControl as a container UIViewController
- Using UIPageControl as a container UIViewController
- 09 Container as a Service
- Using Emacs as a server
- Using MySQL as a NoSQL
- Using XML as a data storage format
- Using PHP As A Shell Scripting Language
- Using Nginx as a load balancer
- display month as a calendar using sql
- display month as a calendar using sql
- Using dd as a Swiss Army knife
- Using C++ std::equal on a container of shared_ptr
- 如何将UIPageControl作为 UIViewController的容器
- 如何将UIPageControl作为 UIViewController的容器
- Using Oracle XML DB Repository as a Filesystem
- Using OTRS with Active Directory as a source for agents
- Using VB.NET 2008 DLL as a COM DLL
- Android VSync信号产生过程源码分析
- android 如何退出整个系统
- 静态方法和非静态方法的调用
- 并发编程之交换器Exchanger
- HTML相关
- Using UIPageControl as a container UIViewController
- boost重新编译
- facebook presto 安装
- JavaScript创建日志文件并记录时间的做法
- poj2155 二维线段树
- apk文件伪加密
- 倒地老人,你扶还是不扶?
- Linux驱动学习5(详细分析字符设备驱动信号量实现互斥)
- DevKit8500开发板-NFS挂载网络文件系统