Android中的TabHost
来源:互联网 发布:apache storm 源码 编辑:程序博客网 时间:2024/06/05 16:09
介绍
有时,我们想在一个window中显示多个视图,这时就需要用到Tab容器。在Android里它叫TabHost。
使用TabHost有两种方式:
- 在相同的activity中使用TabHost导航多个视图
- 使用TabHost导航多个Activity(通过intents)
Tab应用的结构
TabHost的Activity的结构如下:
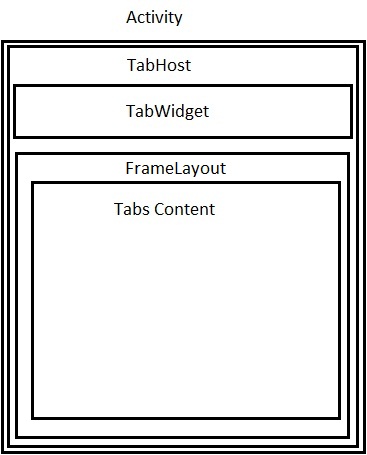
先看个示例:
layout文件:
- <?xml version="1.0" encoding="utf-8"?>
- <TabHost android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- android:id="@+id/tabHost"
- xmlns:android="http://schemas.android.com/apk/res/android"
- >
- <TabWidget
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:id="@android:id/tabs"
- />
- <FrameLayout
- android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- android:id="@android:id/tabcontent"
- >
- <LinearLayout
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:id="@+id/tab1"
- android:orientation="vertical"
- android:paddingTop="60px"
- >
- <TextView
- android:layout_width="fill_parent"
- android:layout_height="100px"
- android:text="This is tab1"
- android:id="@+id/txt1"
- />
- </LinearLayout>
- <LinearLayout
- android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- android:id="@+id/tab2"
- android:orientation="vertical"
- android:paddingTop="60px"
- >
- <TextView
- android:layout_width="fill_parent"
- android:layout_height="100px"
- android:text="This is tab 2"
- android:id="@+id/txt2"
- />
- </LinearLayout>
- <LinearLayout
- android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- android:id="@+id/tab3"
- android:orientation="vertical"
- android:paddingTop="60px"
- >
- <TextView
- android:layout_width="fill_parent"
- android:layout_height="100px"
- android:text="This is tab 3"
- android:id="@+id/txt3"
- />
- </LinearLayout>
- </FrameLayout>
- </TabHost>
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.main);
- TabHost tabHost=(TabHost)findViewById(R.id.tabHost);
- tabHost.setup();
- TabSpec spec1=tabHost.newTabSpec("Tab 1");
- spec1.setContent(R.id.tab1);
- spec1.setIndicator("Tab 1");
- TabSpec spec2=tabHost.newTabSpec("Tab 2");
- spec2.setIndicator("Tab 2");
- spec2.setContent(R.id.tab2);
- TabSpec spec3=tabHost.newTabSpec("Tab 3");
- spec3.setIndicator("Tab 3");
- spec3.setContent(R.id.tab3);
- tabHost.addTab(spec1);
- tabHost.addTab(spec2);
- tabHost.addTab(spec3);
- }
- 这里通过TabSpecs类创建Tab
- 使用setIndicator方法设置tab的文字
- 使用setContent设置tab的内容
- 如果你使用TabActivity作为你的Activity的基类,你不用调用TabHost.Setup()方法。
运行后看起来是这样的:
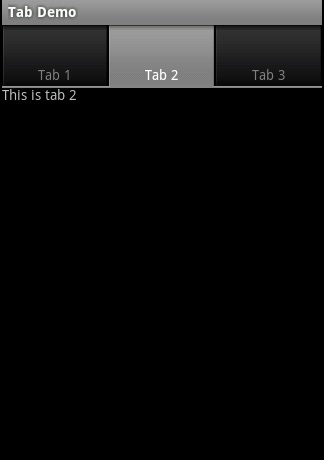
同时还可以指定indicator为一个view:
- TabSpec spec1=tabHost.newTabSpec("Tab 1");
- spec1.setContent(R.id.tab1);
- TextView txt=new TextView(this);
- txt.setText("Tab 1");
- txt.setBackgroundColor(Color.RED);
- spec1.setIndicator(txt);
设置tab的内容
上面的例子展示了使用tab显示不同的layout资源。如果我们需要通过tab导航到不同的Activity,该怎么办?
这种情况,我们需要有一个activity作为应用的根activity。这个Activity包含TabHost,通过intents导航不同的activity。
注意:根Activity必须继承TabActivity。代码如下:
Layout:
- <?xml version="1.0" encoding="utf-8"?>
- <TabHost android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- android:id="@android:id/tabhost"
- xmlns:android="http://schemas.android.com/apk/res/android"
- >
- <TabWidget
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:id="@android:id/tabs"
- />
- <FrameLayout
- android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- android:id="@android:id/tabcontent"
- >
- </FrameLayout>
- </TabHost>
Activity:
- public class TabDemo extends TabActivity {
- /** Called when the activity is first created. */
- @Override
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.main);
- TabHost tabHost=getTabHost();
- // no need to call TabHost.Setup()
- //First Tab
- TabSpec spec1=tabHost.newTabSpec("Tab 1");
- spec1.setIndicator("Tab 1",getResources().getDrawable(R.drawable.sun));
- Intent in1=new Intent(this, Act1.class);
- spec1.setContent(in1);
- TabSpec spec2=tabHost.newTabSpec("Tab 2");
- spec2.setIndicator("Tab 2",getResources().getDrawable(R.drawable.chart));
- Intent in2=new Intent(this,Act2.class);
- spec2.setContent(in2);
- tabHost.addTab(spec2);
- tabHost.addTab(spec3);
- }
- }

在运行时添加Tab
在运行时我们可以通过调用TabSepc.setContent(TabContentFactory)方法添加Tab。
- <span style="white-space:pre"> </span>TabSpec spec1=tabHost.newTabSpec("Tab 1");
- spec1.setIndicator("Tab 1",getResources().getDrawable(R.drawable.sun));
- spec1.setContent(new TabContentFactory() {
- <span style="white-space:pre"> </span> @Override
- <span style="white-space:pre"> </span> public View createTabContent(String tag) {
- <span style="white-space:pre"> </span> // TODO Auto-generated method stub
- <span style="white-space:pre"> </span> return (new AnalogClock(TabDemo.this));
- <span style="white-space:pre"> </span> }
- <span style="white-space:pre"> </span> });
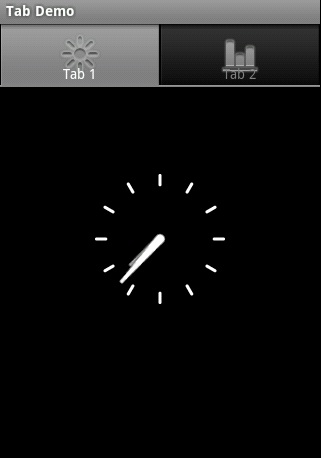
0 0
- Android中的TabHost
- Android中的TabHost
- Android中的TabHost
- Android中的TabHost
- Android中的TabHost
- Android中的TabHost
- Android 中的TabHost控件的使用
- Android tabhost
- Android:TabHost
- TabHost Android
- Android TabHost
- Android TabHost
- android--tabhost
- android tabhost
- android Tabhost
- Android TabHost
- android tabhost
- Android TabHost
- oracle中rownum详解
- CF 443B(253B)Kolya and Tandem Repeat
- winform 实现选择城市列表
- adb在安卓avd安装apk文件
- 图书管理系统
- Android中的TabHost
- Unity3d之生命槽设置
- WildFly角色分配
- 查找字符
- 点击PopupWindow之外的区域让其消失
- USB HID Report Descriptor 报告描述符详解(节选自HID1.11协议)
- 什么是出色的员工
- Android开发历程_1(从1个activity跳转到另一个activity)
- /,/,//