UVA 11992- Fast Matrix Operations(线段树区间各种操作)
来源:互联网 发布:北京股商怎么样知乎 编辑:程序博客网 时间:2024/06/07 06:13
Description
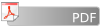
Problem F
Fast Matrix Operations
There is a matrix containing at most 106 elements divided into r rows and c columns. Each element has a location (x,y) where 1<=x<=r,1<=y<=c. Initially, all the elements are zero. You need to handle four kinds of operations:
1 x1 y1 x2 y2 v
Increment each element (x,y) in submatrix (x1,y1,x2,y2) by v (v>0)
2 x1 y1 x2 y2 v
Set each element (x,y) in submatrix (x1,y1,x2,y2) to v
3 x1 y1 x2 y2
Output the summation, min value and max value of submatrix (x1,y1,x2,y2)
In the above descriptions, submatrix (x1,y1,x2,y2) means all the elements (x,y) satisfying x1<=x<=x2 and y1<=x<=y2. It is guaranteed that 1<=x1<=x2<=r, 1<=y1<=y2<=c. After any operation, the sum of all the elements in the matrix does not exceed 109.
Input
There are several test cases. The first line of each case contains three positive integers r, c, m, where m (1<=m<=20,000) is the number of operations. Each of the next m lines contains a query. There will be at most twenty rows in the matrix. The input is terminated by end-of-file (EOF). The size of input file does not exceed 500KB.
Output
For each type-3 query, print the summation, min and max.
Sample Input
4 4 81 1 2 4 4 53 2 1 4 41 1 1 3 4 23 1 2 4 43 1 1 3 42 2 1 4 4 23 1 2 4 41 1 1 4 3 3
Output for the Sample Input
45 0 578 5 769 2 739 2 7
思路:线段树的区间的值add 和 set ,区间求最大值,最小值,总和。
注意:当add 和set 混合使用的时候应该注意在存在set 之后应该将之前的add 清空,但是注意不要将后面的add 清零~在代码中已有注释。
CODE:
#include <iostream>#include <cstdio>#include <algorithm>#include <cmath>#include <string>#include <cstring>#include <queue>#include <stack>#include <vector>#include <set>#include <map>const int inf=1000000009;typedef long long ll;using namespace std;const int Max=60005;int R,C,M,q;int sum,minn,maxn;struct SegmentTree{ int sumv[Max<<2]; int addv[Max<<2]; int setv[Max<<2]; int maxv[Max<<2]; int minv[Max<<2]; void maintain(int pos){ sumv[pos]=sumv[pos*2]+sumv[pos*2+1]; maxv[pos]=max(maxv[pos*2],maxv[pos*2+1]); minv[pos]=min(minv[pos*2],minv[pos*2+1]); } void build(int pos,int l,int r){ setv[pos]=addv[pos]=0; if(l==r){ sumv[pos]=maxv[pos]=minv[pos] =setv[pos]=addv[pos]=0; } else{ int mid=(l+r)/2; build(pos*2,l,mid); build(pos*2+1,mid+1,r); maintain(pos); } } void pushdown(int pos,int l,int r){ int mid=(l+r)/2; if(setv[pos]!=0){ setv[pos*2]=setv[pos*2+1]=setv[pos]; sumv[pos*2]=(mid-l+1)*setv[pos]; sumv[pos*2+1]=(r-mid)*setv[pos]; minv[pos*2]=minv[pos*2+1]=setv[pos]; maxv[pos*2]=maxv[pos*2+1]=setv[pos]; setv[pos]=0; addv[pos*2]=addv[pos*2+1]=0;//记得清0~ } if(addv[pos]!=0){ addv[pos*2]+=addv[pos]; addv[pos*2+1]+=addv[pos]; sumv[pos*2]+=(mid-l+1)*addv[pos]; sumv[pos*2+1]+=(r-mid)*addv[pos]; minv[pos*2]+=addv[pos]; minv[pos*2+1]+=addv[pos]; maxv[pos*2]+=addv[pos]; maxv[pos*2+1]+=addv[pos]; addv[pos]=0; } } void update(int pos,int l,int r,int x,int y,int v){ if(x<=l && y>=r){ if(q==1){ addv[pos]+=v; sumv[pos]+=(r-l+1)*v; minv[pos]+=v; maxv[pos]+=v; } else{ setv[pos]=v; sumv[pos]=(r-l+1)*v; minv[pos]=v; maxv[pos]=v; addv[pos]=0;//同样要记得清零! } } else{ pushdown(pos,l,r); int mid=(l+r)/2; if(x<=mid) update(pos*2,l,mid,x,y,v); if(y>mid) update(pos*2+1,mid+1,r,x,y,v); maintain(pos); } } void query(int pos,int l,int r,int x,int y){ if(x<=l && y>=r){ sum+=sumv[pos]; minn=min(minn,minv[pos]); maxn=max(maxn,maxv[pos]); } else{ pushdown(pos,l,r); int mid=(l+r)/2; if(x<=mid) query(pos*2,l,mid,x,y); if(y>mid) query(pos*2+1,mid+1,r,x,y); } }}Tree[25];int main(){ //freopen("in.in","r",stdin); while(~scanf("%d%d%d",&R,&C,&M)){ for(int i=1;i<=R;i++){ Tree[i].build(1,1,C); } while(M--){ int x1,y1,x2,y2,vv; scanf("%d %d%d%d%d", &q,&x1,&y1,&x2,&y2); if(q==3){ sum=0;minn=inf;maxn=-inf; for(int i=x1;i<=x2;i++){ Tree[i].query(1,1,C,y1,y2); } printf("%d %d %d\n",sum,minn,maxn); } else{ scanf("%d",&vv); for(int i=x1;i<=x2;i++){ Tree[i].update(1,1,C,y1,y2,vv); } } } } return 0;}
- UVA 11992- Fast Matrix Operations(线段树区间各种操作)
- UVA 11992 Fast Matrix Operations (线段树区间更新)
- uva 11992 Fast Matrix Operations (线段树区间更新)
- uva 11992 Fast Matrix Operations(线段树,区间修改)
- UVA - 11992 Fast Matrix Operations 线段树(区间修改)
- Uva 11992 Fast Matrix Operations(线段树区间设值与加操作)
- uva 1401 Fast Matrix Operations 快速矩阵操作 (线段树 区间修改和查询)
- UVa 11992 Fast Matrix Operations (线段树区间修改大杂烩)
- uva 11992 Fast Matrix Operations(线段树)
- UVa 11992 Fast Matrix Operations 线段树
- UVA 11992 - Fast Matrix Operations(线段树)
- uva 11992 Fast Matrix Operations 线段树
- uva 11992 - Fast Matrix Operations(线段树)
- UVA - 11992 Fast Matrix Operations(线段树)
- UVA 11992 Fast Matrix Operations 线段树
- Fast Matrix Operations uva+线段树区间修改
- 【UVA】11992 - Fast Matrix Operations(线段树模板)
- Uva 11992 Fast Matrix Operations (二维线段树)
- iOS_第3方类库SDWebImage
- 觉得覅哦
- 从awr中获取scn变化趋势的另外一个脚本--来自www.oracledatabase12g.com
- 移动客户端与服务器端安全通信方案
- segue 分析小结
- UVA 11992- Fast Matrix Operations(线段树区间各种操作)
- 设计模式六大原则
- PHP中require和include的区别
- 【漫立方译】交互式GPU光线追踪——用于基于物理的灯光预览
- 【漫立方译】交互式GPU光线追踪——用于基于物理的灯光预览
- Java的类载入器
- 【漫立方译】交互式GPU光线追踪——用于基于物理的灯光预览
- 发外链
- KMP模板