Intersection
来源:互联网 发布:卤素灯 led 对比 知乎 编辑:程序博客网 时间:2024/04/27 14:34
Description
An example:
line: start point: (4,9)
end point: (11,2)
rectangle: left-top: (1,5)
right-bottom: (7,1)
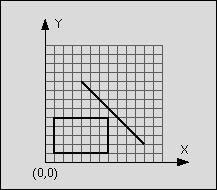
Figure 1: Line segment does not intersect rectangle
The line is said to intersect the rectangle if the line and the rectangle have at least one point in common. The rectangle consists of four straight lines and the area in between. Although all input values are integer numbers, valid intersection points do not have to lay on the integer grid.
Input
xstart ystart xend yend xleft ytop xright ybottom
where (xstart, ystart) is the start and (xend, yend) the end point of the line and (xleft, ytop) the top left and (xright, ybottom) the bottom right corner of the rectangle. The eight numbers are separated by a blank. The terms top left and bottom right do not imply any ordering of coordinates.
Output
Sample Input
14 9 11 2 1 5 7 1
Sample Output
F
Source
做这个题都快哭了,明明一个简单的题花了我一下午外加一晚上,最后错的原因是矩形时左上角坐标和右下角坐标并不确定。另外线段在矩形内部是也输出T,因为矩形是实心的,可以想到的错我全犯了,人才啊,下午的比赛啥也没干全弄它了。
其实看矩形的四条边和已知线段是否相交,和线段是否在矩形内部,就可以确定答案的输出。所以代码如下:
#include<stdio.h>
#include<string.h>
#include<string>
#include <math.h>
#define eps 1e-8
#define zero(x) (((x)>0?(x):-(x))<eps)
struct point
{
double x,y;
};
double xmult(point p1,point p2,point p0)
{
return (p1.x-p0.x)*(p2.y-p0.y)-(p2.x-p0.x)*(p1.y-p0.y);
}
double dmult(point p1,point p2,point p0)
{
return (p1.x-p0.x)*(p2.x-p0.x)+(p1.y-p0.y)*(p2.y-p0.y);
}
int dots_inline(point p1,point p2,point p3)
{
return zero(xmult(p1,p2,p3));
}
int dot_online_in(point p,point l1,point l2)
{
return zero(xmult(p,l1,l2))&&(l1.x-p.x)*(l2.x-p.x)<eps&&(l1.y-p.y)*(l2.y-p.y)<eps;
}
int same_side(point p1,point p2,point l1,point l2)
{
return xmult(l1,p1,l2)*xmult(l1,p2,l2)>eps;
}
int intersect_in(point u1,point u2,point v1,point v2)
{
if (!dots_inline(u1,u2,v1)||!dots_inline(u1,u2,v2))
return !same_side(u1,u2,v1,v2)&&!same_side(v1,v2,u1,u2);
return dot_online_in(u1,v1,v2)||dot_online_in(u2,v1,v2)||dot_online_in(v1,u1,u2)||dot_online_in(v2,u1,u2);
}
int main()
{
point x,y;
point q[10],p,pp;
int T,i,j,k;
scanf("%d",&T);
while(T--)
{
scanf("%lf%lf%lf%lf%lf%lf%lf%lf",&p.x,&p.y,&pp.x,&pp.y,&q[4].x,&q[4].y,&q[2].x,&q[2].y);
q[1].x=q[4].x;
q[1].y=q[2].y;
q[3].x=q[2].x;
q[3].y=q[4].y;
if(((p.x>=q[2].x&&p.x<=q[4].x)||(p.x>=q[4].x&&p.x<=q[2].x)) && ((p.y>=q[2].y&&p.y<=q[4].y)||(p.y>=q[4].y&&p.y<=q[2].y)) )
{
printf("T\n");
continue;
}
if(((pp.x>=q[2].x&&pp.x<=q[4].x)||(pp.x>=q[4].x&&pp.x<=q[2].x)) && ((pp.y>=q[2].y&&pp.y<=q[4].y)||(pp.y>=q[4].y&&pp.y<=q[2].y)))
{
printf("T\n");
continue;
}
if(intersect_in(p,pp,q[1],q[2])||intersect_in(p,pp,q[3],q[2])||intersect_in(p,pp,q[3],q[4])||intersect_in(p,pp,q[1],q[4]))
{
printf("T\n");
}
else
{
printf("F\n");
}
}
return 0;
}
- Intersection
- Intersection
- Intersection
- Intersection
- Intersection graph & Intersection number
- poj1410 - Intersection
- 18:Intersection
- uva191 Intersection
- POJ1410 Intersection
- poj1410 Intersection
- 1879: Intersection
- uva191 Intersection
- poj1410 Intersection
- intersection set
- poj1410 Intersection
- ZOJ3862:Intersection
- HDU_5120 Intersection
- 2.7 Intersection
- 【积性函数】
- POJ 1330 Nearest Common Ancestors
- 漫谈计算机视觉
- hdu 4778 Gems Fight!(状态压缩dp)
- 黑白棋子的移动
- Intersection
- 解析String类和StringBuilder
- Eclipse 用DDMS 调试Android 程序
- ActiveX控件的安全初始化和脚本操作 和 数字签名SIGN
- Oracle优化器和优化模式
- 笔记
- Android-Socket传输 GPRS网络
- linux系统监控——nmon介绍与使用
- 小鑫的城堡(并查集)