Programmatically Injecting Events on Android – Part 2
来源:互联网 发布:网络the one什么意思 编辑:程序博客网 时间:2024/05/11 02:35
While this seems unimportant to the regular user, this is fundamentally crucial for developing "also regular" applications such as: remote administration (like VNC, teamviewer, remote control), for application test tools, or for applications aiming to improve the smartphone control for persons with disabilities (using the chair joystick to control the Android phone). Another example here, is the option to create a custom mouse pointer on top of your Android screen, for application control purposes, as presented here, that also needs to be able to inject the touch events when the user clicks.
Not to mention various automation tasks, hacks & mods but in the end is all about the freedom given to the development community. The Android platform makers should come up with some better solutions.
Introduction
In part 1 of this article I have indicated three ways of injecting events on the Android platform:
Method 1: Using internal APIs, mainly relying on the WindowManager and the two APIs: injectPointerEvent and injectKeyEvent
Method 2: Using an instrumentation object
Method 3: Direct event injection to /dev/input/eventX
While the first two rely on the dreaded INJECT_EVENTS permission, available only to platform makers, and have suffered great changes in the last OS versions, the third method is at the moment of writing this article the best bet for properly injecting events on Android.
The /dev/input/eventX are event input nodes, part of the linux platform beneath Android. Normally their permissions are set so only the Owner and its Group can read and write from/top these files. There are no permissions set for Other, and there is where our code is situated, since it is not owner of the linux input event nodes, nor member of the Owner group:
So to be able to use method 3, and to directly interface to the input event nodes, we need to be able to alter the permissions, in order to set the Other permission bit to Read (if we want to intercept input event data) or Write (if we want to push events, as indicated in the article's title: Injecting events). The best way to go is to set the bit to allow +rw (read and write) , using the chmod native command:
Without root access, this fails, with "operation not permitted". So here is the point where we can only proceed if we are using a Rooted Android phone. Everything else shown in this article will work without root, but for changing the permissions we need root, and there's no way around it. A bit frustrating, considering we are talking about such a tiny information segment. More on linux filesystem permissions, here.
Once the input event nodes are set to allow both reading and writing, we can open them as regular files, and read the content or write our own content, to intercept events or inject our own keys or touch events. The format accepted by the input event node files is:
struct input_event { struct timeval time; unsigned short type; unsigned short code; unsigned int value;};/* type and code are values defined in linux/input.h. * For example, type might be EV_REL for relative moment of a mouse, * or EV_KEY for a keypress, and code is the keycode, or REL_X or ABS_X for a mouse. * The linux/input.h is part of the Android NDK */
Make sure to read more on this topic, for a better understanding. A nice article is available on Linux Journal.
By popular demand on my two previous articles: Programmatically Injecting Events on Android – Part 1 and Android Overlay Mouse Cursor, but also to standardize method #3, I have developed a JNI library for Android, to take care of all these details to do what the standard API refuses to do: simple event injection for all your development needs. But it requires root, as explained previously.
The library, named "android-event-injector" is released as Open Source, under GPL, and available on Google code, here.
Android Event Injector functionality
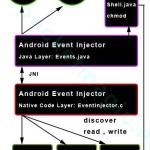
It uses a low level, native component, that completely handles the input event node interaction: discovering the files (ScanFiles), opening them (OpenDev), Closing/Removing allocated memory (RemoveDev) or getting event node details such as Path and Name. With an open Input Event Node, we can do polling (PollDev, getType, getCode, getValue) or event injection (intSendEvent).
Via JNI, we are handling all this functionality in Java (Events.Java). This class builds multiple instances of the InputDevice class, that correspond to the number of discovered native input event nodes (the number of /dev/input/eventX files). When we open such a file, we use the API call Open(boolean forceOpen):
/*** function Open : opens an input event node* @param forceOpen will try to set permissions and then reopen if first open attempt fails* @return true if input event node has been opened*/public boolean Open(boolean forceOpen) { int res = OpenDev(m_nId); // if opening fails, we might not have the correct permissions, try changing 660 to 666 if (res != 0) { // possible only if we have root if(forceOpen && Shell.isSuAvailable()) { // set new permissions Shell.runCommand("chmod 666 "+ m_szPath); // reopen res = OpenDev(m_nId); } } m_szName = getDevName(m_nId); m_bOpen = (res == 0); // debug Log.d(LT, "Open:"+m_szPath+" Name:"+m_szName+" Result:"+m_bOpen); // done, return return m_bOpen;}
As you can see, at this point we also use chmod to make sure our process can read and write the input event node (/dev/input/eventX file).
If we are successful in opening an event node, we can use it for listening for events (system touch events, key presses, etc), or for injecting events. The code on Google code, exemplifies all these:
Listening for incoming events
Here is a simple example on how to use my library, to create a thread that intercepts all system input events and shows them in logcat:
/*** Starts our event monitor thread that does the data extraction via polling* all data is displayed in the textview, as type-code-value, see input.h in the Android NDK for more details* Monitor output is also sent to Logcat, so make sure you used that as well*/public void StartEventMonitor() {m_bMonitorOn = true;Thread b = new Thread(new Runnable() { public void run() { while (m_bMonitorOn) { for (InputDevice idev:events.m_Devs) { // Open more devices to see their messages if (idev.getOpen() && (0 == idev.getPollingEvent())) { final String line = idev.getName()+ ":" + idev.getSuccessfulPollingType()+ " " + idev.getSuccessfulPollingCode() + " " + idev.getSuccessfulPollingValue(); Log.d(LT, "Event:"+line); // update textview to show data //if (idev.getSuccessfulPollingValue() != 0) m_tvMonitor.post(new Runnable() { public void run() { m_tvMonitor.setText(line); } }); } } } }});b.start(); }
This is very useful when you need to better understand the event structure and the involved values for type,code and value. Make sure you have a look on linux/input.h contents as well, inside your Android NDK folder.
Injecting events
Again, input.h is our friend, since it details the event codes we need to use. But you will also want to use the event monitor to be able to write down all the complex events sequence generated by the system when the user touches the screen. Here are a few wrapper functions to serve as a sample:
Home hardware key injection:
/*** Finds an open device that has a name containing keypad. This probably is the event node associated with the keypad* Its purpose is to handle all hardware Android buttons such as Back, Home, Volume, etc* Key codes are defined in input.h (see NDK) , or use the Event Monitor to see keypad messages* This function sends the HOME key */public void SendHomeKeyToKeypad() {boolean found = false;for (InputDevice idev:events.m_Devs) { //* Finds an open device that has a name containing keypad. This probably is the keypad associated event node if (idev.getOpen() && idev.getName().contains("keypad")) { idev.SendKey(102, true); // home key down idev.SendKey(102, false); // home key up found = true; break; }}if (found == false) Toast.makeText(this, "Keypad not found.", Toast.LENGTH_SHORT).show();}
And another example, for injecting touch events:
//absolute coordinates, on my device they go up to 570x960if (m_selectedDev!=-1) events.m_Devs.get(m_selectedDev).SendTouchDownAbs(155,183);
Don't forget to correctly use the Event input devices. When you open them, you also have access to the Device Name. Make sure you insert key events to the device named "keypad" or "keyboard" and not to the compass or other input devices if you want this to work. Also for touch events, make sure you insert your touches/click events to "touchpad" or "ts". The namings can be different from a device to another, or from a platform to another. Try to develop an algorithm to correctly identify your target before injecting input data.
Additional resources:
Programmatically Injecting Events on Android – Part 1
Android Overlay Mouse Cursor
The code is available on Google Code, under GPL. Use it only if you understand the terms of Open Source software distributed under GPL.
The library and a sample can be downloaded here or EventInjector
From: http://www.pocketmagic.net/2013/01/programmatically-injecting-events-on-android-part-2
参考:https://code.google.com/p/android-event-injector/
- Programmatically Injecting Events on Android – Part 2
- Programmatically Injecting Events on Android – Part 1
- Working with Events, part 2
- how to install CA certificate programmatically on Android without user interaction
- Caliburn Micro Part 2: Data Binding and Events
- Margin set programmatically on RadioButton not applied
- Zipping Files with Android (Programmatically)
- Working with Events, part 1
- Oracle BPM: Working with Tasks Programmatically (Part II)
- OpenGL ES Tutorial for Android – Part V – More on Meshes
- OpenGL ES Tutorial for Android – Part V – More on Meshes
- Tutorial on Binary Descriptors – part 1
- WebKit on the iPhone (Part 2)
- The Future of Comet: Part 2, HTML 5’s Server-Sent Events
- Port Windows IPC apps to Linux, Part 2: Semaphores and events
- Port Windows IPC apps to Linux, Part 2: Semaphores and events
- Android逆向工程101 – Part 2
- How-to create a Calculator on Google Android: Part I
- 全志A10tablet1.*反复重启加载用户可用Flash(SD卡)上的程序几率性失败 .
- Openstack和XenServer——基础架构
- 黑马程序员-ArrayList、HashSet比较和HashCode分析
- Raid信息保存位置
- ZOJ-1455 Schedule Problem
- Programmatically Injecting Events on Android – Part 2
- 计算机网络面试题总结
- 字符串处理函数
- arm linux无线路由
- 解决ubuntu下修改my.cnf设置字符集导致mysql无法启动
- 数据库进阶系列之二:细说数据库范式
- epplus概要说明
- Centos6.5系统初学者基本系统配置1
- 去掉最大值、最小值之后剩下的个数(华为上机试题8_29_1)