boost库中bind()和function()的使用方法
来源:互联网 发布:sql基本语句大全 编辑:程序博客网 时间:2024/05/22 05:15
Boost::Function 是对函数指针的对象化封装,在概念上与广义上的回调函数类似。相对于函数指针,function除了使用自由函数,还可以使用函数对象,甚至是类的成员函数,这个就很强大了哈。1. 一个简单的示例代码
输出结果:
输出结果(Cygwin):
>根据上述程序,我们可以发现,这样我们就不需要定义很多很多的类去继承Thread类,而只需要写好线程运行函数,set到Thread类中即可。不过也不能说利用虚函数留接口给用户实现就不好,只不过现在多了一种方法。(陈硕很反对用虚函数作为结构提供给用户去做实现,但是我现在还没有切身的体会觉得那里不好)
1. 一个简单的示例代码
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
#include <boost/function.hpp>
#include <boost/bind.hpp>
#include <iostream>
using
namespace
std;
class
TestA
{
public
:
void
method()
{
cout<<
"TestA: method: no arguments"
<<endl;
}
void
method(
int
a,
int
b)
{
cout<<
"TestA: method: with arguments"
<<
"value of a is:"
<<a
<<
"value of b is "
<<b <<endl;
}
};
void
sum(
int
a,
int
b)
{
int
sum = a + b;
cout<<
"sum: "
<<sum<<endl;
}
int
main()
{
boost::function<
void
()> f;
TestA test;
f = boost::bind(&TestA::method, &test);
f();
f = boost::bind(&TestA::method, &test, 1, 2);
f();
f = boost::bind(&sum, 1, 2);
f();
}
1
2
3
4
5
Administrator@8bd5ec9e02074bf ~/source
$ ./BoostFunction.exe
TestA: method: no arguments
TestA: method: with argumentsvalue of a is:1value of b is 2
sum: 3
2. 应用:Thread封装
在实现自定义的线程类时,曾经这么干过:定义虚函数run(),用户自定义的CustomThread::Thread后,自己实现run()函数就OK了。 当时觉得这么做也不错。
现在有了boost::function/boost::bind我们可以这么干:
定义一个线程类:
.h文件
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
#include <pthread.h>
#include <string>
#include <boost/function.hpp>
#include <boost/bind.hpp>
using
namespace
std;
class
Thread
{
typedef
boost::function<
void
()> ThreadFun;
public
:
Thread(
const
ThreadFun& threadFun,
const
string& threadName = string());
pid_t getThreadId();
string getThreadName();
int
start();
private
:
static
void
* startThread(
void
*
thread
);
private
:
pthread_t m_thread;
//线程句柄
pid_t m_tid;
//线程ID
string m_strThreadName;
//线程名称
bool
m_bStarted;
//线程是否启动
ThreadFun m_func;
//线程处理函数
};
.cpp文件
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
#include "thread.h"
Thread::Thread(
const
Thread::ThreadFun& threadFun,
const
string& threadName):
m_func(threadFun), m_strThreadName(threadName)
{
}
int
Thread::start()
{
m_tid = pthread_create(&m_thread, NULL, &startThread,
this
);
return
0;
}
void
* Thread::startThread(
void
* obj)
{
Thread*
thread
=
static_cast
<Thread*>(obj);
thread
->m_func();
return
NULL;
}
pid_t Thread::getThreadId()
{
return
m_tid;
};
string Thread::getThreadName()
{
return
m_strThreadName;
}
测试程序
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
void
ThreadProcess()
{
int
count = 100;
for
(
int
i = 0; i < count; i++)
{
if
(i % 10 == 0)
cout<<
"\n"
;
cout<<i<<
"\t"
;
}
}
int
main()
{
boost::function<
void
()> f;
f = boost::bind(&ThreadProcess);
Thread
thread
(f,
"ThreadTest"
);
thread
.start();
sleep(1000*1000);
return
0;
}
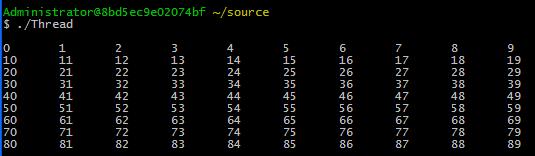
3. 总结
注:
1. 这边只是简单的用boost::function/bind结合pthread简单的实现了一个自己封装的线程类,
自己以前在windows下实现的基于虚函数的线程类实现:http://my.oschina.net/myspaceNUAA/blog/41014
2. boost::function/bind还有很多其他高级用法,我这边只是用来当做一个函数指针用了哈
3. 测试环境:cygwin
4.参考文献:
http://blog.csdn.net/benny5609/article/details/2324474
http://blog.csdn.net/Solstice/article/details/3066268
http://blog.csdn.net/benny5609/article/details/2324474
http://blog.csdn.net/Solstice/article/details/3066268
0 0
- boost库中bind()和function()的使用方法
- boost的库中bind和function使用实例
- 【Boost】boost库中function和bind一起使用的技巧(一)
- 【Boost】boost库中function和bind一起使用的技巧(二)
- C++中std::tr1::function和bind 组件的使用 和 以boost::function和boost:bind取代虚函数
- boost::function和boost::bind
- boost::function 和boost::bind
- boost::bind的使用方法
- boost::bind的使用方法
- boost::bind的使用方法
- boost::bind的使用方法
- boost::bind的使用方法
- boost::bind的使用方法
- boost::bind的使用方法
- boost::bind的使用方法
- boost bind的使用方法
- boost::bind的使用方法
- boost库 bind/function的使用
- 图像物体分类和物体检测算法的概括
- grub rescue
- NSRunloop的解析及autorelease的释放时机
- JAVA程序设计(14.2)----- 图书馆管理系统 初步设计 界面篇
- XMPP服务器环境的搭建
- boost库中bind()和function()的使用方法
- Oracle数据库mybatis 插入空值时报错(with JdbcType OTHER)
- tomcat配置HTTPS
- php用户登录以及后台处理
- HTML 入门,30分钟入门教程.
- 数据库集群实施
- csu1030: 素数槽
- iOS Developer:真机测试
- 第三章 资源管理