POJ 3239 Solution to the n Queens Puzzle
来源:互联网 发布:最大公约数的算法 编辑:程序博客网 时间:2024/05/29 17:50
Solution to the n Queens Puzzle
Time Limit: 1000MS Memory Limit: 131072KTotal Submissions: 3469 Accepted: 1275 Special Judge
Description
The eight queens puzzle is the problem of putting eight chess queens on an 8 × 8 chessboard such that none of them is able to capture any other. The puzzle has been generalized to arbitrary n × n boards. Given n, you are to find a solution to the n queens puzzle.
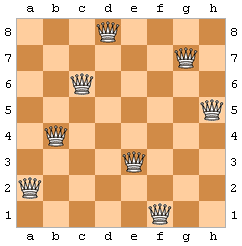
Input
The input contains multiple test cases. Each test case consists of a single integer n between 8 and 300 (inclusive). A zero indicates the end of input.
Output
For each test case, output your solution on one line. The solution is a permutation of {1, 2, …, n}. The number in the ith place means the ith-column queen in placed in the row with that number.
Sample Input
80
Sample Output
5 3 1 6 8 2 4 7
Source
POJ Monthly--2007.06.03, Yao, Jinyu
N皇后问题,主要是为了测试代码:
#include <iostream>#include <vector>using namespace std;class Queen {public: char QChar, BChar; // 代表皇后和空白的字符 int * q_in_row; // q_in_row[i] = j表示在第i行第j列有个皇后 bool * col_free; // col_free[i] = true表示第i列没有皇后 bool * up_free; // 从右上到左下的对角线是否可以放置皇后 bool * down_free; // 从左上到右下的对角线是否可以放置皇后 int N; // 皇后个数、正方形棋盘的边长 bool ONE, NUM, ALL; // 由于只有一个递归函数,三个变量用于区分是哪一个问题 bool ONE_FINISH; // 判断只返回一个解的问题是否已经解决 int num; // 所有解的个数 vector<vector<char> > oneAns; // 一个解 vector<vector<vector<char> > > allAns; // 所有解 Queen() { QChar = 'Q'; BChar = '.'; ONE = NUM = ALL = ONE_FINISH = false; } void changeChar(char q, char b) { // 可以改变代表皇后和空白的字符 QChar = q; BChar = b; } void makeBoard(int n) { N = n; q_in_row = new int[N]; col_free = new bool[N]; up_free = new bool[2 * N - 1]; down_free = new bool[2 * N - 1]; for (int i = 0; i < N; i++) q_in_row[i] = col_free[i] = true; for (int i = 0; i < 2 * N - 1; i++) up_free[i] = down_free[i] = true; } void deleteBoard() { delete []q_in_row; delete []col_free; delete []up_free; delete []down_free; } void construct_solution() { int row = 0; if (N % 6 != 2 && N % 6 != 3) { if (N % 2 == 0) { for (int i = 2; i <= N; i += 2) q_in_row[row++] = i - 1; for (int i = 1; i <= N - 1; i += 2) q_in_row[row++] = i - 1; } else { for (int i = 2; i <= N - 1; i += 2) q_in_row[row++] = i - 1; for (int i = 1; i <= N; i += 2) q_in_row[row++] = i - 1; } } else { if (N % 2 == 0) { if ((N / 2) % 2 == 0) { for (int i = N / 2; i <= N; i += 2) q_in_row[row++] = i - 1; for (int i = 2; i <= N / 2 - 2; i += 2) q_in_row[row++] = i - 1; for (int i = N / 2 + 3; i <= N - 1; i += 2) q_in_row[row++] = i - 1; for (int i = 1; i <= N / 2 + 1; i += 2) q_in_row[row++] = i - 1; } else { for (int i = N / 2; i <= N - 1; i += 2) q_in_row[row++] = i - 1; for (int i = 1; i <= N / 2 - 2; i += 2) q_in_row[row++] = i - 1; for (int i = N / 2 + 3; i <= N; i += 2) q_in_row[row++] = i - 1; for (int i = 2; i <= N / 2 + 1; i += 2) q_in_row[row++] = i - 1; } } else { if ((N / 2) % 2 == 0) { for (int i = N / 2; i <= N - 1; i += 2) q_in_row[row++] = i - 1; for (int i = 2; i <= N / 2 - 2; i += 2) q_in_row[row++] = i - 1; for (int i = N / 2 + 3; i <= N - 2; i += 2) q_in_row[row++] = i - 1; for (int i = 1; i <= N / 2 + 1; i += 2) q_in_row[row++] = i - 1; q_in_row[row++] = N - 1; } else { for (int i = N / 2; i <= N - 2; i += 2) q_in_row[row++] = i - 1; for (int i = 1; i <= N / 2 - 2; i += 2) q_in_row[row++] = i - 1; for (int i = N / 2 + 3; i <= N - 1; i += 2) q_in_row[row++] = i - 1; for (int i = 2; i <= N / 2 + 1; i += 2) q_in_row[row++] = i - 1; q_in_row[row++] = N - 1; } } } write_in(oneAns); } vector<vector<char> > Return_an_ans(int n) { makeBoard(n); if (n > 3) { construct_solution(); deleteBoard(); return oneAns; } ONE = true; dfs(0); deleteBoard(); return oneAns; } int Return_all_ans_num(int n) { makeBoard(n); NUM = true; num = 0; dfs(0); deleteBoard(); return num; } vector<vector<vector<char> > > Return_all_ans(int n) { makeBoard(n); ALL = true; dfs(0); deleteBoard(); return allAns; } void write_in(vector<vector<char> > & a) { a.resize(N); for (int i = 0; i < N; i++) { a[i].resize(N); for (int j = 0; j < N; j++) { if (q_in_row[i] == j) a[i][j] = QChar; else a[i][j] = BChar; } } } void dfs(int now) { if (now == N) { if (ONE) { ONE_FINISH = true; write_in(oneAns); return; } if (NUM) { num++; return; } if (ALL) { vector<vector<char> > a; write_in(a); allAns.push_back(a); return; } } for (int col = 0; col < N; col++) { if (col_free[col] && up_free[now + col] && down_free[now - col + N - 1]) { q_in_row[now] = col; col_free[col] = up_free[now + col] = down_free[now - col + N - 1] = false; dfs(now + 1); if (ONE && ONE_FINISH) return; col_free[col] = up_free[now + col] = down_free[now - col + N - 1] = true; } } }};int main() { std::cout.sync_with_stdio(false); while (1) { Queen q; int n; cin >> n; if (n == 0) break; vector<vector<char> > ans = q.Return_an_ans(n); for (int i = 0; i < ans.size(); i++) { for (int j = 0; j < ans[i].size(); j++) { if (ans[i][j] == 'Q') { if (i) cout << " "; cout << j + 1; } } } cout << endl; } return 0;}
0 0
- POJ 3239 Solution to the n Queens Puzzle
- Poj 3239 Solution to the n Queens Puzzle
- POJ 3239 Solution to the n Queens Puzzle
- poj 3239 Solution to the n Queens Puzzle n皇后问题的构造解法
- PKU3239 Solution to the n Queens Puzzle
- POJ3239 Solution to the n Queens Puzzle
- poj3239 Solution to the n Queens Puzzle (n皇后问题)
- BZOJ_P3101 N皇后/POJ_P3239 Solution to the n Queens Puzzle(N皇后构造)
- POJ3239《Solution to the n Queens Puzzle》方法:构造公式法
- n-queens puzzle
- POJ 3240 Solution to the n2 − 1 Puzzle 英文少
- 1128. N Queens Puzzle 解析
- 1128. N Queens Puzzle (20)
- PAT--1128. N Queens Puzzle
- pat 1128N Queens Puzzle
- 1128. N Queens Puzzle (20)
- 1128. N Queens Puzzle (20)
- 1128. N Queens Puzzle (20)
- Sicily 1151. 魔板
- RDLC报表刷新问题
- Sicily 1004. I Conduit!
- hibernate4基础梳理(一)---一个问题忙一天
- 了解OS
- POJ 3239 Solution to the n Queens Puzzle
- 【WIN32之旅】WINDOWS错误处理与参考(一)
- poj 1338 Ugly Numbers
- 通过office 自带功能进行批量另存为
- N-Queen
- C# TextBox中只允许输入数字的方法
- vim note(6)--vim的一个较全的介绍(转)
- LeetCode OJ Regular Expression Matching
- Nginx的Web缓存服务