poj1753高斯消元法和位运算枚举法。
来源:互联网 发布:oppo状态栏美化软件 编辑:程序博客网 时间:2024/06/03 16:34
Flip Game
Time Limit: 1000MS Memory Limit: 65536KTotal Submissions: 32829 Accepted: 14348
Description
Flip game is played on a rectangular 4x4 field with two-sided pieces placed on each of its 16 squares. One side of each piece is white and the other one is black and each piece is lying either it's black or white side up. Each round you flip 3 to 5 pieces, thus changing the color of their upper side from black to white and vice versa. The pieces to be flipped are chosen every round according to the following rules:
Consider the following position as an example:
bwbw
wwww
bbwb
bwwb
Here "b" denotes pieces lying their black side up and "w" denotes pieces lying their white side up. If we choose to flip the 1st piece from the 3rd row (this choice is shown at the picture), then the field will become:
bwbw
bwww
wwwb
wwwb
The goal of the game is to flip either all pieces white side up or all pieces black side up. You are to write a program that will search for the minimum number of rounds needed to achieve this goal.
- Choose any one of the 16 pieces.
- Flip the chosen piece and also all adjacent pieces to the left, to the right, to the top, and to the bottom of the chosen piece (if there are any).
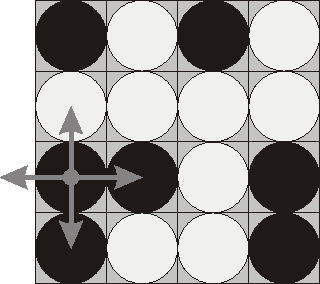
bwbw
wwww
bbwb
bwwb
Here "b" denotes pieces lying their black side up and "w" denotes pieces lying their white side up. If we choose to flip the 1st piece from the 3rd row (this choice is shown at the picture), then the field will become:
bwbw
bwww
wwwb
wwwb
The goal of the game is to flip either all pieces white side up or all pieces black side up. You are to write a program that will search for the minimum number of rounds needed to achieve this goal.
Input
The input consists of 4 lines with 4 characters "w" or "b" each that denote game field position.
Output
Write to the output file a single integer number - the minimum number of rounds needed to achieve the goal of the game from the given position. If the goal is initially achieved, then write 0. If it's impossible to achieve the goal, then write the word "Impossible" (without quotes).
Sample Input
bwwbbbwbbwwbbwww
Sample Output
4
题意很简单,给你一个4*4的黑白棋盘初始状态,问你最少翻转多少次使得棋子都是白色或者黑色。翻转一个棋子,其相邻四周的棋子同时翻转。
方法一就是位运算枚举1<<16次方的复杂度,为1就代表翻转当前位,然后变换四周及他的棋子状态,然后看最后是否满足同色。
方法二就是高斯消元列出方程组。利用每个位置的状态判断翻转后的状态,然后求出自由变元的个数,由于有几个自由变元就代表这几个棋子的位置是可以任意选择翻转或者不翻转的,其他位置棋子相应产生影响,结果也是可以满足条件,所以还要枚举这几个自由变元,然后求得翻转次数最小的。
注意这里黑白两个同色都可以,我就增加了一列var+1,和var一样,分别存放最后全白,最后全黑的情况。就这样了。可以当做模板刷一刷。
#include<limits>#include<queue>#include<vector>#include<list>#include<map>#include<set>#include<deque>#include<stack>#include<bitset>#include<algorithm>#include<functional>#include<numeric>#include<utility>#include<sstream>#include<iostream>#include<iomanip>#include<cstdio>#include<cmath>#include<cstdlib>#include<cstring>#include<ctime>#define LL long long#define eps 1e-8#define pi acos(-1)#define INF 0x7fffffff#define delta 0.98 //模拟退火递增变量using namespace std;int x[30],a[30][30];int freex[30],freexnum;int equ,var;int solve(int t){int ans=0x3fffffff;int i,j;for (i=0;i<(1<<t);i++){int cnt=0;memset(x,0,sizeof(x));for (j=0;j<t;j++)if (i & (1<<j)){cnt++;x[freex[j]]=1;}else x[freex[j]]=0;int dx;for (j=var-t-1;j>=0;j--){for (dx=j;dx<var;dx++)if (a[j][dx]) break;x[dx]=a[j][var];for (int l=dx+1;l<var;l++)if (a[j][l])x[dx]^=x[l];cnt+=x[dx];}ans=min(ans,cnt); } for (i=0;i<(1<<t);i++){int cnt=0;memset(x,0,sizeof(x));for (j=0;j<t;j++)if (i & (1<<j)){cnt++;x[freex[j]]=1;}else x[freex[j]]=0;int dx;for (j=var-t-1;j>=0;j--){for (dx=j;dx<var;dx++)if (a[j][dx]) break;x[dx]=a[j][var+1];for (int l=dx+1;l<var;l++)if (a[j][l])x[dx]^=x[l];cnt+=x[dx];}ans=min(ans,cnt); }return ans;}int Gauss(){int r,l,i,j;freexnum=0;for (r=0,l=0;r<equ && l<var;r++,l++){int mr=r;if (a[mr][l]==0)for (i=r+1;i<equ;i++)if (a[i][l]){mr=i;break;}if (a[mr][l]==0){r--;freex[freexnum++]=l;continue;}if (mr!=r)for (i=l;i<=var+1;i++)swap(a[mr][i],a[r][i]);for (i=r+1;i<equ;i++)if (a[i][l])for (j=l;j<=var+1;j++)a[i][j]^=a[r][j]; }for (i=r+1;i<equ;i++)if (a[i][var]!=0 && a[i][var+1]!=0) return -1;return solve(var-r); }int main(){equ=0;var=16;int i,j;for(i=0;i<4;i++){for (j=0;j<4;j++){char k;scanf("%c",&k);if (i>0) a[equ][(i-1)*4+j]=1;if (i<3) a[equ][(i+1)*4+j]=1;if (j>0) a[equ][i*4+j-1]=1;if (j<3) a[equ][i*4+j+1]=1;a[equ][i*4+j]=1;if (k=='b'){a[equ][var]=1;a[equ][var+1]=0;}else{a[equ][var]=0;a[equ][var+1]=1; }equ++;}getchar();}int f=Gauss();if (f==-1) cout<<"Impossible"<<endl;else cout<<f<<endl;return 0;}
#include <iostream>using namespace std;int a[20][20];int b[20][20];void update(int x){int r=x/4;int l=x%4;b[r][l]^=1;if (r-1>=0) b[r-1][l]^=1;if (r+1<4) b[r+1][l]^=1;if (l-1>=0) b[r][l-1]^=1;if (l+1<4) b[r][l+1]^=1;}int go(){int i,j;for (i=0;i<4;i++)for (j=0;j<4;j++)if (b[i][j]!=b[0][0]) return 0;return 1;}int main(){int i,j;for (i=0;i<4;i++){for (j=0;j<4;j++){char k;scanf("%c",&k);if (k=='b') a[i][j]=0;else a[i][j]=1;}getchar();}int ans=20;for (i=0;i<(1<<16);i++){int t=0;for (int i1=0;i1<4;i1++)for (int i2=0;i2<4;i2++)b[i1][i2]=a[i1][i2];for (j=0;j<16;j++){if (i & (1<<j)){t++;update(j);}}if (ans>t && go()) {ans=t;}}if (ans==20) cout<<"Impossible"<<endl;else cout<<ans<<endl;return 0;}
0 0
- poj1753高斯消元法和位运算枚举法。
- POJ1753(枚举+位运算)
- POJ1753 -- BFS和位运算
- poj1753 Flip Game 位运算+科学枚举法 0ms无压力~
- poj1753 Flip Game dfs 枚举 待补完 位运算
- POJ1753 Flip Game(搜索,枚举,位运算压缩)
- POJ1753:Flip Game(BFS、枚举、位运算)
- poj1753(暴搜+位运算)
- poj1753--flipgame---位运算 + bfs
- poj1753 广搜+位运算
- poj1753 搜索,枚举法
- poj1753/poj2965 枚举法
- iOS:枚举和位运算
- poj1753(位运算(异或)+ bfs)
- poj1753 Flip Game BFS+位运算
- #POJ1753#Flip Game(位运算+BFS)
- POJ1753 Flip Game 位运算+搜索
- poj1753枚举
- Hadoop2.4.1集群搭建
- ios-day18-11(使用CAAnimationGroup实现对UIView的组合动画)
- final 关键字 限定方法参数
- HDOJ 1058 - Humble Numbers 更新Treap模板..
- 操作标签
- poj1753高斯消元法和位运算枚举法。
- C++ application fails to start correctly (0xc000000d)
- 使用GitHub
- Lucene 实战:快速开始 创建索引
- leetcode 日经贴,Cpp code -Rotate Image
- static成员函数
- poj2159 Ancient Cipher
- 自定义Git
- 源码阅读(一)RadialTransitionExample