[ACM] hdu 1035 Robot Motion (模拟或DFS)
来源:互联网 发布:齐鲁石化网络电视台 编辑:程序博客网 时间:2024/05/01 06:13
Robot Motion
Problem Description
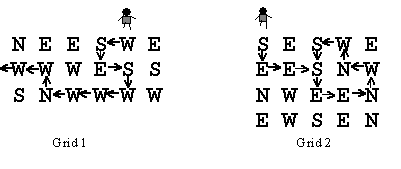
A robot has been programmed to follow the instructions in its path. Instructions for the next direction the robot is to move are laid down in a grid. The possible instructions are
N north (up the page)
S south (down the page)
E east (to the right on the page)
W west (to the left on the page)
For example, suppose the robot starts on the north (top) side of Grid 1 and starts south (down). The path the robot follows is shown. The robot goes through 10 instructions in the grid before leaving the grid.
Compare what happens in Grid 2: the robot goes through 3 instructions only once, and then starts a loop through 8 instructions, and never exits.
You are to write a program that determines how long it takes a robot to get out of the grid or how the robot loops around.
Input
There will be one or more grids for robots to navigate. The data for each is in the following form. On the first line are three integers separated by blanks: the number of rows in the grid, the number of columns in the grid, and the number of the column in which the robot enters from the north. The possible entry columns are numbered starting with one at the left. Then come the rows of the direction instructions. Each grid will have at least one and at most 10 rows and columns of instructions. The lines of instructions contain only the characters N, S, E, or W with no blanks. The end of input is indicated by a row containing 0 0 0.
Output
For each grid in the input there is one line of output. Either the robot follows a certain number of instructions and exits the grid on any one the four sides or else the robot follows the instructions on a certain number of locations once, and then the instructions on some number of locations repeatedly. The sample input below corresponds to the two grids above and illustrates the two forms of output. The word "step" is always immediately followed by "(s)" whether or not the number before it is 1.
Sample Input
3 6 5NEESWEWWWESSSNWWWW4 5 1SESWEEESNWNWEENEWSEN0 0
Sample Output
10 step(s) to exit3 step(s) before a loop of 8 step(s)
Source
Mid-Central USA 1999
每个地图位置上都有一个方向,到达当前坐标,根据当前坐标的方向进行下一步的行走,给出起始位置,模拟机器人行走,可能会逃脱地图,输出步数,也可能会陷入死循环,也就是某个坐标位置第二次遇到,输出陷入循环前走的步数以及循环里面走的步数。模拟一下,判断是否有循环,到达坐标x,y的步数用step[x][y]存储,如果下一步是合法的行走(即没有越界,也没有重复访问),那么step[nextx][nexty]=step[x][y]+1,如果有循环,那么用单独一个变量duo来保存第二次到达某坐标位置所需要的步数,break掉。
代码:
0 0
- [ACM] hdu 1035 Robot Motion (模拟或DFS)
- [ACM] hdu 1035 Robot Motion (模拟或DFS)
- HDU 1035 Robot Motion (搜索-DFS)
- 【HDU 1035】Robot Motion(DFS)
- hdu 1035 Robot Motion(dfs)
- hdu 1035Robot Motion(dfs)
- hdu 1035 Robot Motion(dfs)
- HDU 1035 Robot Motion(大模拟)
- HDU 1035--Robot Motion(模拟)
- HDU 1035 Robot Motion (模拟)
- HDU 1035 Robot Motion(水题,模拟)
- HDU ACM 1035 Robot Motion 简单模拟题
- hdu 1035 Robot Motion(模拟)
- HDU 1035 Robot Motion 模拟
- hdoj 1035 Robot Motion (DFS+模拟)
- HDOJ(HDU).1035 Robot Motion (DFS)
- 【POJ】1573 & 【HDU】1035 - Robot Motion(模拟)
- HDU 1035 && poj 1573 Robot Motion【模拟】
- [ACM] hdu 2717 Catch That Cow (BFS)
- Get Luffy Out (poj 2723 二分+2-SAT)
- LeetCode之Recover Binary Search Tree
- OC之集合家族
- machine learning in coding(python):拼接原始数据;生成高次特征
- [ACM] hdu 1035 Robot Motion (模拟或DFS)
- c++学习笔记
- POJ 2750 Potted Flower(线段树 + DP)
- No package 'theoraenc' found gstreamer
- Maven私服搭建
- 抛硬币的模拟
- 四道面试题
- HDU 1312 Red and Black
- 模式 - KVC