POJ 2352 Star (树状数组)
来源:互联网 发布:2017淘宝双11活动规则 编辑:程序博客网 时间:2024/05/29 11:31
Description
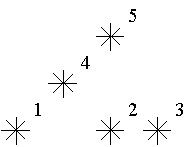
For example, look at the map shown on the figure above. Level of the star number 5 is equal to 3 (it's formed by three stars with a numbers 1, 2 and 4). And the levels of the stars numbered by 2 and 4 are 1. At this map there are only one star of the level 0, two stars of the level 1, one star of the level 2, and one star of the level 3.
You are to write a program that will count the amounts of the stars of each level on a given map.
Input
Output
题目很好理解,求每个星星的等级,我们可以一个一个按照顺序加星星,每加一次都对这个星星求前缀和,这个就是这个星星的等级,直接暴力求是要超时的,因为数据要在线增加,所以这里要用到树状数组来维护。
首先先来说一个lowbit(x),这个子函数的用处是来求x的二进制最低位的1及其后面的0所代表的数,比如38288 的二进制是 1001010110010000,那么lowbit(38288)=10000(二进制)=16(十进制),给出lowbit的求法:lowbit(x)=x&-x;
然后我们把一个数组中的下标用lowbit处理之后分成,形成类似于一棵树的状态:
其中的a数组为基础数组,而c数组这是我们要维护的数组,而c数组是怎么维护的,我们先来看一个结论:对于一个节点i,如果他是左子树,那么他的父节点就是i+lowbit(i),如果他的右子树,那么他的父节点就是i-lowbit(i).
然后我们再来维护c数组,c数组中的每个元素都是A数组中一段连续和,c[i]是以a[i]为终点的连续和,那么起点在哪里,我们就要用到上面的那个结论,cn表示的求以an为终点向左求lowbit(n)个数的连续和,也就是以该点为右节点,求从这个点的父节点+1开始到他本身的a元素的连续和,我们从左往右建立c数组。然后我们来求前缀和,用sum函数来求得:
int sum(int x){ int ret=0; while(x>0) { ret+=c[x]; x-=lowbit(x); } return ret;}如果我们修改a数组中的某个值,我们就需要更新c数组,更新函数如下:
void add(int x,int d) //x为坐标,d为修改的值的增减数{ while(x<=n) { c[x]+=d; x+=lowbit(x); }}
两个操作的时间复杂度都是logn,先对a数组进行预处理求出c数组,然后根据需要求和,在预处理中,我们进行n次add操作来建立树状数组既可。
最后附上本题的AC代码。(本题有一个坑点就是x可能等于0,在处理过程中应将x坐标后移一位保证以下标1开始)
#include<string.h>#include<algorithm>#include<stdio.h>using namespace std;int ans[40000];int c[40000];int n;int maxx,minx;inline int lowbit(int x){ return x&-x;}int sum(int x){ int ret=0; while(x>0) { ret+=c[x]; x-=lowbit(x); } return ret;}void add(int x,int d){ while(x<=33000) { c[x]+=d; x+=lowbit(x); }}int main(){ while(scanf("%d",&n)!=EOF) { memset(c,0,sizeof(c)); memset(ans,0,sizeof(ans)); int tempans; int tempx,tempy; for(int i=1;i<=n;i++) { scanf("%d%d",&tempx,&tempy); tempx++; add(tempx,1); tempans=sum(tempx); ans[tempans]++; } for(int i=1;i<=n;i++) printf("%d\n",ans[i]); }return 0;}
- poj 2352 star 树状数组
- 树状数组 POJ 2352 Star
- POJ 2352 Star 树状数组
- POJ 2352 Star (树状数组)
- POJ 2352 star (树状数组)
- POJ 2352 Star Treap||树状数组
- 树状数组入门之POJ 2352 Star
- poj 2352 star 树状数组的变型应用
- hdu1541 star 树状数组
- HDU 1541 star 树状数组
- POJ 2352 树状数组
- poj 2352 树状数组
- POJ 2352 树状数组
- poj 2352(树状数组)
- POJ 2352 树状数组
- POJ 2352 (树状数组)
- poj 2352 树状数组
- poj 2352树状数组
- [源码学习][知了开发]WebMagic四大组件-Downloader,Pipeline,PageProcesser
- DM368学习--捕获视频图像分辨率修改
- 简单换肤
- Linux 文件搜索指令 find locate whereis which type
- Visual Studio中11个强大的调试技巧和方法
- POJ 2352 Star (树状数组)
- Matlab实现将excel文件数据写到txt文件中
- Linux冷门却很重要的命令(三)---kill(pkill,killall)
- 统一配置管理-百度disconf
- HDU 5750 Dertouzos(数论)
- **BOOTSTRAP** Bootstrap简介
- Java的4种Json类库介绍
- 不要让孩子在最好的年纪做个只上补习班的“书呆子”
- @Configuration和@Bean的用法和理解