Android UI之ProgressBar(进度条)
来源:互联网 发布:手机解压缩软件 编辑:程序博客网 时间:2024/06/06 04:32
进度条是一个很实用的组件,一般用来显示用户某个耗时操作的进度百分比,首先来看一下Android支持的几种风格的进度条:
style="@android:style/Widget.ProgressBar.Inverse" 普通大小进度条
style="@android:style/Widget.ProgressBar.Large" 大进度条
style="@android:style/Widget.ProgressBar.Large.Inverse" 大进度条
style="@android:style/Widget.ProgressBar.Small" 小进度条
style="@android:style/Widget.ProgressBar.Small.Inverse" 小进度条
style="@android:style/Widget.ProgressBar.Horizontal"水平进度条
在样式文件中分别添加这六个不同样式的进度条,看看在模拟器上的效果
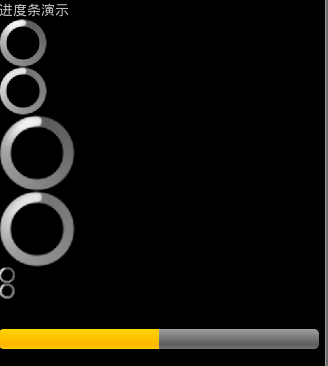
从上到下的六个进度条第一个没有声明样式,他默认的为和第二个一样是普通大小的进度条,第三个第四个分别是大进度条,第五个第六个是小进度条,最后一个是水平进度条
除了样式ProgressBar的几个常用属性:
android::max 设置进度条的最大值(最后一个水平进度条的设置为1000)
android::progress 设置当前的进度(最后一个水平进度条设置当前进度为500)
android::secondaryProgress 设置第二进度条
模拟一个进度条的进度变动:
效果: 0 0
- Android UI之ProgressBar(进度条)
- Android UI之ProgressBar(进度条)
- Android UI之ProgressBar(进度条)
- UI控件之ProgressBar(进度条)
- android UI篇 进度条 progressbar
- android 之进度条--------progressbar
- Android之进度条ProgressBar
- Android UI控件之ProgressBar进度条简单实现
- 【Android 开发】:UI控件之 ProgressBar 进度条的使用
- Android常用控件之进度条(Progressbar)
- Android高级组件之进度条 (progressBar)
- Android--UI之ProgressBar
- Android--UI之ProgressBar
- Android UI之ProgressBar
- JQuery UI 之进度条——Progressbar
- Android 控件之ProgressBar进度条
- Android 控件之ProgressBar进度条
- Android 控件之ProgressBar进度条
- Android中Path类的lineTo方法和quadTo方法画线的区别
- Tarjan 求无向图中的 关节点,和重连通分量
- STM32 USB部分中断函数简要分析
- Java多态性理解
- C文件操作
- Android UI之ProgressBar(进度条)
- 手机端滑动事件监听,可绑定多个DIV
- SPFA
- Viola-Jones technique
- 使用 Eclipse 远程调试 Java 应用程序
- ueditor1.4.3 单独上传文件以及图片的使用和配置
- STM32例程之USB HID双向数据传输(源码下载)
- C#之 浅复制学习案例
- HNOI 2010 弹飞绵羊LCT