Java NIO Channel
来源:互联网 发布:大数据入门经典书籍 编辑:程序博客网 时间:2024/05/18 19:19
Java NIO Channels are similar to streams with a few differences:
- You can both read and write to a Channels. Streams are typically one-way (read or write).
- Channels can be read and written asynchronously.
- Channels always read to, or write from, a Buffer.
As mentioned above, you read data from a channel into a buffer, and write data from a buffer into a channel. Here is an illustration of that:
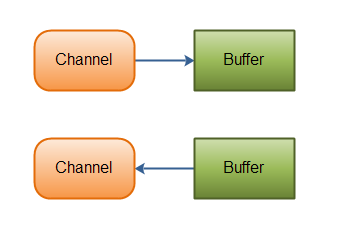
Channel Implementations
Here are the most important Channel implementations in Java NIO:
- FileChannel
- DatagramChannel
- SocketChannel
- ServerSocketChannel
The FileChannel
reads data from and to files.
The DatagramChannel
can read and write data over the network via UDP.
The SocketChannel
can read and write data over the network via TCP.
The ServerSocketChannel
allows you to listen for incoming TCP connections, like a web server does. For each incoming connection a SocketChannel
is created.
Basic Channel Example
Here is a basic example that uses a FileChannel
to read some data into a Buffer
:
RandomAccessFile aFile = new RandomAccessFile("data/nio-data.txt", "rw"); FileChannel inChannel = aFile.getChannel(); ByteBuffer buf = ByteBuffer.allocate(48); int bytesRead = inChannel.read(buf); while (bytesRead != -1) { System.out.println("Read " + bytesRead); buf.flip(); while(buf.hasRemaining()){ System.out.print((char) buf.get()); } buf.clear(); bytesRead = inChannel.read(buf); } aFile.close();
Notice the buf.flip()
call. First you read into a Buffer. Then you flip it. Then you read out of it. I'll get into more detail about that in the next text about Buffer
's.
- Java NIO Channel
- Java NIO Socket Channel
- Java NIO(3-Channel)
- Java NIO Channel
- Java NIO笔记 Channel
- Java NIO Channel
- Java NIO--Channel
- java NIO Channel
- Java NIO (二) Channel
- Java NIO Channel
- Java NIO Channel
- java NIO-Channel
- Java NIO Channel
- Java NIO之Channel
- java nio channel
- Java NIO Channel tranfer
- Java NIO Channel
- java NIO Channel详解
- Android中Serializable和Parcelable的使用
- 出口托收与进口代收
- linux c语言 select函数用法
- 1074. Reversing Linked List
- Javascript面向对象编程(三):非构造函数的继承
- Java NIO Channel
- Cannot change version of project facet Dynamic Web Module to 2.5解决方案
- D3D11教程二十六之Blur(模糊处理)
- eclipse中egit无法访问github问题
- POJ 2442 Sequence
- eclipse安装Spring插件
- 比较数组中最大值最小值
- 区块链应用开发入门
- 更改Yelee主题标签云为球形标签云