tabcontrol 自绘
来源:互联网 发布:淘宝用卷怎么用 编辑:程序博客网 时间:2024/06/06 01:08
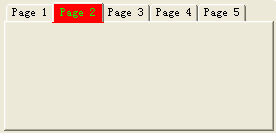
//头文件
- #pragma once
-
-
-
-
-
- class COwnerdrawTabCtrl : public CTabCtrl
- {
-
- public:
- COwnerdrawTabCtrl();
-
-
- public:
-
-
- public:
-
-
-
-
- public:
- virtual BOOL Create(LPCTSTR lpszClassName, LPCTSTR lpszWindowName, DWORD dwStyle, const RECT& rect, CWnd* pParentWnd, UINT nID, CCreateContext* pContext = NULL);
- protected:
- virtual void PreSubclassWindow();
-
-
-
- public:
- virtual ~COwnerdrawTabCtrl();
-
-
- protected:
-
-
-
- void DrawItem(LPDRAWITEMSTRUCT lpDrawItemStruct);
-
- DECLARE_MESSAGE_MAP()
- };
//CPP实现
-
-
-
- #include "stdafx.h"
- #include "OwnerdrawTabCtrl.h"
-
- #ifdef _DEBUG
- #define new DEBUG_NEW
- #undef THIS_FILE
- static char THIS_FILE[] = __FILE__;
- #endif
-
-
-
-
- COwnerdrawTabCtrl::COwnerdrawTabCtrl()
- {
- }
-
- COwnerdrawTabCtrl::~COwnerdrawTabCtrl()
- {
- }
-
-
- BEGIN_MESSAGE_MAP(COwnerdrawTabCtrl, CTabCtrl)
-
-
- END_MESSAGE_MAP()
-
-
-
-
- void COwnerdrawTabCtrl::DrawItem(LPDRAWITEMSTRUCT lpDrawItemStruct)
- {
-
-
- if(lpDrawItemStruct->CtlType == ODT_TAB)
- {
- CRect rect = lpDrawItemStruct->rcItem;
- INT nTabIndex = lpDrawItemStruct->itemID;
- if (nTabIndex < 0) return;
-
- TCHAR label[64];
- TC_ITEM tci;
- tci.mask = TCIF_TEXT|TCIF_IMAGE;
- tci.pszText = label;
- tci.cchTextMax = 63;
- GetItem(nTabIndex, &tci );
-
- CDC* pDC = CDC::FromHandle(lpDrawItemStruct->hDC);
- if (!pDC) return;
- int nSavedDC = pDC->SaveDC();
-
-
- COLORREF rcBack;
- if (lpDrawItemStruct->itemState & CDIS_SELECTED )
- {
- rcBack = RGB(255, 0, 0);
- }
- else if(lpDrawItemStruct->itemState & (CDIS_DISABLED | CDIS_GRAYED) )
- {
- rcBack = RGB(0, 255, 0);
- }
- else
- {
- rcBack = GetSysColor(COLOR_BTNFACE);
- }
- pDC->FillSolidRect(rect, rcBack);
-
- rect.top += ::GetSystemMetrics(SM_CYEDGE);
-
- pDC->SetBkMode(TRANSPARENT);
-
-
- CImageList* pImageList = GetImageList();
- if (pImageList && tci.iImage >= 0)
- {
- rect.left += pDC->GetTextExtent(_T(" ")).cx;
-
-
- IMAGEINFO info;
- pImageList->GetImageInfo(tci.iImage, &info);
- CRect ImageRect(info.rcImage);
- INT nYpos = rect.top;
-
- pImageList->Draw(pDC, tci.iImage, CPoint(rect.left, nYpos), ILD_TRANSPARENT);
- rect.left += ImageRect.Width();
- }
-
-
- COLORREF txtColor;
- if (lpDrawItemStruct->itemState & CDIS_SELECTED )
- {
- rect.top -= ::GetSystemMetrics(SM_CYEDGE);
-
- txtColor = RGB(0,255,0);
- }
- else if(lpDrawItemStruct->itemState & (CDIS_DISABLED | CDIS_GRAYED) )
- {
- txtColor = RGB(128, 128, 128);
- }
- else
- {
- txtColor = GetSysColor(COLOR_WINDOWTEXT);
- }
- pDC->SetTextColor(txtColor);
- pDC->DrawText(label, rect, DT_SINGLELINE|DT_VCENTER|DT_CENTER);
-
- pDC->RestoreDC(nSavedDC);
-
- }
-
- }
-
- void COwnerdrawTabCtrl::PreSubclassWindow()
- {
- ModifyStyle(0, TCS_OWNERDRAWFIXED);
- CTabCtrl::PreSubclassWindow();
- }
-
- BOOL COwnerdrawTabCtrl::Create(LPCTSTR lpszClassName, LPCTSTR lpszWindowName, DWORD dwStyle, const RECT& rect, CWnd* pParentWnd, UINT nID, CCreateContext* pContext)
- {
-
- dwStyle |= TCS_OWNERDRAWFIXED;
- return CWnd::Create(lpszClassName, lpszWindowName, dwStyle, rect, pParentWnd, nID, pContext);
- }
0 0