MySQL: 基于 Android 远程连接
来源:互联网 发布:java webservice教程 编辑:程序博客网 时间:2024/04/28 05:54
MySQL: 基于 Android 远程连接
程序效果图
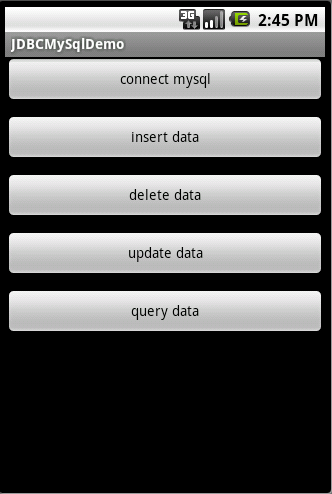
项目结构
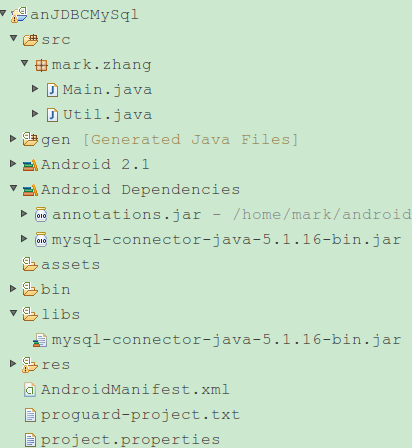
项目文件中新建 libs 目录,将 jdbc 的 jar 文件放到里面。
这样 adt 插件自动将该 jar 添加到 build path.
数据库表里面的原始数据

插入数据

删除数据
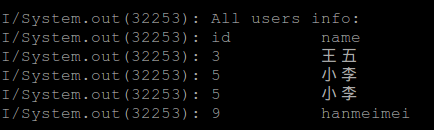
更新数据
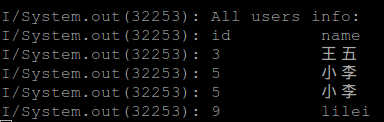
Main.Java
- package mark.zhang;
-
- import java.sql.Connection;
- import java.sql.SQLException;
-
- import android.app.Activity;
- import android.os.Bundle;
- import android.view.View;
-
- public class Main extends Activity {
-
- private static final String REMOTE_IP = "192.168.1.102";
- private static final String URL = "jdbc:mysql://" + REMOTE_IP + "/mydb";
- private static final String USER = "mark";
- private static final String PASSWORD = "123456";
-
- private Connection conn;
-
- @Override
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.main);
- }
-
- public void onConn(View view) {
- conn = Util.openConnection(URL, USER, PASSWORD);
- }
-
- public void onInsert(View view) {
- String sql = "insert into mytable values(9, 'hanmeimei')";
- Util.execSQL(conn, sql);
- }
-
- public void onDelete(View view) {
- String sql = "delete from mytable where name='mark'";
- Util.execSQL(conn, sql);
- }
-
- public void onUpdate(View view) {
- String sql = "update mytable set name='lilei' where name='hanmeimei'";
- Util.execSQL(conn, sql);
- }
-
- public void onQuery(View view) {
- System.out.println("All users info:");
- Util.query(conn, "select * from mytable");
- }
-
- @Override
- protected void onDestroy() {
- super.onDestroy();
- if (conn != null) {
- try {
- conn.close();
- } catch (SQLException e) {
- conn = null;
- } finally {
- conn = null;
- }
- }
- }
- }
Util.java
- package mark.zhang;
-
- import java.sql.Connection;
- import java.sql.DriverManager;
- import java.sql.ResultSet;
- import java.sql.SQLException;
- import java.sql.Statement;
-
- public class Util {
-
- public static Connection openConnection(String url, String user,
- String password) {
- Connection conn = null;
- try {
- final String DRIVER_NAME = "com.mysql.jdbc.Driver";
- Class.forName(DRIVER_NAME);
- conn = DriverManager.getConnection(url, user, password);
- } catch (ClassNotFoundException e) {
- conn = null;
- } catch (SQLException e) {
- conn = null;
- }
-
- return conn;
- }
-
- public static void query(Connection conn, String sql) {
-
- if (conn == null) {
- return;
- }
-
- Statement statement = null;
- ResultSet result = null;
-
- try {
- statement = conn.createStatement();
- result = statement.executeQuery(sql);
- if (result != null && result.first()) {
- int idColumnIndex = result.findColumn("id");
- int nameColumnIndex = result.findColumn("name");
- System.out.println("id\t\t" + "name");
- while (!result.isAfterLast()) {
- System.out.print(result.getString(idColumnIndex) + "\t\t");
- System.out.println(result.getString(nameColumnIndex));
- result.next();
- }
- }
- } catch (SQLException e) {
- e.printStackTrace();
- } finally {
- try {
- if (result != null) {
- result.close();
- result = null;
- }
- if (statement != null) {
- statement.close();
- statement = null;
- }
-
- } catch (SQLException sqle) {
-
- }
- }
- }
-
- public static boolean execSQL(Connection conn, String sql) {
- boolean execResult = false;
- if (conn == null) {
- return execResult;
- }
-
- Statement statement = null;
-
- try {
- statement = conn.createStatement();
- if (statement != null) {
- execResult = statement.execute(sql);
- }
- } catch (SQLException e) {
- execResult = false;
- }
-
- return execResult;
- }
- }
main.xml
- <?xml version="1.0" encoding="utf-8"?>
- <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- android:orientation="vertical" >
-
- <Button
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:layout_marginBottom="10dip"
- android:onClick="onConn"
- android:text="connect mysql" />
-
- <Button
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:layout_marginBottom="10dip"
- android:onClick="onInsert"
- android:text="insert data" />
-
- <Button
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:layout_marginBottom="10dip"
- android:onClick="onDelete"
- android:text="delete data" />
-
- <Button
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:layout_marginBottom="10dip"
- android:onClick="onUpdate"
- android:text="update data" />
-
- <Button
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:onClick="onQuery"
- android:text="query data" />
-
- </LinearLayout>
manifest.xml
- <?xml version="1.0" encoding="utf-8"?>
- <manifest xmlns:android="http://schemas.android.com/apk/res/android"
- package="mark.zhang"
- android:versionCode="1"
- android:versionName="1.0" >
-
- <uses-sdk android:minSdkVersion="7" />
-
- <application
- android:icon="@drawable/ic_launcher"
- android:label="@string/app_name" >
- <activity
- android:name=".Main"
- android:label="@string/app_name" >
- <intent-filter>
- <action android:name="android.intent.action.MAIN" />
-
- <category android:name="android.intent.category.LAUNCHER" />
- </intent-filter>
- </activity>
- </application>
-
- <uses-permission android:name="android.permission.INTERNET"/>
-
- </manifest>
注意: <uses-permission android:name="android.permission.INTERNET"/>
-----------------------------------------------------------------------------
2016.7.28 更新
-----------------------------------------------------------------------------
首先非常感谢@lyz12600对工程的整理.
大家可以去下载 AndroidStudio 的代码, 运行, 有问题多交流.
下载地址:http://download.csdn.net/detail/lyz12600/9581532
再次感谢@lyz12600.
-----------------------------------------------------------------------------
原文链接:http://blog.csdn.net/veryitman/article/details/7764894