Protect Your Flash Files From Decompilers by Using Encryption
来源:互联网 发布:手机挂机赚钱软件 编辑:程序博客网 时间:2024/04/30 13:36
Decompilers are a real worry for people who create Flash content. You can put a lot of effort into creating the best game out there, then someone can steal it, replace the logo and put it on their site without asking you. How? Using a Flash Decompiler. Unless you put some protection over your SWF it can be decompiled with a push of a button and the decompiler will output readable source code.
In this tutorial I will demonstrate a technique I use to protect code and assets from theft.
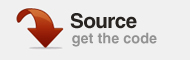
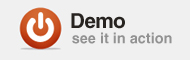
Editor’s Note: Thanks to Vaclav for use of his lock icon. Visit Psdtuts+ to see how he made it..
Before We Begin
I used a small project of mine to demonstrate how vulnerable SWFs are to decompilation. You can download it and test yourself via the source link above. I used Sothink SWF Decompiler 5 to decompile the SWF and look under its hood. The code is quite readable and you can understand and reuse it fairly easily.
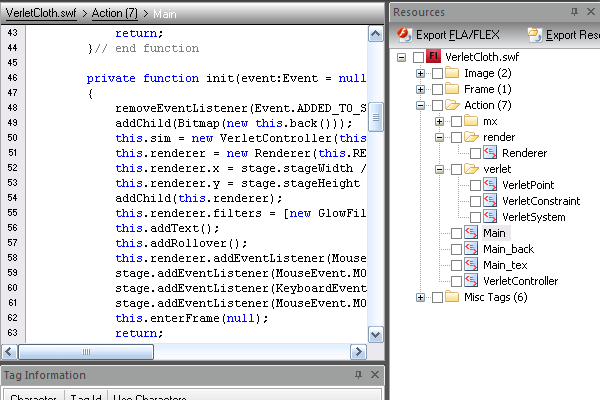
What Can We do About it?
I came up with a technique for protecting SWFs from decompilers and I’m going to demonstrate it in this tutorial. We should be able to produce this:
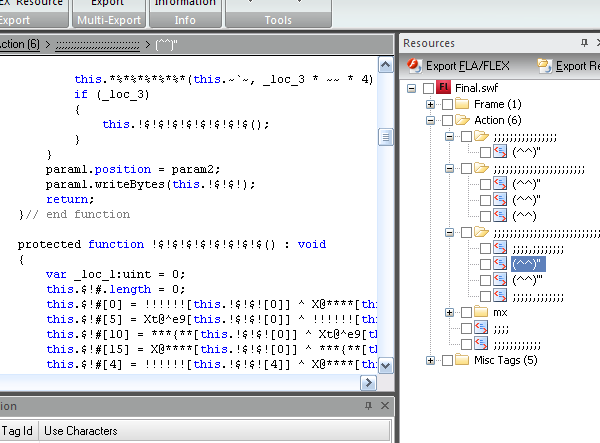
The code that is decompiled is actually the code for decrypting the content and has nothing to do with your main code. Additionally, the names are illegal so it won’t compile back. Try to decompile it yourself.
Before we get going, I want to point out that this tutorial is not suitable for beginners and you should have solid knowledge of AS3 if you want to follow along. This tutorial is also about low level programming that involves bytes, ByteArrays and manipulating SWF files with a hex editor.
Here’s what we need:
- A SWF to protect. Feel free to download the SWF I’ll be working on.
- Flex SDK. We will be using it to embed content using the Embed tag. You can download it from opensource.adobe.com.
- A hex editor. I’ll be using a free editor called Hex-Ed. You can download it from nielshorn.net or you can use an editor of your choice.
- A decompiler. Whilst not necessary, it would be nice to check if our protection actually works. You can grab a trial of Sothink SWF Decompiler from sothink.com
Step 1: Load SWF at Runtime
Open a new ActionScript 3.0 project, and set it to compile with Flex SDK (I use FlashDevelop to write code). Choose a SWF you want to protect and embed it as binary data using the Embed tag:
Now the SWF is embedded as a ByteArray into the loader SWF and it can be loaded through Loader.loadBytes().
In the end we should have this code:
Compile and see if it works (it should). From now on I will call the embedded SWF the “protected SWF”, and the SWF we just compiled the “loading SWF”.
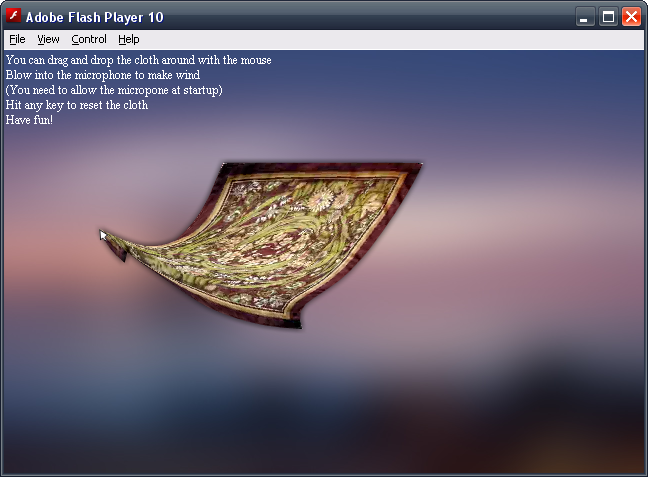
Step 2: Analyze the Result
Let’s try to decompile and see if it works.
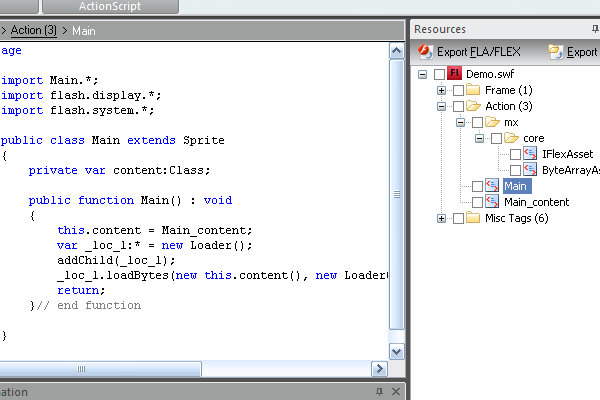
Yey! The assets and the original code are gone! What’s shown now is the code that loads the protected SWF and not its content. This would probably stop most of the first-time attackers who are not too familiar with Flash but it’s still not good enough to protect your work from skilled attackers because the protected SWF is waiting for them untouched inside the loading SWF.
Step 3: Decompressing the SWF
Let’s open the loading SWF with a hex editor:
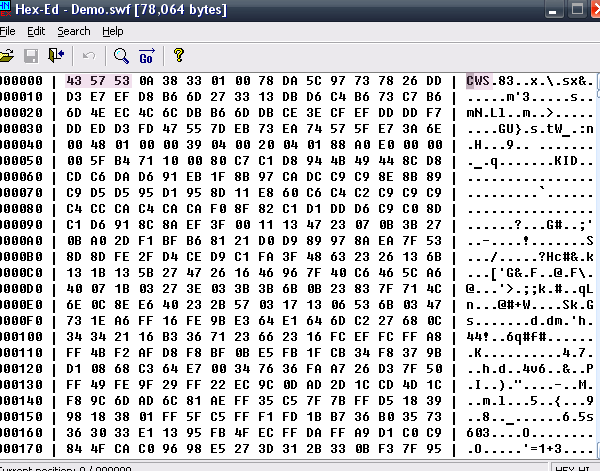
It should look like random binary data because it’s compressed and it should begin with ASCII “CWS”. We need to decompress it! (If your SWF begins with “FWS” and you see meaningful strings in the SWF it’s likely that it didn’t get compressed. You have to enable compression to follow along).
At first it might sound difficult but it’s not. The SWF format is an open format and there is a document that describes it. Download it from adobe.com and scroll down to page 25 in the document. There is a description of the header and how the SWF is compressed, so we can uncompress it easily.
What is written there is that the first 3 bytes are a signature (CWS or FWS), the next byte is the Flash version, the next 4 bytes are the size of the SWF. The remaining is compressed if the signature is CWS or uncompressed if the signature is FWS. Let’s write a simple function to decompress a SWF:
The function does a few things:
- It reads the uncompressed header (the first 8 bytes) without the signature and remembers it.
- It reads the rest of the data and uncompresses it.
- It writes back the header (with the “FWS” signature) and the uncompressed data, creating a new, uncompressed SWF.
Step 4: Creating a Utility
Next we’ll create a handy utility in Flash for compressing and decompressing SWF files. In a new AS3 project, compile the following class as a document class:
As you probably noticed I’ve added 2 things: File loading and the compress function.
The compress function is identical to the decompress function, but in reverse. The file loading is done using FileReference (FP10 required) and the loaded file is either compressed or uncompressed. Note that you have to run the SWF locally from a standalone player, as FileReference.browse() must be invoked by user interaction (but the local standalone player allows to run it without).
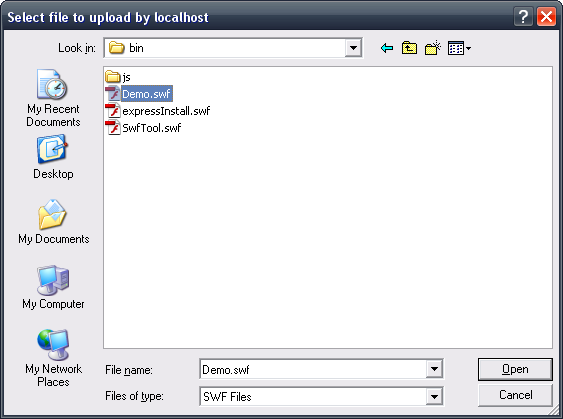
Step 5: Uncompressing the Loading SWF
To test the tool, fire it up, select the loading SWF and choose where to save it. Then open it up with a hex editor and scrub through. You should see ascii strings inside like this:
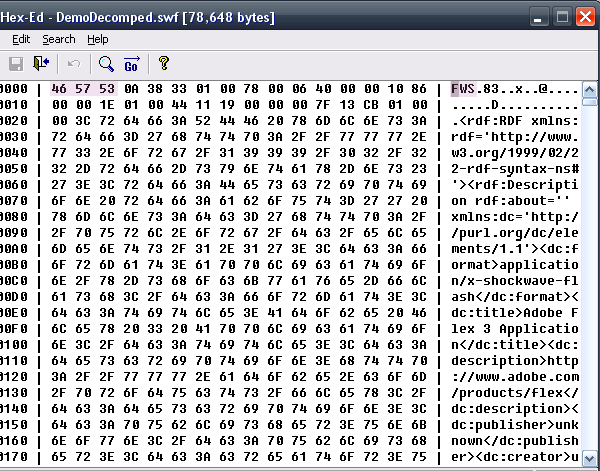
Step 6: Analyze Again
Let’s return back to step 2. While the decompiler didn’t show any useful info about the protected SWF, it’s quite easy to get the SWF from the now uncompressed loader; just search for the signature “CWS” (if the protected SWF is uncompressed search for “FWS”) and see the results:
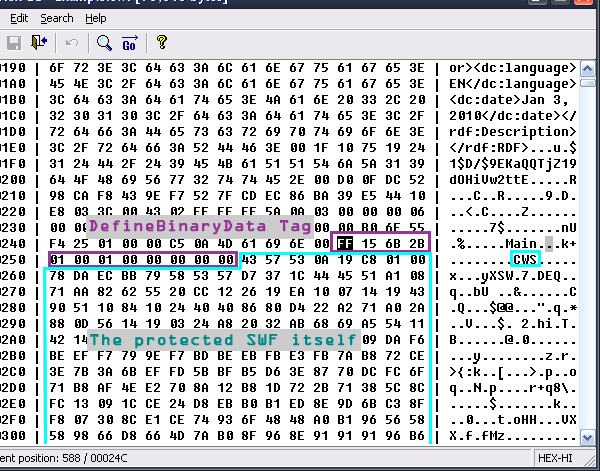
What we found is a DefineBinaryData tag that contains the protected SWF, and extracting it from there is a piece of cake. We are about to add another layer of protection over the loading SWF : Encryption.
Step 7: Encryption
To make the protected SWF less “accessible” we will add some kind of encryption. I chose to use as3crypto and you can download it from code.google.com. You can use any library you want instead (or your own implementation, even better), the only requirement is that it should be able to encrypt and decrypt binary data using a key.
Step 8: Encrypting Data
The first thing we want to do is write a utility to encrypt the protected SWF before we embed it. It requires very basic knowledge of the as3crypto library and it’s pretty straightforward. Add the library into your library path and let’s begin by writing the following:
What’s going on here? We use a class from as3crypto called AESKey to encrypt the content. The class encrypts 16 bytes in a time (128-bit), and we have to for-loop over the data to encrypt it all. Note the second line : data.length & ~15. It makes sure that the number of bytes encrypted can be divided by 16 and we don’t run out of data when calling aes.encrypt().
Note: It’s important to understand the point of encryption in this case. It’s not really encryption, but rather obfuscation since we include the key inside the SWF. The purpose is to turn the data into binary rubbish, and the code above does it’s job, although it can leave up to 15 unencrypted bytes (which doesn’t matter in our case). I’m not a cryptographer, and I’m quite sure that the above code could look lame and weak from a cryptographer’s perspective, but as I said it’s quite irrelevant as we include the key inside the SWF.
Step 9: Encryption Utility
Time to create another utility that will help us encrypt SWF files. It’s almost the same as the compressor we created earlier, so I won’t talk much about it. Compile it in a new project as a document class:
Now run it, and make an encrypted copy of the protected SWF by selecting it first and then saving it under a different name.
Step 10: Modifying the Loader
Return back to the loading SWF project. Because the content is now encrypted we need to modify the loading SWF and add decryption code into it. Don’t forget to change the src in the Embed tag to point to the encrypted SWF.
This is the same as before except with the decryption code stuck in the middle. Now compile the loading SWF and test if it works. If you followed carefully up to now, the protected SWF should load and display without errors.
Step 11: Look Inside Using a Decompiler
Open the new loading SWF with a decompiler and have a look.
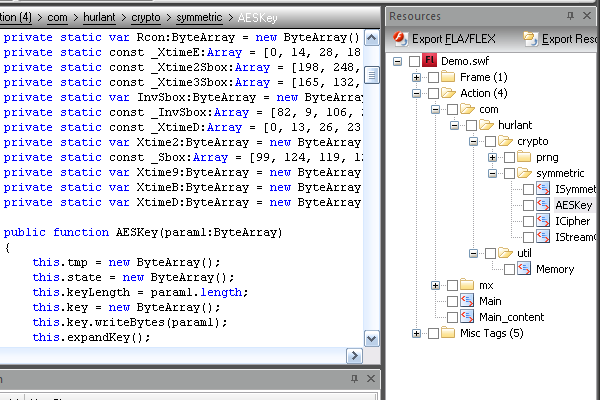
It contains over a thousand lines of tough looking encryption code, and it’s probably harder to get the protected SWF out of it. We’ve added a few more steps the attacker must undertake:
- He (or she) has to find the DefineBinaryData that holds the encrypted content and extract it.
- He must create a utility to decrypt it.
The problem is that creating a utility is as simple as copy-pasting from the decompiler into the code editor and tweaking the code a little bit. I tried to break my protection myself, and it was quite easy – I managed to do it in about 5 minutes. So we’re going to have to take some measurements against it.
Step 12: String Obfuscation
First we’d put the protected SWF into the loading SWF, then encrypted it, and now we’ll put the final touches to the loading SWF. We’ll rename classes, functions and variables to illegal names.
By saying illegal names I mean names such as ,;!@@,^#^ and (^_^). The cool thing is that this matters to the compiler but not to the Flash Player. When the compiler encounters illegal characters inside identifiers, it fails to parse them and thus the project fails to compile. On the other hand, the Player doesn’t have any problems with those illegal names. We can compile the SWF with legal identifiers, decompress it and rename them to a bunch of meaningless illegal symbols. The decompiler will output illegal code and the attacker will have to go over the hundreds of lines of code manually, removing illegal identifiers before he can compile it. He deserves it!
This is how it looks before any string obfuscation:
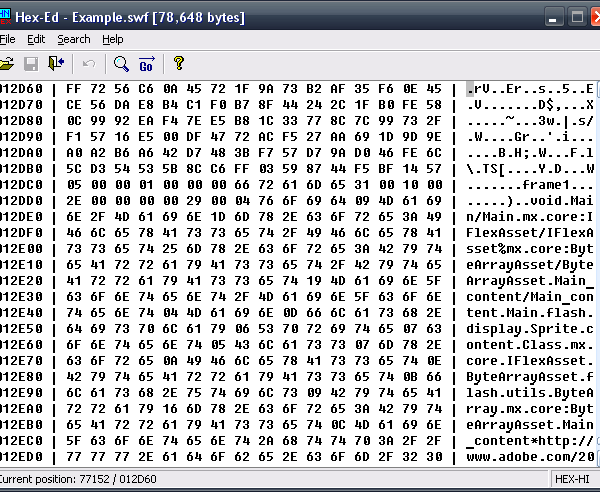
Let’s start! Decompress the loading SWF using the utility we created before and fire up a hex editor.
Step 13: Your First Obfuscation
Let’s try to rename the document class. Assuming you’ve left the original name (Main), let’s search for it in the uncompressed loader SWF with a hex editor:
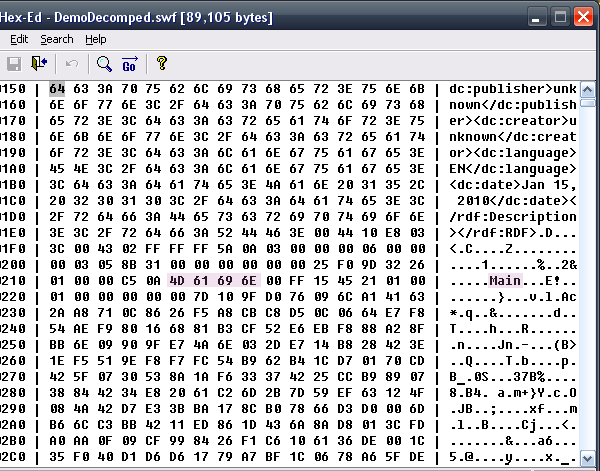
Rename “Main” to ;;;;. Now search for other “Main”s and rename them to ;;;; too.
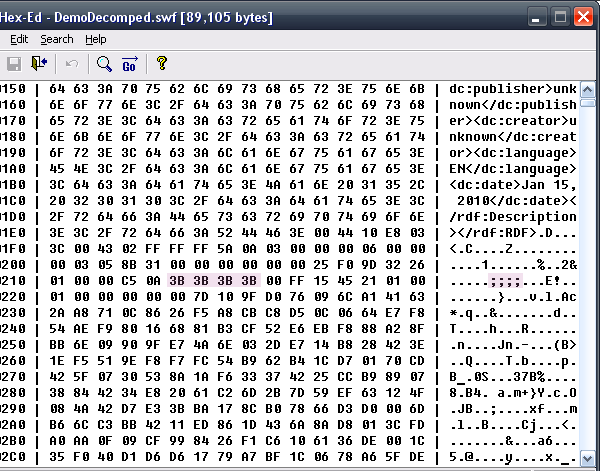
When renaming make sure that you don’t rename unnecessary strings or the SWF will not run.
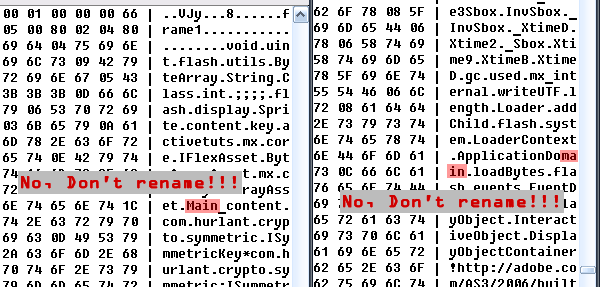
Save and run the SWF. It works! And look what the decompiler says:
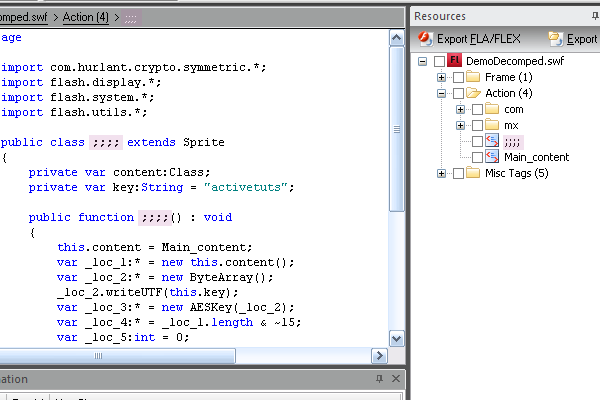
Victory!! :)
Step 14: Renaming the Rest of the Classes
Keep renaming the rest of your classes. Choose a class name and search for it, replacing it with illegal symbols until you reach the end of the file. As I said, the most important thing here is to use your common sense, make sure you don’t mess your SWF up. After renaming the classes you can start renaming the packages. Note that when renaming a package, you can erase the periods too and make it one long illegal package name. Look what I made:
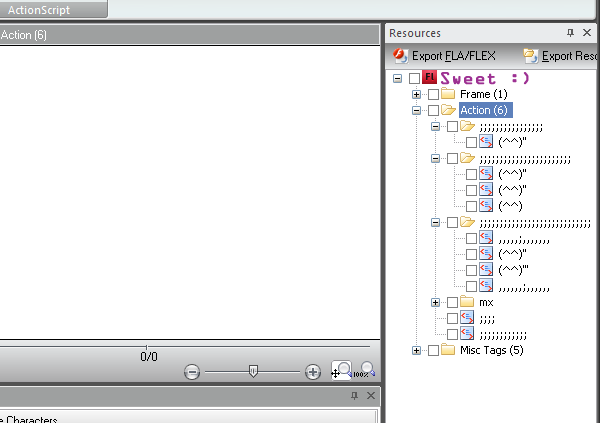
After you finish renaming the classes and the packages, you can start renaming functions and variables. They are even easier to rename as they usually appear only once, in one large cloud. Again, make sure you rename only “your” methods and not the built-in Flash methods. Make sure you don’t wipe out the key (“activetuts” in our case).
Step 15: Compress the SWF
After you finish renaming you would probably want to compress the SWF so it will be smaller in size. No problem, we can use the compressing utility we created before and it will do the job. Run the utility, select the SWF and save it under another name.
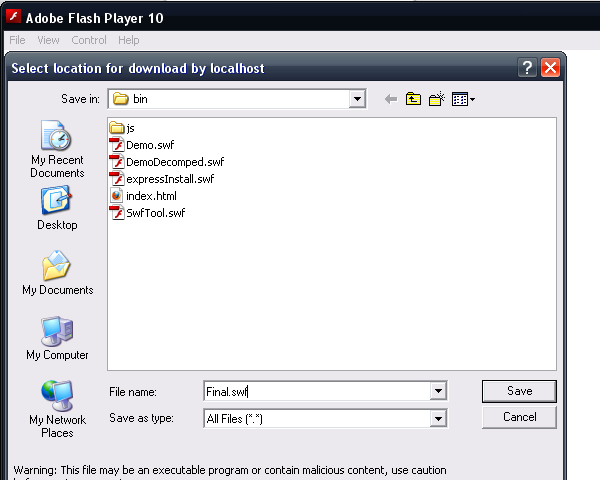
Conclusion: Have a Final Look
Open it one last time and have a look. The classes, the variables and the method names are obfuscated and the protected SWF is somewhere inside, encrypted. This technique could be slow to apply at first, but after a few times it takes only a few minutes.
A while ago I created an automatic utility to inject the protected SWF for me into the loading SWF, and it worked fine. The only problem is that if it can be injected using an automatic utility, it can be decrypted using another utility, so if the attacker makes a utility for that he will get all your SWF easily. Because of this I prefer to protect the SWFs manually each time, adding a slight modification so it would be harder to automate.
Another nice application of the technique is Domain locking. Instead of decrypting the SWF with a constant string you can decrypt it with the domain the SWF is currently running on. So instead of having an if statement to check the domain, you can introduce a more powerful way to protect the SWF from placement on other sites.
Last thing, you may want to replace the encryption code with your own implementation. Why? We invested efforts in making the crypto code illegal, but the code we use is from a popular open source library and the attacker could recognize it as such. He will download a clean copy, and all the obfuscation work is rendered unnecessary. On the other hand, using your own implementation will require him to fix all the illegal names before he can continue.
Other Protection Methods
Because SWF theft is a big problem in the Flash world, there are other options for protecting SWFs. There are numerous programs out there to obfuscate AS on the bytecode level (like Kindisoft’s secureSWF). They mess up the compiled bytecode and when the decompiler attempts to output code it will fail, and even crash sometimes. Of course this protection is better in terms of security but it costs $$$, so before choosing how to protect your SWF consider the amount of security needed. If it’s about protecting a proprietary algorithm your 50-employee Flash studio has been developing for the past two years, you may consider something better then renaming the variables. On the other hand if you want to prevent the kiddies from submitting false high scores you may consider using this technique.
What I like about this technique is the fact that your protected SWF is left untouched when run. AS obfuscation tampers with the byte code and it could possibly damage the SWF and cause bugs (although I haven’t encountered any myself).
That’s all for today, hope you enjoyed the tutorial and learned something new! If you have any questions feel free to drop a comment.
link:http://active.tutsplus.com/tutorials/workflow/protect-your-flash-files-from-decompilers-by-using-encryption/
- Protect Your Flash Files From Decompilers by Using Encryption
- Protect Your Flash Files From Decompilers by Using Encryption
- Protect Flash Files from Being Downloaded
- Using files from web applications
- How to Download Embedded Flash Files using your Browser 从浏览器下载网页内嵌的Flash文件的方法
- Protect Your Windows Network : From Perimeter to Data
- Shared storage cannot protect your application from code injection attacks
- Using xcconfig files for your XCode Project
- Protect your applications against advanced reverse engineering and software cracking by AntiDebugLIB By Jim Charles
- Protect your java code
- Protect your eyes, NOW!
- protect your eye
- e-Commerce Bot Attacks! Learn How To Protect Your Website From Price Scraping
- 10 Easy Ways To Protect Your Eyes From The Harms of Digital Devices
- Protect your applications against advanced reverse engineering and software cracking by AntiDebugLIB
- Using Padding in Encryption
- Using Padding in Encryption
- Using Hadoop Encryption Zone
- 理想
- Ubuntu10.04修复grub
- Silverlight调用WebService时出现的跨域方式访问服务
- qq克隆匹配DOM,并选中当前克隆匹配元素集合
- 转 .NET 运行全程简析
- Protect Your Flash Files From Decompilers by Using Encryption
- Android平台硬件调试之Camera篇
- SQL SERVER PIVOT 行列转换
- 部分IT公司笔试算法题(转)
- 创智播客 .NET 文章精华 没看完 存个传送门在这里
- dfasdfasdf
- 如何在 Windows CE 5.0 中开发和测试设备驱动程序
- Windows PowerShell 2.0 开发之命令别名
- 怎么样可以把网站域名的cookies代码套出来?