FW:Reverse a Singly Linked List Recursively in Java
来源:互联网 发布:灯珠花 淘宝 编辑:程序博客网 时间:2024/04/28 21:02
http://www.technicalypto.com/2010/03/reverse-singly-linked-list-recursively.html
Reverse a Singly Linked List Recursively in Java
We have already seen how to reverse a singly linked listwith illustrative pictures. Now lets see how we can do it recursively.In the previous problem we did it iteratively, now we shall do itrecursively.
To attack any problem in a recursive approach, we need to be very clearabout the end/boundary conditions. For a linked list, reverse of a nulllist or reverse of list of size 1 is going to be the same.
Reverse of a linked list of size x will be the reverse of the 'next' element followed by first.
A picture means a thousand words. So, here is what happens internally.
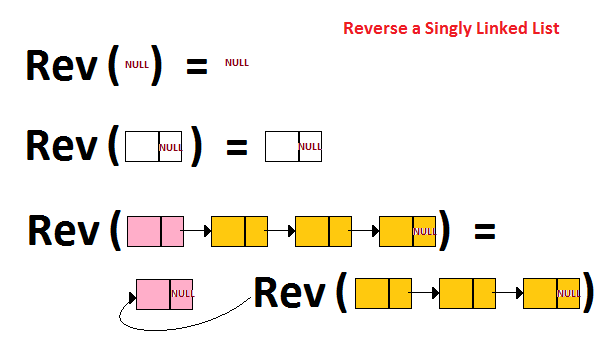
Now for the comprehensive Java code (reference for SinglyLinkedList implementation can be found here)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
package
dsa.linkedlist;
public
class
ReverseLinkedListRecursively {
public
static
void
main(String args[]){
ReverseLinkedListRecursively reverser =
new
ReverseLinkedListRecursively();
SinglyLinkedList<Integer> originalList = reverser.getLabRatList(
10
);
System.out.println(
"Original List : "
+originalList.toString());
originalList.start = reverser.reverse(originalList.start);
System.out.println(
"Reversed List : "
+originalList.toString());
}
public
Node<Integer> reverse(Node<Integer> list)
{
if
(list ==
null
|| list.next==
null
)
return
list;
Node<Integer> nextItem = list.next;
list.next =
null
;
Node<Integer> reverseRest = reverse(nextItem);
nextItem.next = list;
return
reverseRest;
}
private
SinglyLinkedList<Integer> getLabRatList(
int
count){
SinglyLinkedList<Integer> sampleList =
new
SinglyLinkedList<Integer>();
for
(
int
i=
0
;i<count;i++){
sampleList.add(i);
}
return
sampleList;
}
}
/*
* SAMPLE OUTPUT
* Original List : 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
* Reversed List : 9, 8, 7, 6, 5, 4, 3, 2, 1, 0
*/
- FW:Reverse a Singly Linked List Recursively in Java
- Reverse a singly linked list python
- LeecCode Reverse Linked List; Reverse a singly linked list.
- Reverse singly linked list using Java
- 两两反转单向链表 (reverse a singly linked list in pair ) [#22]
- 反转单向链表(reverse a singly linked list)
- Finding a Loop in a Singly Linked List
- 单链表反转的递归实现(Reversing a Linked List in Java, recursively)
- 如何反转一个单链表 How to reverse a singly linked list
- 反转单向链表(reverse a singly linked list)(单个反转) [# 7]
- 单链表Singly Linked List之JAVA实现
- 如何判断 单链表中是否存在环 ( How judges in Singly Linked List whether has a circle )
- How to determine whether there are circles in a singly linked list?
- Ch2-3: remove the middle node in a singly linked list
- 倒转单向链表(Reverse Singly Linked List)
- 【Leetcode】Reverse Linked List II in JAVA
- reverse a linked list
- Reverse a linked list.
- 处理表重复记录(查询和删除)
- 国米OUT,意甲酱油了
- HDOJ-Monkey and Banana-动态规划
- [转载]C#中使用WindowsSDK
- Ubuntu下django安装
- FW:Reverse a Singly Linked List Recursively in Java
- 用ssh在虚拟机与宿主机之间传送文件的一些问题
- Spring 动态切入点讲解
- 数据云URL过滤技术(zz 51cto)
- 超链接点击范围扩大到外层包含元素
- 敏捷合同
- jQuery对象与DOM对象之间的转换(转自:http://wozailongyou.iteye.com/blog/299311)
- [详解+多图]Android开发环境搭建超级详细
- LiveZilla 详细 配置 设置 (三) 配置 LiveZilla 服务