SRM527 275 DP
来源:互联网 发布:手机淘宝装修 编辑:程序博客网 时间:2024/06/06 02:23
NOTE: This problem statement contains images that may not display properly if viewed outside of the applet.
You want to build a graph consisting of N nodes and N-1 edges. The graph must be connected. That is, for each pair of nodes there must be some sequence of edges that connects them. For example, the following picture shows a connected graph with N=5 nodes (dots) and N-1=4 edges (lines connecting pairs of dots):
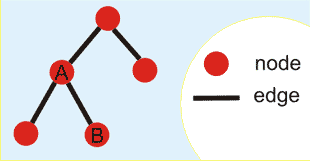
An edge is adjacent to the two nodes that it connects. The degree of a node in the graph is equal to the number of edges adjacent to the node. For example, the degree of node A in the picture above is 3, while the degree of node B is 1. Note that in your graph the degree of each node will be between 1 and N-1, inclusive.
You are given a vector <int> scores with N-1 elements. The score of a node with degree d isscores[d-1]. The score of a graph is the sum of the scores of its nodes.
Your method should compute and return the maximum possible score for a graph you can construct.
Definition
Class:P8XGraphBuilderMethod:solveParameters:vector <int>Returns:intMethod signature:int solve(vector <int> scores)(be sure your method is public)Notes
-In your solution, the number of nodes N in your graph can be determined as one plus the length ofscores.-In your graph, there must be at most one edge connecting any pair of nodes, and an edge cannot connect a node with itself.Constraints
-scores will contain between 1 and 50 elements, inclusive.-Each element in scores will be between 0 and 10,000, inclusive.Examples
0){1, 3, 0}
Returns: 8
As scores contains 3 elements, we are building a graph with N=4 nodes. Nodes of degree 1 have score 1, nodes of degree 2 have score 3, and nodes of degree 3 have score 0.
One possible graph with the highest score looks as follows:
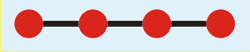
In this graph the degrees of the nodes are 1, 2, 2, 1, respectively. The sum of their scores is 1+3+3+1 = 8.
1){0, 0, 0, 10}
Returns: 10One possible solution for this test case is:
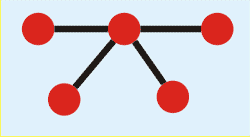
{1, 2, 3, 4, 5, 6}
Returns: 123)
{5, 0, 0}
Returns: 154)
{1, 3, 2, 5, 3, 7, 5}
Returns: 20
I-Forgot-The-Name Lemma: The total degree of all nodes in a tree with N nodes is 2*N - 2.
To see this, it has N-1 edges. Each edge has 2 adjacent nodes. So, each edge adds exactly 2 to the sum of all degrees of the nodes in the graph. Hence, the total degree is 2 * (number of edges) = 2 * (N-1) = 2*N - 2.
Degree Bound Lemma:The degree of each node in a tree is at least 1 and at most N-1
#include<stdio.h>#include<string.h>#include<vector>using namespace std;int dp[150][150];//dp[i][j]表示用i个节点产生度为j的最大值class P8XGraphBuilder{public:int Max(int a,int b){if(a<b)return b;return a;}int solve(vector <int> scores){int i,j,k;int n=scores.size();memset(dp,-1,sizeof(dp));dp[0][0]=0;for(i=1;i<=n+1;i++)for(j=0;j<=2*n;j++)if(dp[i-1][j]!=-1){for(k=1;k<=n&& j+k<=2*n;k++){dp[i][j+k]=Max(dp[i][j+k],dp[i-1][j]+scores[k-1]);}}return dp[n+1][2*n];}};
- SRM527 275 DP
- Topcoder.SRM527.Div1.T2
- srm527 T1 && T2
- dp
- dp
- dp
- 【DP】
- dp
- dp
- DP
- DP
- DP
- DP
- DP
- dp
- DP
- dp
- DP
- 对滚动区域QScrollArea用法的摸索(2)
- 在Android上实现多格式多媒体播放器的几种方式
- DNS 报文格式
- usb cdrom support on USB OHCI
- php fckeditor 上传文件(或图片)中文显示为乱码的解决方法(两种方法)
- SRM527 275 DP
- 设计模式之03抽象工厂模式(笔记)
- JAVA类库分析之Vector
- 一些常用的字符转换
- poj 2488( 搜索 )
- Harmonic map
- JVM性能调优
- HashMap,LinkHashMap和TreeMap的区别及适用范围简单记录
- git ssh远程登录