No. 17 - Queue Implemented with Two Stacks
来源:互联网 发布:网络爬虫书籍推荐 编辑:程序博客网 时间:2024/04/30 15:47
No. 17 - Queue Implemented with Two Stacks
Problem: Implement a queue with two stacks. The class for queues is declared in C++ as below. Please implement two functions: appendTail to append an element into tail of a queue, anddeleteHead to delete an element from head of a queue.
template <typename T> class CQueue
{
public:
CQueue(void);
~CQueue(void);
void appendTail(const T& node);
T deleteHead();
private:
stack<T> stack1;
stack<T> stack2;
};
Analysis: According to declaration above, a queue contains two stacks stack1 and stack2. Therefore, it is required to implement a queue which follows the rule “First In First Out” with two stacks which follow the rule of “First In Last Out”.
We analyze the process to add and delete some elements via some examples. Firstly en element a is inserted. Let us push it into stack1. There is an element {a} in stack1and stack2 is empty. We continue to add two more elements b and c (push them into stack1 too). There are three elements {a, b, c} in stack1 now, where c is on its top, and stack2 is still empty (as shown in Figure 1-a).
We then have a try to delete an element from a queue. According to the rule “First in First out”, the first element to be deleted is a since it is added before b and c. The element a is stored in tostack1, and it is not on the top of stack. Therefore, we cannot pop it directly. We can notice thatstack2 has not been used, so it is the time for us to utilize it. If we pop elements from stack1and push them into stack2 one by one, the order of elements in stack2 is reverse to the order instack1. After three popping and pushing operations, stack1 becomes empty and there are three elements {c, b, a} in stack2. The element a can be popped out now since it is on the top ofstack2. Now there are two elements left {c, b} in stack2 and b is on its top (as shown in Figure 1-b).
How about to continue deleting more elements from the tail of queue? The element b is inserted into queue before c, so it should be deleted when there are two elements b and c left in queue. It can be popped out since it is on the top of stack2. After the popping operation, stack1 remains empty and there is only an element c in stack2 (as shown in Figure 1-c).
It is time to summarize the steps to delete an element from a queue: The top of stack2 can be popped out since it is the first element inserted into queue when stack2 is not empty. Whenstack2 is empty, we pop all elements from stack1 and push them into stack2 one by one. The first element in a queue is pushed into the bottom of stack1. It can be popped out directly after popping and pushing operations since it is on the top of stack2.
Let us insert another element d. How about to push it into stack1 (as shown in Figure1-d)? When we continue to delete the top of stack2, which is element c, can be popped because it is not empty (as shown in Figure 1-d). The element c is indeed inserted into queue before the element d, so it is a reasonable operation to delete c before d. The final status of the queue is shown as Figure 1-e.
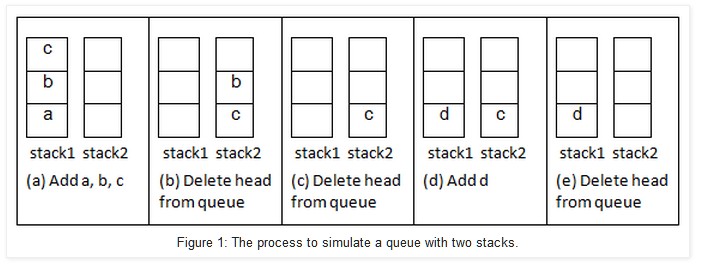
We can write code after we get clear ideas about the process to insert and delete elements. Some sample code is shown below:
template<typename T> void CQueue<T>::appendTail(const T& element)
{
stack1.push(element);
}
template<typename T> T CQueue<T>::deleteHead()
{
if(stack2.size()<= 0)
{
while(stack1.size()>0)
{
T& data = stack1.top();
stack1.pop();
stack2.push(data);
}
}
if(stack2.size() == 0)
throw new exception("queue is empty");
T head = stack2.top();
stack2.pop();
return head;
}The author Harry He owns all the rights of this post. If you are going to use part of or the whole of this ariticle in your blog or webpages, please add a reference to http://codercareer.blogspot.com/. If you are going to use it in your books, please contact me (zhedahht@gmail.com) . Thanks.
- No. 17 - Queue Implemented with Two Stacks
- Implement Queue with Two Stacks
- Implement Queue with two Stacks Java
- Queue Implemented with LinkedList
- implement a queue by using two stacks [No. 31]
- implement-queue-by-two-stacks
- Implement Queue by Two Stacks
- Implement Queue by Two Stacks
- Implement Queue by Two Stacks
- Implement Queue by Two Stacks
- implement a queue by using two stacks
- [刷题]Implement Queue by Two Stacks
- lintcode: Implement Queue by Two Stacks
- #40 Implement Queue by Two Stacks
- [Lintcode]Implement Queue by Two Stacks
- Implement Queue by Two Stacks 解题报告
- Lintcode 40:Implement Queue by Two Stacks
- Leetcode NO.232 Implement Queue using Stacks
- 串行端口地址及寄存器功能详细资料
- No. 16 - Maximal Length of Incremental Subsequences
- 实习总结(四)---OA办公业务资源系统需求规格说明书
- 腾讯面试题—附答案
- DirectX游戏编程入门——第二部分(游戏编程工具箱) ——精灵编程之简介
- No. 17 - Queue Implemented with Two Stacks
- No. 18 - Reverse a Linked List
- No. 19 - Left Rotation of String
- No. 20 - Number of 1 in a Binary
- Linux学习笔记(一)
- lq51--基于8051的嵌入式实时操作系统
- hdu1068
- ObjectInputStream 和 ObjectOutputStream(对象的序列化、持久化)
- VC 多线程编程