TOPCODER/SRM 566 DIVII(250、500、1000题)(1000PT暂未附上代码)
来源:互联网 发布:手机淘宝如何刷新秒杀 编辑:程序博客网 时间:2024/05/01 10:51
Problem Statement
Percy has just received a new game called Penguin Tiles. The game is played on a rectangular grid.
Except for one square, each square of the grid contains a tile with a part of an image of a penguin.
The one remaining square is empty, and it is called the open square. The player is allowed to
slide one of the tiles adjacent to the open square onto the open square. After several moves
the tile game is supposed to form a picture with the bottom right corner containing the open square.
Percy's version of Penguin Tiles is a misprint. Instead of each tile containing a different part of a
penguin all tiles contain an image of the same penguin. In other words each pair of tiles in
Percy's Penguin Tiles is indistinguishable.
Percy has decided to play with the game anyway but instead of moving just one tile at a time
he has decided to move several tiles at once. In one move, Percy can either move some c
onsecutive vertical tiles one square vertically, or some consecutive horizontal tiles one square
horizontally. Of course, one of the tiles has to be moved onto the open square. (In other words,
instead of moving several tiles one at a time, Percy may move them all at once, if they all lie in
the same row or in the same column.)
You are given a vector <string> tiles representing the game. The j-th character of the i-th element
oftiles is 'P' if the square at row i, column j contains a tile, and it is '.' (a period) for the open square.
Return the minimum number of moves to complete Percy's game.
Definition
Class:
PenguinTiles
Method:
minMoves
Parameters:
vector <string>
Returns:
int
Method signature:
int minMoves(vector <string> tiles)
(be sure your method is public)
Constraints
-
tiles will contain between 2 and 50 elements, inclusive.
-
Each element of tiles will contain between 2 and 50 characters, inclusive.
-
Each element of tiles will contain the same number of characters.
-
Each character of each element of tiles will be either 'P' or '.'.
-
tiles will contain exactly 1 occurrence of the character '.'.
Examples
0)
{"PP", "P."}
Returns: 0
The open tile is already in the bottom right corner.
1)
{"PPPPPPPP", ".PPPPPPP"}
Returns: 1
2)
{"PPP", "P.P", "PPP"}
Returns: 2
3)
{"P.", "PP", "PP", "PP"}
Returns: 1
4)
{".PPP", "PPPP", "PPPP", "PPPP"}
Returns: 2
#include <deque>
#include <vector>
#include <algorithm>
#include <functional>
#include <iterator>
#include <map>
#include <memory>
#include <numeric>
#include <queue>
#include <set>
#include <utility>
#include <stack>
#include <iostream>
#include <string>
#include <cstdio>
#include <cstdlib>
#include <cmath>
#define PI 3.1415926535898
#define LL long long
using namespace std;
class PenguinTiles
{
public:
int minMoves(vector <string> tiles)
{
int size = tiles.size();
int x=0,y=0;
for(int i=0;i<size;i++)
for(int j=0;j<size;j++)
{
if( tiles[i][j] == '.')
{
x = i;
y = j;
}
}
size--;
if (( x == size )&&( y == size))
return 0;
else
{
if(( x!= size)&&( y != size))
return 2;
else
return 1;
}
}
};
500pt:
Problem Statement
Penguin Pals is a match making service that matches penguins to new friends, using the following
procedure:
1.ch penguin is asked a single question: "Do you prefer the color blue, or the color red?"
2.All penguins are arranged so that they stand on a circle, equally spaced.
3.The organizers draw some straight lines, connecting some pairs of penguins.
4.Each penguin may only be connected to at most one other penguin. Two penguins cannot be
connected if they prefer a different color.
5.Each penguin who is connected to some other penguin follows the line to find their match.
The only problem with the above system was that it allowed penguins to collide if two lines crossed
each other. Therefore, a new additional rule was adopted: no two lines may cross. Penguin Pals now
has some penguins arranged on a circle (after step 2 of the above procedure). They need to know the
maximum number of pairs of penguins they can create.
You are given a string colors whose i-th character represents the prefered color of the i-th penguin
(0-based index) in the circular arrangement. The i-th character is 'R' if the i-th penguin prefers red
and 'B' if the i-th penguin prefers blue. Return the maximum number of matched pairs that can be formed.
Definition
Class:PenguinPalsMethod:findMaximumMatchingParameters:stringReturns:intMethod signature:int findMaximumMatching(string colors)(be sure your method is public)Constraints
-colors will contain between 1 and 50 characters, inclusive.-Each character of colors will be either 'R' or 'B'.Examples
0)"RRBRBRBB"
Returns: 3In this picture the penguins have been colored in their preferred color.
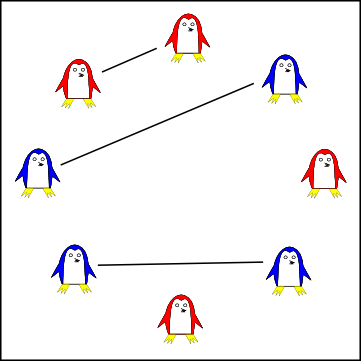
"RRRR"
Returns: 22)
"BBBBB"
Returns: 23)
"RBRBRBRBR"
Returns: 44)
"RRRBRBRBRBRB"
Returns: 55)
"R"
Returns: 06)
"RBRRBBRB"
Returns: 37)
"RBRBBRBRB"
Returns: 4
#include <deque>
#include <vector>
#include <algorithm>
#include <functional>
#include <iterator>
#include <map>
#include <memory>
#include <numeric>
#include <queue>
#include <set>
#include <utility>
#include <stack>
#include <iostream>
#include <string>
#include <cstdio>
#include <cstdlib>
#include <cmath>
#define PI 3.1415926535898
#define LL long long
using namespace std;
class PenguinPals
{
public:int findMaximumMatching(string colors)
{
string clrs = colors;
int length = 0;
int cnt = 0;
int tail = 0;;
int i=0;;
for(i=0;i<colors.size()-1;i++)
{
if( colors[i] == colors[i+1] )
{
i++;
cnt++;
}
else
{
clrs[length] = colors[i];
length++;
}
}
if( colors[i] != colors[i-1])
{
clrs[length] = colors[i];
length++;
}
tail = length-1;
for(i=0;i<length-1;i++)
{
if( i >= tail)
break;
else
{
if( clrs[i] == clrs[tail])
{
cnt++;
tail--;
}
}
}
return cnt;
}
};
1000pt:
Problem Statement
Paco collects penguins. His penguins like to play and run around on the ice where he lives. In order to
keep his penguins safe he decided to construct a single fence enclosure to keep danger out.
Paco's penguins have fallen asleep. Acting quickly Paco placed numPosts posts in a circular configuration
on the ice. Each post is placedradius meters from location (0,0). The posts are equally spaced with the
first post being placed at location (radius, 0).
To construct the fencing, Paco will connect some pairs of fence posts using straight fences. No two s
egments of the fence are allowed to cross. In the resulting fencing, each fence post will either be
unused, or it will be connected to exactly two other fence posts. In order to minimize the damage his
penguins cause to the ice, he would like to minimize the area enclosed by the fence.
In order to keep all his penguins happy Paco would like to have all his penguins in the one single
enclosure. Two penguins are in the same enclosure if it is possible to walk from one penguin to the
other without crossing a fence.
You are given two ints numPosts and radius. You are also given two int[]sx andy representing the
(x,y) location of each of the sleeping penguins. Each penguin is small enough to be considered a
point. Compute an return the smallest area of an enclosure that can contain all the penguins.
If it is not possible to enclose all the penguins return -1.
Definition
Class:FencingPenguinsEasyMethod:calculateMinAreaParameters:int, int, int[], int[]Returns:doubleMethod signature:double calculateMinArea(int numPosts, int radius, int[] x, int[] y)(be sure your method is public)Constraints
-numPosts will be between 3 and 222, inclusive.-radius will be between 5 and 100,000, inclusive.-x will contain between 1 and 50 elements, inclusive.-y will contain the same number of elements as x.-Each element in x will be between -100,000 and 100,000, inclusive.-Each element in y will be between -100,000 and 100,000, inclusive.-No penguin will come within 10^-6 of any potential fencing.-No two penguins will occupy the same location.Examples
0)3
5
{-1}
{0}
Returns: 32.47595264191645There is only one possible enclosure that uses all three fence posts.1)
30
5
{6}
{0}
Returns: -1.02)
4
5
{2}
{1}
Returns: 25.0
If the posts are numbered starting from 0 at (radius, 0) and increasing in the
counter-clockwise direction. The resulting possible answers would be:
(0,1,2)
(0,1,3)
Both enclosures cover the same area.
4
5
{2,-2}
{1,-1}
Returns: 50.04)
8
5
{8}
{8}
Returns: -1.0
It is not possible to enclose this penguin.
Please note that while a penguin can't be located close to a potential fencing (see constraints),
it can be located close to or exactly on a straight line passing through any pair of fence posts.
5)7
10
{9}
{1}
Returns: 29.4367526377118056)
30
800
{8,2,100,120,52,67,19,-36}
{-19,12,88,-22,53,66,-140,99}
Returns: 384778.74757953023
- TOPCODER/SRM 566 DIVII(250、500、1000题)(1000PT暂未附上代码)
- Topcoder SRM 544 1000pt
- Topcoder SRM 569 1000pt
- TOPCODER/SRM565 DIVII 250、500pt(500pt无递归解法)
- TOPCODE/SRM567 DIVII 250、500PT
- SRM 596 1000pt
- SRM 497(DIVII) 250 Filtering
- SRM 497(DIVII) 500 PermutationSignature
- Topcoder SRM 566 DIV2 1000 FencingPenguinsEasy
- Topcoder SRM 709 DIV 2 250pt Robofactory solution
- Topcoder SRM 709 DIV 2 500pt Permatchd2 solution
- SRM 431 div2 1000pt(好题,数学题)
- SRM 440 DIVII 250中文翻译及源码(C#)
- SRM 441 DIVII 250概述及源码(C#)
- SRM 441 DIVII 250概述及源码(C语言版)
- SRM 440 DIVII 500中文翻译及思路(C#)
- Topcoder SRM536 div2 1000pt
- TopCoder SRM 474 DIV1 1000
- Intel处理器的保护模式-分段和分页
- 用动态链接库做系统测试的插件
- POJ1001 Exponenentiation
- 浅谈C/C++中运算符的优先级、运算符的结合性以及操作数的求值顺序
- windows gdi - 保存bitmap
- TOPCODER/SRM 566 DIVII(250、500、1000题)(1000PT暂未附上代码)
- 最长公共子序列问题(动态规划法)
- 2012总结--第6篇--装备篇
- ModelSim PE, DE, SE, XE
- [细节决定B度]之二分搜索也不易啊
- 【解决】EXP-00056 ORA-06550 PLS-00302 ORA-06550错误
- C++博客十八罗汉造像
- [体检]悲从中来,伤不起
- sql写文件