CoreGraphics 学习摘记之 Transforms
来源:互联网 发布:java代码审查表 编辑:程序博客网 时间:2024/05/18 03:23
The Quartz 2D drawing model defines two completely separate coordinate spaces: user space, which represents the document page, and device space, which represents the native resolution of a device. User space coordinates are floating-point numbers that are unrelated to the resolution of pixels in device space. When you want to print or display your document, Quartz maps user space coordinates to device space coordinates.
You can modify the default user space by operating on the current transformation matrix, or CTM.
Modifying the Current Transformation Matrix
Before you transform the CTM, you need to save the graphics state so that you can restore it after drawing.Translation moves the origin of the coordinate space by the amount you specify for the x and y axes. You call the function CGContextTranslateCTM
to modify the x and y coordinates of each point by a specified amount. Figure 5-3 shows an image translated by 100 units in the x-axis and 50 units in the y-axis, using the following line of code:
CGContextTranslateCTM (myContext, 100, 50);
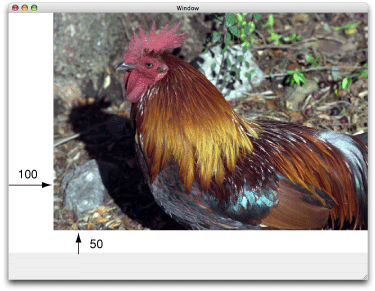
Rotation moves the coordinate space by the angle you specify. You call the function CGContextRotateCTM
to specify the rotation angle, in radians. Figure 5-4 shows an image rotated by –45 degrees about the origin, which is the lower left of the window, using the following line of code:
CGContextRotateCTM (myContext, radians(–45.));
The image is clipped because the rotation moved part of the image to a location outside the context. You need to specify the rotation angle in radians.
It’s useful to write a radians routine if you plan to perform many rotations.
#include <math.h>
static inline double radians (double degrees) {return degrees * M_PI/180;}
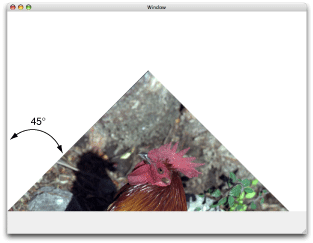
Scaling changes the scale of the coordinate space by the x and y factors you specify, effectively stretching or shrinking the image. The magnitude of the x and y factors governs whether the new coordinates are larger or smaller than the original. In addition, by making the x factor negative, you can flip the coordinates along the x-axis; similarly, you can flip coordinates horizontally, along the y-axis, by making the y factor negative. You call the function CGContextScaleCTM
to specify the x and y scaling factors. Figure 5-5 shows an image whose x values are scaled by .5 and whose y values are scaled by .75, using the following line of code:
CGContextScaleCTM (myContext, .5, .75);
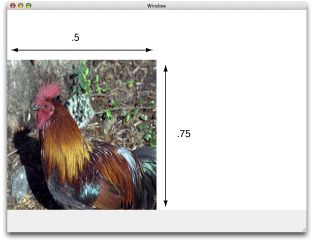
Concatenation combines two matrices by multiplying them together. You can concatenate several matrices to form a single matrix that contains the cumulative effects of the matrices. You call the function CGContextConcatCTM
to combine the CTM with an affine transform. Affine transforms, and the functions that create them, are discussed in “Creating Affine Transforms.”
Another way to achieve a cumulative effect is to perform two or more transformations without restoring the graphics state between transformation calls. Figure 5-6 shows an image that results from translating an image and then rotating it, using the following lines of code:
CGContextTranslateCTM (myContext, w,h);
CGContextRotateCTM (myContext, radians(-180.));
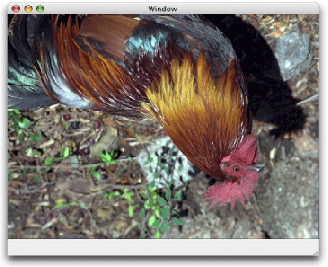
Creating Affine Transforms
The affine transform functions available in Quartz operate on matrices, not on the CTM. You can use these functions to construct a matrix that you later apply to the CTM by calling the functionCGContextConcatCTM
. Function
Use
CGAffineTransformMakeTranslation
To construct a new translation matrix from x and y values that specify how much to move the origin.
CGAffineTransformTranslate
To apply a translation operation to an existing affine transform.
CGAffineTransformMakeRotation
To construct a new rotation matrix from a value that specifies in radians how much to rotate the coordinate system.
CGAffineTransformRotate
To apply a rotation operation to an existing affine transform.
CGAffineTransformMakeScale
To construct a new scaling matrix from x and y values that specify how much to stretch or shrink coordinates.
CGAffineTransformScale
To apply a scaling operation to an existing affine transform.
Quartz also provides an affine transform function that inverts a matrix, CGAffineTransformInvert
. Inversion is generally used to provide reverse transformation of points within transformed objects. Inversion can be useful when you need to recover a value that has been transformed by a matrix: Invert the matrix, and multiply the value by the inverted matrix, and the result is the original value. You usually don’t need to invert transforms because you can reverse the effects of transforming the CTM by saving and restoring the graphics state.
In some situations you might not want to transform the entire space, but just a point or a size. You operate on a CGPoint
structure by calling the function CGPointApplyAffineTransform
. You operate on a CGSize
structure by calling the function CGSizeApplyAffineTransform
. You can operate on a CGRect
structure by calling the function CGRectApplyAffineTransform
.
Evaluating Affine Transforms
You can determine whether one affine transform is equal to another by calling the functionCGAffineTransformEqualToTransform
. The function CGAffineTransformIsIdentity
is a useful function for checking whether a transform is the identity transform. The identity transform performs no translation, scaling, or rotation. Getting the User to Device Space Transform
Quartz provides a number of convenience functions to transform the following geometries between user space and device space. You might find these functions easier to use than applying the affine transform returned from the function CGContextGetUserSpaceToDeviceSpaceTransform
.
Points. The functions
CGContextConvertPointToDeviceSpace
andCGContextConvertPointToUserSpace
transform aCGPoint
data type from one space to the other.Sizes. The functions
CGContextConvertSizeToDeviceSpace
andCGContextConvertSizeToUserSpace
transform aCGSize
data type from one space to the other.Rectangles. The functions
CGContextConvertRectToDeviceSpace
andCGContextConvertRectToUserSpace
transform aCGRect
data type from one space to the other.
- CoreGraphics 学习摘记之 Transforms
- CoreGraphics 学习摘记之 Paths
- CoreGraphics 学习摘记之 Shadows
- CoreGraphics 学习摘记之 Gradients
- CoreGraphics 学习摘记 之 Color and Color Spaces
- iOS学习笔记之CoreGraphics框架
- iOS学习笔记之CoreGraphics框架
- iOS学习笔记之CoreGraphics框架
- CoreGraphics简单绘图学习
- iOS之CoreGraphics
- 学习摘记
- Transforms
- CoreGraphics
- CoreGraphics
- CoreGraphics
- CoreGraphics
- CoreGraphics之CGContextSaveGState与UIGraphicsPushContext
- perl学习摘记4
- 数据库连接方式汇总
- Android Drawable 转化为 Bitmap
- cygwin显示中文
- 单片机中断中 void time0() interrupt 1 using 1中的interrupt 1 和 using 1各代表什么意思
- solr-用mmseg4j配置同义词索引和检索(IKanlyzer需要修改源码适应solr接口才能使用同义词功能)
- CoreGraphics 学习摘记之 Transforms
- 当ie地址栏参数为中文时,传给php后端时乱码的解决办法
- Android 笔记 ---- PopupWindow中有EditText,EditText被输入法盖住
- C# 中使用Linq和Lambda表达式对List<T>进行排序
- cocos2d-x 3.0 final 在android 中添加多盟插屏广告
- sift的opencv简易实现
- maven pom 元素 详解
- nginx搭建支持http和rtmp协议的流媒体服务器之一
- C语言概述