模版解析
来源:互联网 发布:电脑重装数据恢复 编辑:程序博客网 时间:2024/05/22 06:38
1. [图片] tpl.png

2. [图片] conf.png
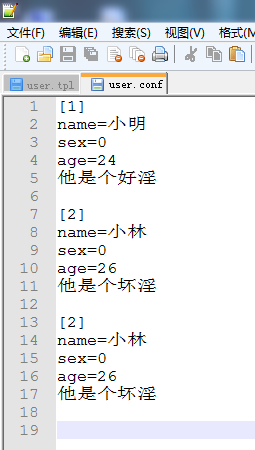
3. [代码][Java]代码
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
226
227
228
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
264
265
import
java.io.BufferedReader;
import
java.io.File;
import
java.io.FileOutputStream;
import
java.io.FileReader;
import
java.io.LineNumberReader;
import
java.io.OutputStreamWriter;
import
java.util.HashMap;
import
java.util.Map;
import
java.util.regex.Matcher;
import
java.util.regex.Pattern;
import
javax.servlet.http.HttpServletRequest;
public
class
TemplateEngine{
private
static
HttpServletRequest request;
// servlet参数,用于获取WebContent目录下模板路径
private
static
String confPath;
// 配置文件,包括完整绝对路径
private
static
String enter;
// 换行符
// 构造函数,初始化数据
public
TemplateEngine(HttpServletRequest request, String confPath){
TemplateEngine.request = request;
TemplateEngine.confPath = confPath;
TemplateEngine.enter = System.getProperty(
"line.separator"
);
}
/**
* 测试
*
* @param args
*/
public
static
void
main(String[] args){
Map<String, String> user =
new
HashMap<String, String>();
user.put(
"id"
,
"2"
);
user.put(
"name"
,
"小林"
);
user.put(
"sex"
,
"0"
);
user.put(
"age"
,
"26"
);
user.put(
"description"
,
"他是个坏淫"
);
// 设置换行符
setEnter(System.getProperty(
"line.separator"
));
// 读取模板文件
String template = readTemplate(
"f://user.tpl"
);
// 替换模板变量
String dataString = replaceArgs(template, user);
// 追加写入配置文件
writeConf(
"f://user.conf"
, dataString,
true
);
// 测试删除对象
TemplateEngine te =
new
TemplateEngine(
null
,
"f://user.conf"
);
te.deleteObject(
"1"
);
}
/**
* 读取模板文件(文件名跟配置文件相同,后缀为.tpl)
*
* @return
*/
public
static
String readTemplate(String tplPath){
StringBuffer sb =
new
StringBuffer();
try
{
FileReader fr =
new
FileReader(tplPath);
BufferedReader br =
new
BufferedReader(fr);
String line =
""
;
while
((line = br.readLine()) !=
null
){
sb.append(line + enter);
// 加一个换行符
}
br.close();
fr.close();
}
catch
(Exception e){
e.printStackTrace();
}
return
sb.toString();
}
/**
* 替换模板变量
*
* @param template
* @param data
* @return
*/
public
static
String replaceArgs(String template, Map<String, String> data){
// sb用来存储替换过的内容,它会把多次处理过的字符串按源字符串序 存储起来。
StringBuffer sb =
new
StringBuffer();
try
{
Pattern pattern = Pattern.compile(
"\\$\\{(.+?)\\}"
);
Matcher matcher = pattern.matcher(template);
while
(matcher.find()){
String name = matcher.group(
1
);
// 键名
String value = (String)data.get(name);
// 键值
if
(value ==
null
){
value =
""
;
}
else
{
value = value.replaceAll(
"\\$"
,
"\\\\\\$"
);
}
matcher.appendReplacement(sb, value);
}
// 最后还得要把尾串接到已替换的内容后面去,这里尾串为“,欢迎下次光临!”
matcher.appendTail(sb);
}
catch
(Exception e){
e.printStackTrace();
}
return
sb.toString() + enter;
//加一个空行(结束行)
}
/**
* 读取配置文件
*
* @param confPath
*/
public
static
String readConf(String confPath){
StringBuffer sb =
new
StringBuffer();
try
{
FileReader fr =
new
FileReader(confPath);
BufferedReader br =
new
BufferedReader(fr);
String line =
""
;
while
((line = br.readLine()) !=
null
){
sb.append(line + enter);
}
br.close();
fr.close();
}
catch
(Exception e){
e.printStackTrace();
}
return
sb.toString();
}
/**
* 写入到配置文件
*
* @param confPath
* @param stringData
* @param isAppend 是否追加写入
*/
public
static
void
writeConf(String confPath, String stringData,
boolean
isAppend){
try
{
File f =
new
File(confPath);
if
( !f.exists()){
f.createNewFile();
}
String fileEncode = System.getProperty(
"file.encoding"
);
FileOutputStream fos =
new
FileOutputStream(confPath, isAppend);
OutputStreamWriter osw =
new
OutputStreamWriter(fos, fileEncode);
osw.write(
new
String(stringData.getBytes(), fileEncode));
osw.close();
fos.close();
}
catch
(Exception e){
e.printStackTrace();
}
}
/**
* 根据中括号内的ID查询对象
*
* @param id
* @return
*/
public
String getObject(String id){
StringBuffer sb =
new
StringBuffer();
try
{
FileReader fr =
new
FileReader(confPath);
LineNumberReader nr =
new
LineNumberReader(fr);
String line =
""
;
int
startLineNumber = -
1
;
while
((line = nr.readLine()) !=
null
){
// 匹配到开头
if
(line.indexOf(
"["
+ id +
"]"
) >=
0
){
startLineNumber = nr.getLineNumber();
}
if
(startLineNumber != -
1
&& nr.getLineNumber() >= startLineNumber){
sb.append(line + enter);
// 匹配到结束,以换行符结束
if
(line.trim().equals(
""
)){
break
;
}
}
}
nr.close();
fr.close();
}
catch
(Exception e){
e.printStackTrace();
}
return
sb.toString();
}
/**
* 追加写入对象到配置文件
*
* @param data
*/
public
void
addObject(Map<String, String> data){
try
{
// 读取模板文件
String tplName = confPath.substring(confPath.lastIndexOf(File.separator) +
1
, confPath.lastIndexOf(
"."
)) +
".tpl"
;
String tplPath = request.getSession().getServletContext().getRealPath(File.separator +
"template"
+ File.separator + tplName);
String template = readTemplate(tplPath);
// 替换模板变量
String stringData = replaceArgs(template, data);
// 追加写入配置文件
writeConf(confPath, stringData,
true
);
}
catch
(Exception e){
e.printStackTrace();
}
}
/**
* 删除对象(IO没有对文本直接删除的方法,先读出所有内容,过滤删除内容,重新写回文件。)
*
* @param id
* @return
*/
public
void
deleteObject(String id){
// 读取配置文件
String data = readConf(confPath);
// 过滤删除内容
data = data.replace(getObject(id),
""
);
// 重新写回文件
writeConf(confPath, data,
false
);
}
/**
* 修改对象(模板数据并非全是键值对,所以只能先删除再添加)
*
* @param id
* @param data
*/
public
void
updateObject(String id, Map<String, String> data){
// 读取配置文件
String dataString = readConf(confPath);
// 过滤删除内容
dataString = dataString.replace(getObject(id),
""
);
// 读取模板文件
String tplName = confPath.substring(confPath.lastIndexOf(File.separator) +
1
, confPath.lastIndexOf(
"."
)) +
".tpl"
;
String tplPath = request.getSession().getServletContext().getRealPath(File.separator +
"template"
+ File.separator + tplName);
String template = readTemplate(tplPath);
// 替换模板变量
String newData = replaceArgs(template, data);
// 末尾追加新对象
writeConf(confPath, dataString + newData,
false
);
}
public
static
HttpServletRequest getRequest(){
return
request;
}
public
static
void
setRequest(HttpServletRequest request){
TemplateEngine.request = request;
}
public
static
String getConfPath(){
return
confPath;
}
public
static
void
setConfPath(String confPath){
TemplateEngine.confPath = confPath;
}
public
static
String getEnter(){
return
enter;
}
public
static
void
setEnter(String enter){
TemplateEngine.enter = enter;
}
}
0 0
- 模版解析
- Discuz模版解析
- js模版深度解析
- 深度解析C++模版
- C++模版深度解析
- C++模版深度解析
- KMP模版及解析
- C++模版深度解析
- JS模版解析
- C++模版深度解析
- 深度解析C++模版
- 深度解析C++模版
- ECshop前台模版详细解析
- 字典树 (解析加模版)
- 字典树 (解析加模版)
- php 正则实例 模版解析
- Halcon模版匹配算子解析
- LCA转RMQ 模版及解析 + LCA倍增法模版
- CSS编码规范(二)
- Charm Bracelet poj 3624 (01) 背包问题 c++
- 使用归档来创建对象的深复制
- socket套接字TCP API说明
- hibernate-Query.list()与Query.iterate()比较
- 模版解析
- 对一段Oracle GoldenGate (OGG) 传输进程日志(.rpt文件)的解释
- UI05-学习笔记
- 分数加减法 poj 3979
- Codeforces Round #259 (Div. 2) C Little Pony and Expected Maximum
- 自定义类的copy实现(实现NSCopying协议)
- 铺尔铺客户端打包失败Failed to export application 之完美解决办法。
- Java Web知识
- J2EE相关技术