UE4学习笔记4th
来源:互联网 发布:广州男装网络批发 编辑:程序博客网 时间:2024/04/30 06:27
在这一节中,我们来处理对输入的响应
双击打开MyPawn在vs中进行编辑。
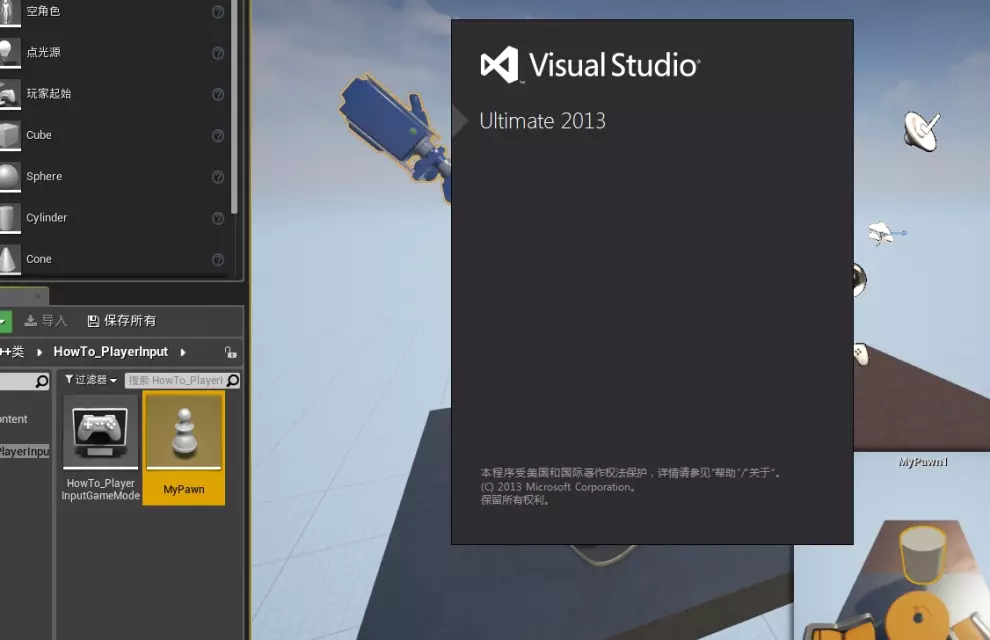
在MypPawn.h中添加以下定义:
现在我们已经完成了对输入函数的定义,接下来要将它们进行绑定
在AMyPawn::SetupPlayerInputComponent函数中输入以下代码:
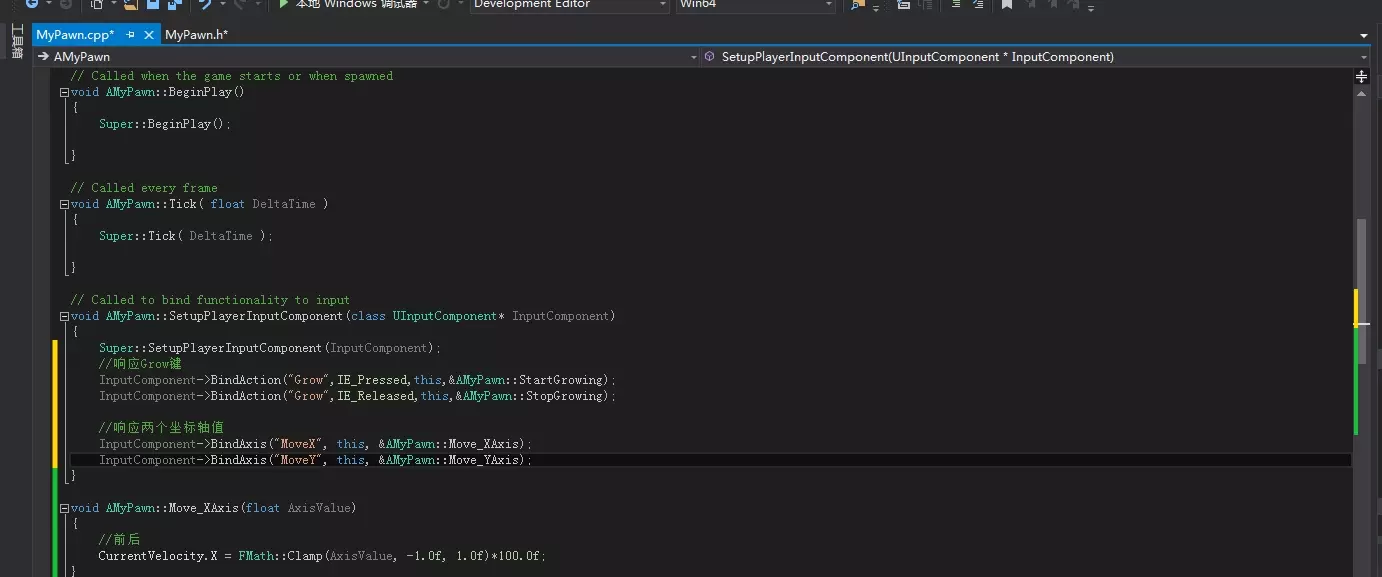
现在我完成了对输入的绑定,接下来我要将它们在视口中体现出来。
在AMyPawn::Tick中添加如下代码:
编译
编译完代码后,在编辑器中按下 Play ,应该可以使用WASD键来控制 Pawn ,而且我们应该可以通过按住空格键来使之增大,并在松开后看着其缩小。
下面是完整的代码:
MyPawn.h
MyPawn.cpp
双击打开MyPawn在vs中进行编辑。
在MypPawn.h中添加以下定义:
void Move_XAxis(float AxisValue);
void Move_YAxis(float AxisValue);
void StartGrowing();
void StopGrowing();
//输入变量
FVector CurrentVelocity;
bool bGrowing;
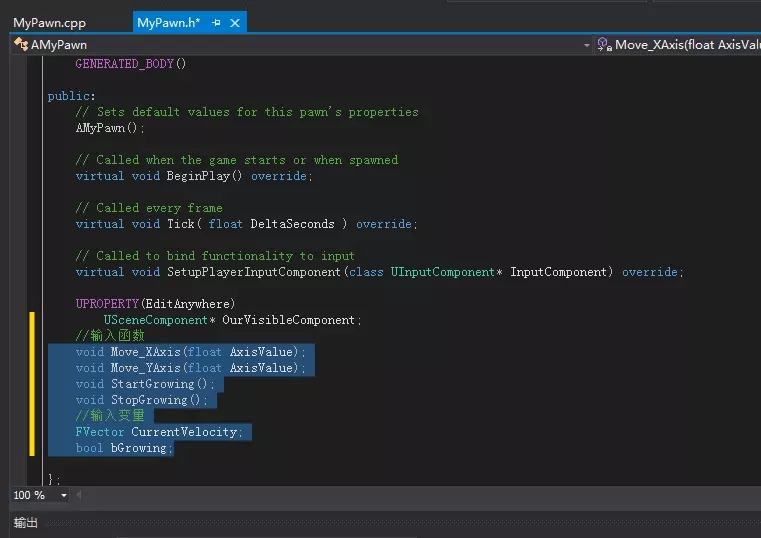
前两个用于前后左右方向上的处理,后两个处理跳跃。
它们在运行时,会改变变量中的值,从而确定在游戏中应执行的操作。
切换到MyPawn.cpp,对这四个函数进行编程。
在最下方输入
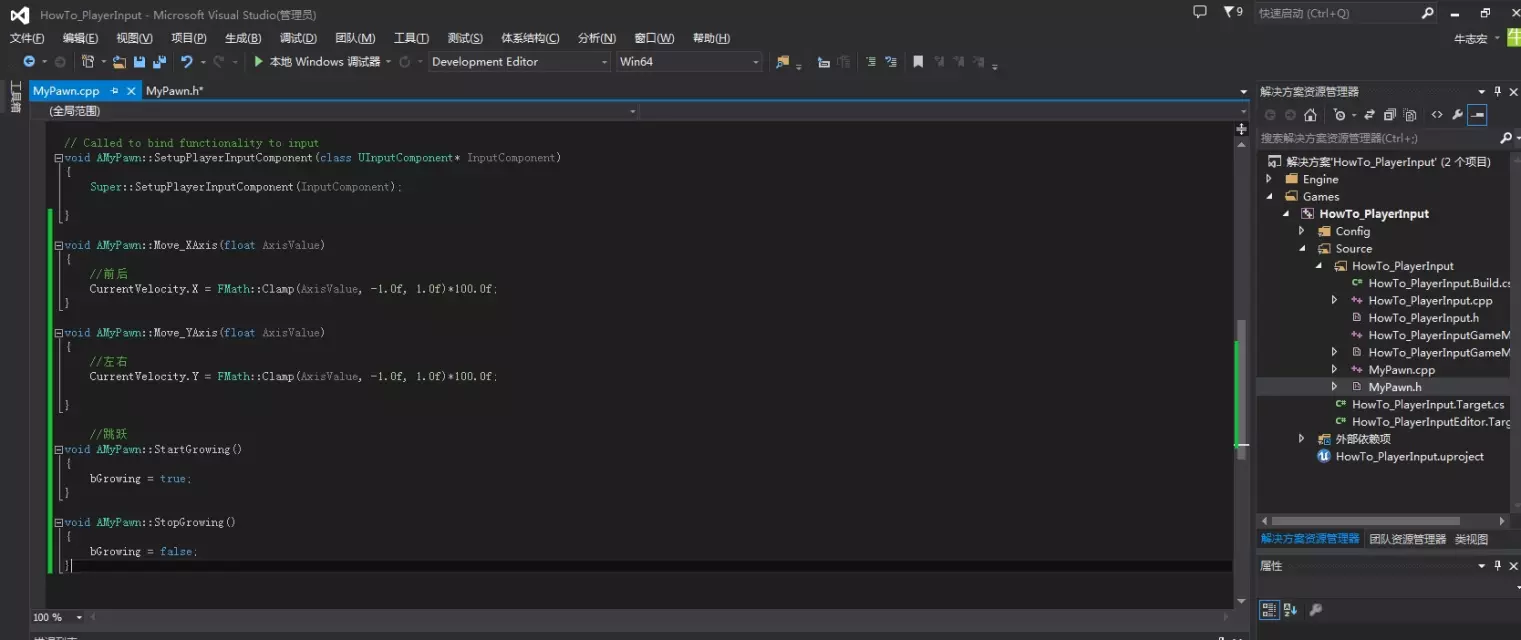
前两个用于前后左右方向上的处理,后两个处理跳跃。
它们在运行时,会改变变量中的值,从而确定在游戏中应执行的操作。
切换到MyPawn.cpp,对这四个函数进行编程。
在最下方输入
void AMyPawn::Move_XAxis(float AxisValue)
{
//前后
CurrentVelocity.X = FMath::Clamp(AxisValue, -1.0f, 1.0f)*100.0f;
}
void AMyPawn::Move_YAxis(float AxisValue)
{
//左右
CurrentVelocity.Y = FMath::Clamp(AxisValue, -1.0f, 1.0f)*100.0f;
}
//跳跃
void AMyPawn::StartGrowing()
{
bGrowing = true;
}
void AMyPawn::StopGrowing()
{
bGrowing = false;
}
现在我们已经完成了对输入函数的定义,接下来要将它们进行绑定
在AMyPawn::SetupPlayerInputComponent函数中输入以下代码:
//响应Grow键
InputComponent->BindAction("Grow",IE_Pressed,this,&AMyPawn::StartGrowing);
InputComponent->BindAction("Grow",IE_Released,this,&AMyPawn::StopGrowing);
//响应两个坐标轴值
InputComponent->BindAxis("MoveX", this, &AMyPawn::Move_XAxis);
InputComponent->BindAxis("MoveY", this, &AMyPawn::Move_YAxis);
现在我完成了对输入的绑定,接下来我要将它们在视口中体现出来。
在AMyPawn::Tick中添加如下代码:
//基于Grow操作来处理增长和收缩
{
float CurrentScale = OurVisibleComponent->GetComponentScale().X;
if (bGrowing)
{
//在一秒内增长到两倍大小
CurrentScale += DeltaTime;
}
else
{
//随着增长收缩到一半
CurrentScale -= (DeltaTime*0.5f);
}
//确认不小于初始大小、增长前的两倍大小
CurrentScale = FMath::Clamp(CurrentScale, 1.0f, 2.0f);
OurVisibleComponent->SetWorldScale3D(FVector(CurrentScale));
}
//基于MoveX和MoveY来处理移动
{
if (!CurrentVelocity.IsZero())
{
FVector NewLoaction = GetActorLocation() + (CurrentVelocity*DeltaTime);
SetActorLocation(NewLoaction);
}
}
完成后编译,效果如下
完成后编译,效果如下
编译完代码后,在编辑器中按下 Play ,应该可以使用WASD键来控制 Pawn ,而且我们应该可以通过按住空格键来使之增大,并在松开后看着其缩小。
下面是完整的代码:
MyPawn.h
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "GameFramework/Pawn.h"
#include "MyPawn.generated.h"
UCLASS()
class HOWTO_PLAYERINPUT_API AMyPawn : public APawn
{
GENERATED_BODY()
public:
// Sets default values for this pawn's properties
AMyPawn();
// Called when the game starts or when spawned
virtual void BeginPlay() override;
// Called every frame
virtual void Tick( float DeltaSeconds ) override;
// Called to bind functionality to input
virtual void SetupPlayerInputComponent(class UInputComponent* InputComponent) override;
UPROPERTY(EditAnywhere)
USceneComponent* OurVisibleComponent;
//输入函数
void Move_XAxis(float AxisValue);
void Move_YAxis(float AxisValue);
void StartGrowing();
void StopGrowing();
//输入变量
FVector CurrentVelocity;
bool bGrowing;
};
MyPawn.cpp
// Fill out your copyright notice in the Description page of Project Settings.
#include "HowTo_PlayerInput.h"
#include "MyPawn.h"
// Sets default values
AMyPawn::AMyPawn()
{
// Set this pawn to call Tick() every frame. You can turn this off to improve performance if you don't need it.
PrimaryActorTick.bCanEverTick = true;
//设置为玩家控制
AutoPossessPlayer = EAutoReceiveInput::Player0;
//添加一个可以添加对象的空根组件
RootComponent = CreateDefaultSubobject<USceneComponent>(TEXT("RootComponent"));
//创建相机和可见项目
UCameraComponent* OurCamera = CreateDefaultSubobject<UCameraComponent>(TEXT("OurCamera"));
OurVisibleComponent = CreateDefaultSubobject<UStaticMeshComponent>(TEXT("OurVisibleComponent"));
//附加相机和可见对象到根组建,偏移并旋转相机。
OurCamera->AttachTo(RootComponent);
OurCamera->SetRelativeLocation(FVector(-250.0f, 0.0f, 250.0f));
OurCamera->SetRelativeRotation(FRotator(-45.0f, 0.0f, 0.0f));
OurVisibleComponent->AttachTo(RootComponent);
}
// Called when the game starts or when spawned
void AMyPawn::BeginPlay()
{
Super::BeginPlay();
}
// Called every frame
void AMyPawn::Tick( float DeltaTime )
{
Super::Tick(DeltaTime);
//基于Grow操作来处理增长和收缩
{
float CurrentScale = OurVisibleComponent->GetComponentScale().X;
if (bGrowing)
{
//在一秒内增长到两倍大小
CurrentScale += DeltaTime;
}
else
{
//随着增长收缩到一半
CurrentScale -= (DeltaTime*0.5f);
}
//确认不小于初始大小、增长前的两倍大小
CurrentScale = FMath::Clamp(CurrentScale, 1.0f, 2.0f);
OurVisibleComponent->SetWorldScale3D(FVector(CurrentScale));
}
//基于MoveX和MoveY来处理移动
{
if (!CurrentVelocity.IsZero())
{
FVector NewLoaction = GetActorLocation() + (CurrentVelocity*DeltaTime);
SetActorLocation(NewLoaction);
}
}
}
// Called to bind functionality to input
void AMyPawn::SetupPlayerInputComponent(class UInputComponent* InputComponent)
{
Super::SetupPlayerInputComponent(InputComponent);
//响应Grow键
InputComponent->BindAction("Grow",IE_Pressed,this,&AMyPawn::StartGrowing);
InputComponent->BindAction("Grow",IE_Released,this,&AMyPawn::StopGrowing);
//响应两个坐标轴值
InputComponent->BindAxis("MoveX", this, &AMyPawn::Move_XAxis);
InputComponent->BindAxis("MoveY", this, &AMyPawn::Move_YAxis);
}
void AMyPawn::Move_XAxis(float AxisValue)
{
//前后
CurrentVelocity.X = FMath::Clamp(AxisValue, -1.0f, 1.0f)*100.0f;
}
void AMyPawn::Move_YAxis(float AxisValue)
{
//左右
CurrentVelocity.Y = FMath::Clamp(AxisValue, -1.0f, 1.0f)*100.0f;
}
//跳跃
void AMyPawn::StartGrowing()
{
bGrowing = true;
}
void AMyPawn::StopGrowing()
{
bGrowing = false;
}
0 0
- UE4学习笔记4th
- UE4学习笔记5th
- UE4学习笔记6th
- UE4学习笔记7th
- UE4学习笔记8th
- UE4学习笔记9th
- UE4学习笔记10th
- UE4学习笔记11th
- UE4学习笔记12th
- UE4学习笔记13th
- UE4学习笔记14th
- UE4学习笔记15th
- UE4学习笔记16th
- UE4学习笔记17th
- UE4学习笔记18th
- UE4学习笔记19th
- UE4学习笔记20th
- UE4学习笔记21th
- 精简点名IAP错误
- 暑假作业 三
- mysql和sqlite语法区别
- UNIX的标准与选项
- UE4学习笔记3rd
- UE4学习笔记4th
- 2014款新宝来车引擎盖开关在哪里
- [Leetcode 124, Hard] Binary Tree Maximum Path Sum
- 爷爷去世了。
- 天才的大三暑假修行之旅.表面着色器(二)
- [Leetcode 44, Hard] Wildcard match
- html,js ,jsp工作学习
- Web服务器Nginx+PHP&DB服务器mysql主从(新手)-1.DB服务器配置
- 暑假习题 四