218 .The Skyline Problem
来源:互联网 发布:mac怎么删除abc输入法 编辑:程序博客网 时间:2024/05/18 17:39
A city's skyline is the outer contour of the silhouette formed by all the buildings in that city when viewed from a distance. Now suppose you are given the locations and height of all the buildings as shown on a cityscape photo (Figure A), write a program to output the skyline formed by these buildings collectively (Figure B).
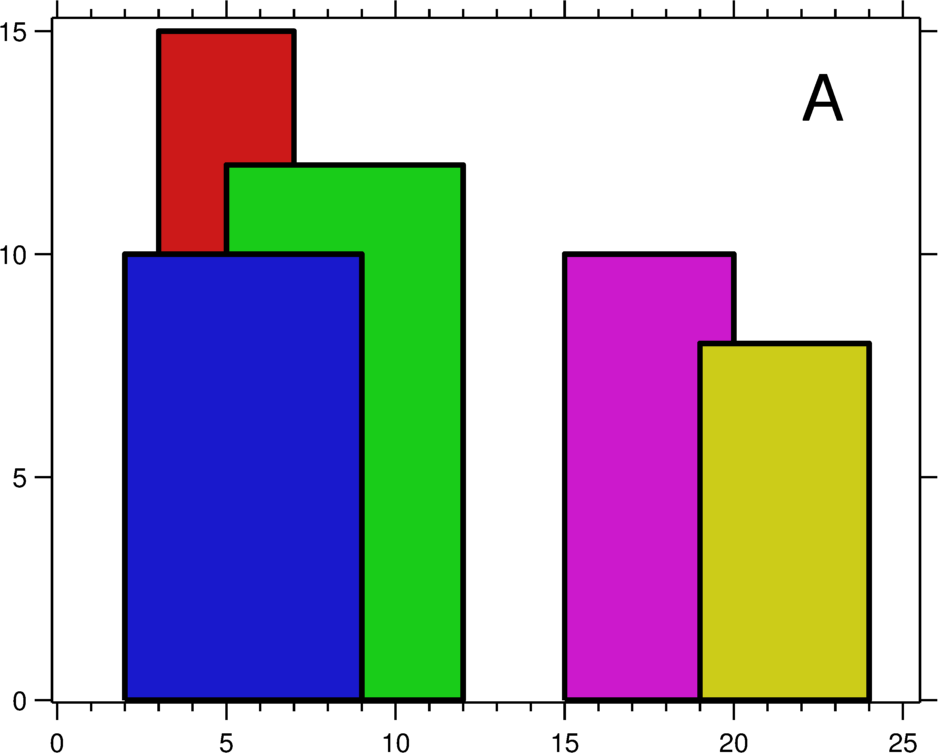
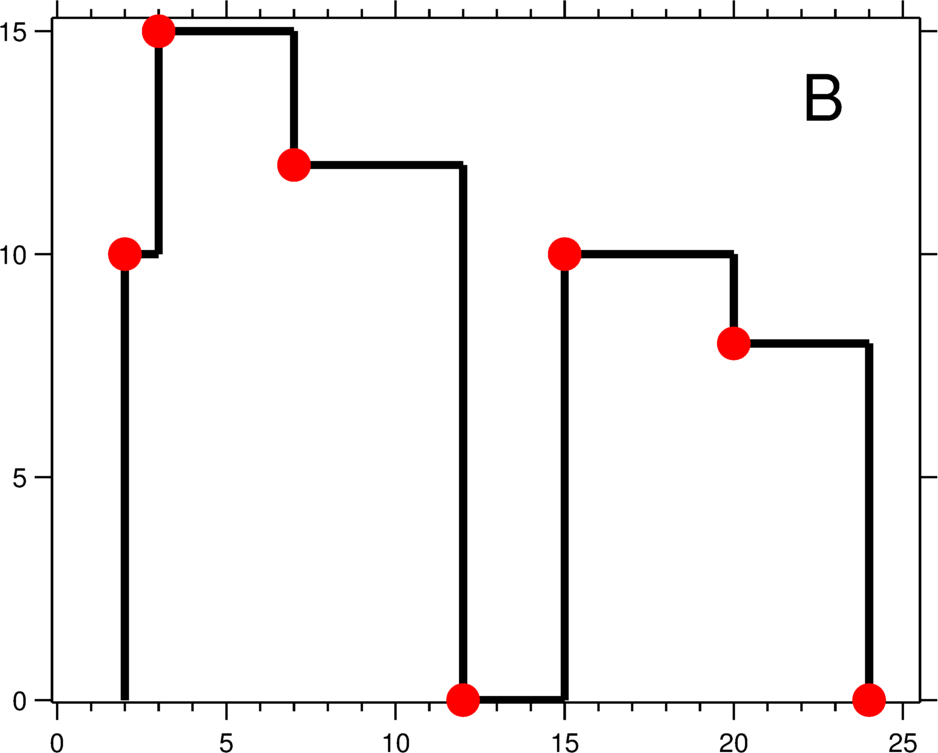
The geometric information of each building is represented by a triplet of integers [Li, Ri, Hi]
, where Li
and Ri
are the x coordinates of the left and right edge of the ith building, respectively, and Hi
is its height. It is guaranteed that 0 ≤ Li, Ri ≤ INT_MAX
, 0 < Hi ≤ INT_MAX
, and Ri - Li > 0
. You may assume all buildings are perfect rectangles grounded on an absolutely flat surface at height 0.
For instance, the dimensions of all buildings in Figure A are recorded as: [ [2 9 10], [3 7 15], [5 12 12], [15 20 10], [19 24 8] ]
.
The output is a list of "key points" (red dots in Figure B) in the format of [ [x1,y1], [x2, y2], [x3, y3], ... ]
that uniquely defines a skyline. A key point is the left endpoint of a horizontal line segment. Note that the last key point, where the rightmost building ends, is merely used to mark the termination of the skyline, and always has zero height. Also, the ground in between any two adjacent buildings should be considered part of the skyline contour.
For instance, the skyline in Figure B should be represented as:[ [2 10], [3 15], [7 12], [12 0], [15 10], [20 8], [24, 0] ]
.
Notes:
- The number of buildings in any input list is guaranteed to be in the range
[0, 10000]
. - The input list is already sorted in ascending order by the left x position
Li
. - The output list must be sorted by the x position.
- There must be no consecutive horizontal lines of equal height in the output skyline. For instance,
[...[2 3], [4 5], [7 5], [11 5], [12 7]...]
is not acceptable; the three lines of height 5 should be merged into one in the final output as such:[...[2 3], [4 5], [12 7], ...]
Sweepline is used in solving the problem. List<int[]> height
is used to save each of the line segments including both start and end point. The trick here is to set the start segment as negative height. This has a few good uses:
first, make sure the start segment comes before the end one after sorting.
second, when pushing into the queue, it is very each to distinguish either to add or remove a segment.
lastly, when the two adjacent building share same start and end x value, the next start segment always come before due to the negative height, this makes sure that when we peek the queue, we always get the value we are supposed to get. When the first building is lower, when we peek the queue, we get the height of the second building, and the first building will be removed in the next round of iteration. When the second building is lower, the first peek returns the first building and since it equals to prev
, the height will not be added.
public List<int[]> getSkyline(int[][] buildings){List<int[]> res = new ArrayList<int[]>();PriorityQueue<Integer> maxHeap = new PriorityQueue<Integer>(11,new Comparator<Integer>(){public int compare(Integer a, Integer b){return b - a;}});List<int[]> bl = new ArrayList<int[]>();for (int i = 0; i < buildings.length; i++){bl.add(new int[] { buildings[i][0], buildings[i][2] });bl.add(new int[] { buildings[i][1], -buildings[i][2] });}Collections.sort(bl, new Comparator<int[]>(){public int compare(int[] a, int[] b){return a[0] == b[0] ? b[1] - a[1] : a[0] - b[0];}});int pre = 0, cur = 0;for (int i = 0; i < bl.size(); i++){int[] b = bl.get(i);if (b[1] > 0){maxHeap.add(b[1]);cur = maxHeap.peek();} else{maxHeap.remove(-b[1]);cur = (maxHeap.peek() == null) ? 0 : maxHeap.peek();}if (cur != pre){res.add(new int[] { b[0], cur });pre = cur;}}return res;}
-------------------------------------------------------------------------------------------
刚开始看错数据范围了。。。。。Integer.MAXVALUE o(n)的空间肯定爆的,规模小一点还可以上线段树
public List<int[]> getSkyline(int[][] buildings){int m = buildings.length;int maxn = 10002;SegmentTree3 segt = new SegmentTree3(maxn);ArrayList<int[]> arraylist = new ArrayList<>();HashMap<Integer, Integer> starthashmap = new HashMap<>(m);HashMap<Integer, Integer> endhashmap = new HashMap<>(m);for (int i = 0; i < m; i++){int start = buildings[i][0] + 1;int end = buildings[i][1] + 1;starthashmap.put(start, starthashmap.size());endhashmap.put(end, endhashmap.size());segt.update(start, end, buildings[i][2], 1, maxn, 1);}int ground = 0;int preheight = 0;for (int i = 0; i <= maxn; i++){int height = segt.query(i + 1, i + 1, 1, maxn, 1);if (height > 0 && preheight == 0)ground = height;else if (height < ground)ground = height;// System.out.println(i+":"+height+", ground:"+ground);if (height > preheight)arraylist.add(new int[] { i, height });else if (height < preheight){if (height <= ground|| (starthashmap.containsKey(i - 1) && endhashmap.containsKey(i - 1)))arraylist.add(new int[] { i - 1, height });else{arraylist.add(new int[] { i - 1, ground });arraylist.add(new int[] { i, height });}}preheight = height;}return arraylist;}
class SegmentTree3{private int[] ele;private int[] col;public SegmentTree3(int maxn){ele = new int[maxn << 2];col = new int[maxn << 2];}private void PushUP(int rt){ele[rt] = Math.max(ele[rt << 1], ele[rt << 1 | 1]);}private void PushDown(int rt){if (col[rt] != 0){col[rt << 1] = Math.max(col[rt << 1], col[rt]);col[rt << 1 | 1] = Math.max(col[rt << 1 | 1], col[rt]);ele[rt << 1] = Math.max(ele[rt << 1], col[rt]);ele[rt << 1 | 1] = Math.max(ele[rt << 1 | 1], col[rt]);col[rt] = 0;}}public void update(int L, int R, int c, int l, int r, int rt){if (L <= l && r <= R){col[rt] = Math.max(col[rt], c);ele[rt] = Math.max(ele[rt], c);return;}PushDown(rt);int m = (l + r) >> 1;if (L <= m)update(L, R, c, l, m, rt << 1);if (R > m)update(L, R, c, m + 1, r, rt << 1 | 1);PushUP(rt);}public int query(int L, int R, int l, int r, int rt){if (L <= l && r <= R)return ele[rt];PushDown(rt);int m = (l + r) >> 1;int ret = 0;if (L <= m)ret = Math.max(ret, query(L, R, l, m, rt << 1));if (R > m)ret = Math.max(ret, query(L, R, m + 1, r, rt << 1 | 1));return ret;}}
- 218 .The Skyline Problem
- leetcode 218: The Skyline Problem
- Leetcode 218 The Skyline Problem
- leetcode 218: The Skyline Problem
- Leetcode #218 The Skyline Problem
- [LeetCode 218] The Skyline Problem
- 105 - The Skyline Problem
- leetcode - The Skyline Problem
- The Skyline Problem
- The Skyline Problem
- The Skyline Problem
- LeetCode - The Skyline Problem
- leetcode:the skyline problem
- LeetCode218:The Skyline Problem
- The Skyline Problem -- leetcode
- Leetcode -- The Skyline Problem
- The Skyline Problem
- The Skyline Problem
- <JAVA学习笔记6>——程序死锁、中断
- nginx之keepalive请求长连接复用率统计
- C语言基础 swtich结构中变量是字符,case的形式
- 观察者模式下的搜索引擎结合浏览器的完美实现
- JZOJ2756. 【SDOI2012】Longge的问题
- 218 .The Skyline Problem
- Python 包管理工具解惑
- 23. Merge k Sorted Lists 合并K个有序链表
- MySQL的CAST函数用法
- C++设计模式浅识组合模式
- MYSQL-----数据库的操作及存储引擎的选择
- Sql Server之旅——第七站 为什么都说状态少的字段不能建索引
- 列表状态自动切换
- Problem C: 小数计算——结构体