How To Execute Shell Command From Java
来源:互联网 发布:携程幼儿园虐童 知乎 编辑:程序博客网 时间:2024/06/05 10:47
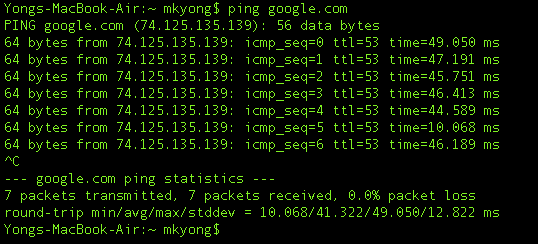
In Java, you can use Runtime.getRuntime().exec
to execute external shell command :
p = Runtime.getRuntime().exec("host -t a " + domain); p.waitFor(); BufferedReader reader = new BufferedReader(new InputStreamReader(p.getInputStream())); String line = ""; while ((line = reader.readLine())!= null) {sb.append(line + "\n"); }
1. PING example
Classical example to execute the ping
command and print out its output.
ExecuteShellComand.java
package com.mkyong.shell; import java.io.BufferedReader;import java.io.InputStreamReader; public class ExecuteShellComand { public static void main(String[] args) { ExecuteShellComand obj = new ExecuteShellComand(); String domainName = "google.com"; //in mac oxsString command = "ping -c 3 " + domainName; //in windows//String command = "ping -n 3 " + domainName; String output = obj.executeCommand(command); System.out.println(output); } private String executeCommand(String command) { StringBuffer output = new StringBuffer(); Process p;try {p = Runtime.getRuntime().exec(command);p.waitFor();BufferedReader reader = new BufferedReader(new InputStreamReader(p.getInputStream())); String line = "";while ((line = reader.readLine())!= null) {output.append(line + "\n");} } catch (Exception e) {e.printStackTrace();} return output.toString(); } }
Output
PING google.com (74.125.135.x): 56 data bytes64 bytes from 74.125.135.x: icmp_seq=0 ttl=53 time=8.289 ms64 bytes from 74.125.135.x: icmp_seq=1 ttl=53 time=7.733 ms64 bytes from 74.125.135.x: icmp_seq=2 ttl=53 time=8.343 ms --- google.com ping statistics ---3 packets transmitted, 3 packets received, 0.0% packet lossround-trip min/avg/max/stddev = 7.733/8.122/8.343/0.276 msnull
2. HOST Example
Example to execute shell command
host -t a google.com
to get all the IP addresses that attached to google.com. Later, we use regular expression to grab all the IP addresses and display it.P.S “host” command is available in *nix system only.
ExecuteShellComand.javapackage com.mkyong.shell; import java.io.BufferedReader;import java.io.InputStreamReader;import java.util.ArrayList;import java.util.List;import java.util.regex.Matcher;import java.util.regex.Pattern; public class ExecuteShellComand { private static final String IPADDRESS_PATTERN = "([01]?\\d\\d?|2[0-4]\\d|25[0-5])" + "\\.([01]?\\d\\d?|2[0-4]\\d|25[0-5])" + "\\.([01]?\\d\\d?|2[0-4]\\d|25[0-5])" + "\\.([01]?\\d\\d?|2[0-4]\\d|25[0-5])"; private static Pattern pattern = Pattern.compile(IPADDRESS_PATTERN);private static Matcher matcher; public static void main(String[] args) { ExecuteShellComand obj = new ExecuteShellComand(); String domainName = "google.com";String command = "host -t a " + domainName; String output = obj.executeCommand(command); //System.out.println(output); List<String> list = obj.getIpAddress(output); if (list.size() > 0) {System.out.printf("%s has address : %n", domainName);for (String ip : list) {System.out.println(ip);}} else {System.out.printf("%s has NO address. %n", domainName);} } private String executeCommand(String command) { StringBuffer output = new StringBuffer(); Process p;try {p = Runtime.getRuntime().exec(command);p.waitFor();BufferedReader reader = new BufferedReader(new InputStreamReader(p.getInputStream())); String line = "";while ((line = reader.readLine())!= null) {output.append(line + "\n");} } catch (Exception e) {e.printStackTrace();} return output.toString(); } public List<String> getIpAddress(String msg) { List<String> ipList = new ArrayList<String>(); if (msg == null || msg.equals(""))return ipList; matcher = pattern.matcher(msg);while (matcher.find()) {ipList.add(matcher.group(0));} return ipList;}}Output
转自:http://www.mkyong.com/java/how-to-execute-shell-command-from-java/google.com has address : 74.125.135.x74.125.135.x74.125.135.x74.125.135.x74.125.135.x74.125.135.x
0 0
- How To Execute Shell Command From Java
- How to execute shell script in Java?
- How to execute system command in MSSQL
- How to execute system command in MSSQL
- How to execute system command in MSSQL
- How to execute system command in MSSQL
- How to Execute a Command in C# ?
- How to Execute a Command in C# ?
- How to create and execute JAR file in Java – Command line Eclipse Netbeans
- How to execute the command with root jurisdiction in pyCharm
- How to execute Makefile
- How to run FTE and FTE from command line
- How to Recover from a Truncate Command [ID 117055.1]
- How to open Firefox and chrome from command line
- How to use FTP from the command line.
- How to access Dropbox from the command line in Linux
- How to identify video formats from command line on Linux
- Ubuntu Tip:How to show desktop from command line
- iOS 5 Storyboard 入门-1
- Hive 内建操作符与函数开发
- 并查集
- XML CDATA
- Unity3D接入移动MMSDK支付的问题(弱联网篇)
- How To Execute Shell Command From Java
- SharePoint Security and Permission System Overview
- 块""可能与您正在运行的Windows版本不兼容。检查该模块是否与regsvr32.exe的x86或x64版
- GitHub超详细图文攻略 - Git客户端下载安装 GitHub提交修改源码工作流程 Git分支 标签 过滤 Git版本工作流
- top命令
- FTP是什么
- Hive JDBC
- 是世间的活动和施工队
- 1.排序(插入排序)